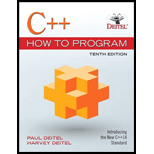
C++ How to Program (10th Edition)
10th Edition
ISBN: 9780134448237
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 19, Problem 19.21E
Program Plan Intro
Program Plan:
This Program defines the following structure to represent a List Node in Linked List
structlist _node {
intdata ;
structlist _node *next; // pointer to next node in the list
}node;
To allow searching an element in a list following method is defined.
node * searchNode (node *head, int data);
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Topic: Singly Linked ListImplement the following functions in C++ program. Read the question carefully. Below is the "CODE THAT NEEDS TO BE IMPROVED" or "NOT FINAL CODE" (See attached photo for reference)
bool addAt(int num, int pos)
This method will add the integer num to the posth position of the list and returns true if the value of pos is valid.
Performing addAt(20, 2) in the example list will add 20 at the 2nd position and the linked list will now look like this: 10 -> 20 -> 30 -> 40 -> 50.
When the value of pos is invalid i.e. greater than the size + 1 or less than one, return false.
bool addAt(int num, int pos) {
if (pos == 1) { // case 1: addHead
addHead(num);
return true;
}
if (pos == index + 1) { // case 3: addTail
addTail(num);
return true;
}
node* currnode = head; //addAt(20, 3)
int count = 0;
while (currnode…
C++ PROGRAMMING
(Linked list) complete the functions:
You have to continue on implementing your Array List namely the following functions:
Example ArrayList: [10, 30, 40, 50]
void addAt(int num, int pos)
This method will add the integer num to the posth position of the list.
Performing addAt(20, 2) in the example list will add 20 at the 2nd position and the array will now look like this: [10, 20, 30, 40, 50]
When the value of pos is greater than the size + 1 or less than one, output "Position value invalid"
void removeAt(int pos)
Removes the number in the posth position of the list.
Performing removeAt(3) in the example list will remove the 3rd element of the list and the updated array will be: [10, 30, 50]
When the value of pos is greater than the size or less than one, output "Position value invalid"
My incomplete code:
#include <cstdlib>#include <iostream>using namespace std;
class ArrayList : public List { // : means "is-a" / extend int* array; int index;…
[ ]
[]
power
In the cell below, you are to write a function "power(list)" that takes in a list as its input, and then returns the power set of that list. You may assume that the input will not have any duplicates (i.e., it's a set).
After compiling the above cell, you should be able to compile the following cell and obtain the desired outputs.
print (power ( ["A", "B"]),
power ( ["A", "B", "C"]))
+ Code + Markdown
This should return
[[], ["A"], ["B"], ["A", "B"]] [[], ["A"], ["B"], ["C"], ["A", "B"], ["A", "C"], ["B", "C"], ["A", "B", "C"]] (the order in which the 'sets' appear does not matter, just that they are all there)
Python
Python
Chapter 19 Solutions
C++ How to Program (10th Edition)
Ch. 19 - Prob. 19.6ECh. 19 - Prob. 19.7ECh. 19 - Prob. 19.8ECh. 19 - Prob. 19.9ECh. 19 - Prob. 19.10ECh. 19 - Prob. 19.11ECh. 19 - (Infix-to-Post fix conversion) Stacks are used by...Ch. 19 - Prob. 19.13ECh. 19 - Prob. 19.14ECh. 19 - Prob. 19.15E
Ch. 19 - Prob. 19.16ECh. 19 - Prob. 19.17ECh. 19 - (Duplicate Elimination) In this chapter, we saw...Ch. 19 - Prob. 19.19ECh. 19 - Prob. 19.20ECh. 19 - Prob. 19.21ECh. 19 - Prob. 19.22ECh. 19 - Prob. 19.23ECh. 19 - Prob. 19.24ECh. 19 - Prob. 19.25ECh. 19 - Prob. 19.26ECh. 19 - Prob. 19.27ECh. 19 - Prob. 19.28E
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Create a c++ shopping cart program using: linked list- - this will be used as the customer's cart. This is where the items that the customer will buy are placed before checking them out. Array - to store your store products. Pointers - since you will be using linked lists, it is imperative that you also will be using pointers. Functions - Make sure that your program will have user-defined functions (e.g.: function for adding nodes, function for displaying the cart, etc.) The menu options would be coutarrow_forward.cpp file pleasearrow_forwardPsecode code explaination of the problem C++arrow_forward
- (Q5) This is a Data Structures problem and the programming language used is Lisp. Solve the question we detailed steps and make it concise and easy to understand. Please and thank you.arrow_forward(Q6) This is a Data Structures problem and the programming language used is Lisp. Solve the question we detailed steps and make it concise and easy to understand. Please and thank you. Can you please explain this question specifically what you are doing in each step because it is a very confusing topic. Thanks!arrow_forwardDon't use vector array .using ifstream and ofstream. C++ programarrow_forward
- (Circular linked lists) This chapter defined and identified various operations on a circular linked list.a. Write the definitions of the class circularLinkedList and its member functions. (You may assume that the elements of the circular linked list are in ascending order.)b. Write a program to test various operations of the class defined in (a).arrow_forwardC++arrow_forward(Don't use C++) Only use Carrow_forward
- Please help with the follow question in C++arrow_forward1. Write and test a function to meet this specification. (You only need to write the function; your instructor will test it). squareEach(nums) nums is a list of numbers. Modifies the list by squaring each entry. Should return the list of squared entries. (You only need to write the function; your instructor will test it). 2. Write and test a function to meet this specification. sumList(nums) nums is a list of numbers. Returns the sum of the numbers in a list. (You only need to write the function; your instructor will test it). 3. Write and test a function to meet this specification. toNumbers(strList) strList is a list of strings, each of which represents a number. Modifies each entry in the list by converting it into a number. It should return the list of numbers. (You only need to write the function; your instructor will test it).arrow_forward[In c#] Write a class with name Arrays . This class has an array which should be initialized by user.Write a method Sum that should sum even numbers in array and return sum. write a function with name numFind in this class with working logic as to find the mid number of an array. After finding this number calculate its factorial.Write function that should display sum and factorial.Don’t use divide operatorarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
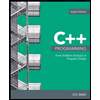
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning