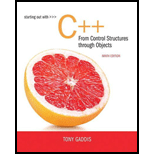
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 18, Problem 23RQE
Program Plan Intro
Standard Template Library (STL) list container:
The STL includes the collection of data types and algorithms which can be used by the programmer in their programs.
The STL “list” container is a template version of “doubly” linked list. The elements of the container can iterate either forward or backward. It can grow at either front or back of the list.
The header file named “#include<list>” is used to implement the list container in a
Syntax:
The syntax for “STL list” container is as follows:
list <data_type> variable_name;
- “list” is a keyword which is used to create a list.
- “<data_type>” is the type for each element in the list.
- “variable_name” is the name of the list which is defined by the user.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
emacs/lisp function
Write a function in Lisp that takes one parameter which we can assume to be a list. The function should print all the elements of the list using the function princ and a space between them. Use the function mapc for this purpose.Show a few examples of testing this function. Show one example using the function apply and one using the function funcall.
A "generic" data structure cannot use a primitive type as its generic type.
O True
False
Should write in c language not c++ or not c#
Chapter 18 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 18.1 - Prob. 18.1CPCh. 18.1 - Prob. 18.2CPCh. 18.1 - Prob. 18.3CPCh. 18.1 - Prob. 18.4CPCh. 18.2 - Prob. 18.5CPCh. 18.2 - Prob. 18.6CPCh. 18.2 - Prob. 18.7CPCh. 18.2 - Prob. 18.8CPCh. 18.2 - Prob. 18.9CPCh. 18.2 - Prob. 18.10CP
Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - Prob. 3RQECh. 18 - Prob. 4RQECh. 18 - Prob. 5RQECh. 18 - Prob. 6RQECh. 18 - Prob. 7RQECh. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - Prob. 17RQECh. 18 - Prob. 18RQECh. 18 - Prob. 19RQECh. 18 - Prob. 20RQECh. 18 - Prob. 21RQECh. 18 - Prob. 22RQECh. 18 - Prob. 23RQECh. 18 - Prob. 24RQECh. 18 - Prob. 25RQECh. 18 - T F The programmer must know in advance how many...Ch. 18 - T F It is not necessary for each node in a linked...Ch. 18 - Prob. 28RQECh. 18 - Prob. 29RQECh. 18 - Prob. 30RQECh. 18 - Prob. 31RQECh. 18 - Prob. 32RQECh. 18 - Prob. 33RQECh. 18 - Prob. 34RQECh. 18 - Prob. 35RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Prob. 6PCCh. 18 - Prob. 7PCCh. 18 - List Template Create a list class template based...Ch. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Prob. 12PCCh. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In c++ Please show me the Test output *You may not use any STL containers in your implementations Need help with the following functions: -LinkedList(const LinkedList<T>& other_list) Constructs a container with a copy of each of the elements in another, in the same order. -LinkedList& operator=(const LinkedList& other_list) Replaces the contents of this list with a copy of each element in another, in the same order. -void resize(std::size_t n) resizes the list so that it contains n elements. -void resize(std::size_t n, const T &fill_values) resizes the list so that it contains n elements -void remove(const T &val) Removes from the container all the elements that compare equal to val - bool operator == (const LinkedList &another) Compares this list with another for equality - bool operator != (const LinkedList &another) Compares this list with another for equality CODE #include <iostream> template <typename T> class LinkedList { struct…arrow_forwardASSUMING C LANGUAGE True or False: You can have the data portion of a Linked List be a Struct containing a Linked List itselfarrow_forwardPlease find this in quickly time pleasearrow_forward
- struct insert_at_back_of_dll { // Function takes a constant Book as a parameter, inserts that book at the // back of a doubly linked list, and returns nothing. void operator()(const Book& book) { / // TO-DO (2) |||| // Write the lines of code to insert "book" at the back of "my_dll". // // // END-TO-DO (2) ||| } std::list& my_dll; };arrow_forwardin c++ In a linked list made of integer data type, write only an application file that prints thoseintegers that are divisible by 5. Note that you assume that linked list exists, you onlyprints the value of data items that meet the condition (divisible by 5)arrow_forwardThe definition of linked list is given as follows: struct Node { ElementType Element ; struct Node *Next ; } ; typedef struct Node *PtrToNode, *List, *Position; If L is head pointer of a linked list, then the data type of L should be ??arrow_forward
- c++ data structures, linked list. write a function that rearrange the linked list of integers such as the odd items comes first don''t use different data structures.arrow_forward#include <bits/stdc++.h> using namespace std; // Structure of a Node struct Node { int data;s struct Node *next; struct Node *prev; }; // Function to insert at the end void insertEnd(struct Node** start, int value) { // If the list is empty, create a single node // circular and doubly list if (*start == NULL) { struct Node* new_node = new Node; new_node->data = value; new_node->next = new_node->prev = new_node; *start = new_node; return; } // If list is not empty /* Find last node */ Node *last = (*start)->prev; // Create Node dynamically struct Node* new_node = new Node; new_node->data = value; // Start is going to be next of new_node new_node->next = *start; // Make new node previous of start (*start)->prev = new_node; // Make last preivous of new node new_node->prev = last; // Make new node next of old last last->next = new_node; } // Function to insert Node at the beginning // of the List, void insertBegin(struct…arrow_forwardwrite the following program using simply linked list in C++ language Add definition of the following functions into a simply linked list: 1) Insert before tail : Insert a value into a simply linked list, such that it's location will be before tail. So if a list contains {1, 2, 3}, insert before tail value 9 is called, the list will become {1, 2, 9, 3}. 2) Insert before value : Insert a value into a simply linked list, such that it's location will be before a particular value. So if a list contains {1, 2, 3}, insert before 2 value 9 is called, the list will become {1, 9, 2, 3}. 3)Count common elements : Count common values between two simply linked lists. So if a list1 contains {1, 2, 3, 4, 5}, and list2 contains {1, 3, 4, 6}, number of common elements is 3. 4) Check if sorted : Check if elements of simply linked lists are sorted in ascending order or not. So if a list contains {1, 3, 7, 8, 9} its sorted, but if a list contains {1, 3, 7, 2, 5} its not sorted. 5) Find…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
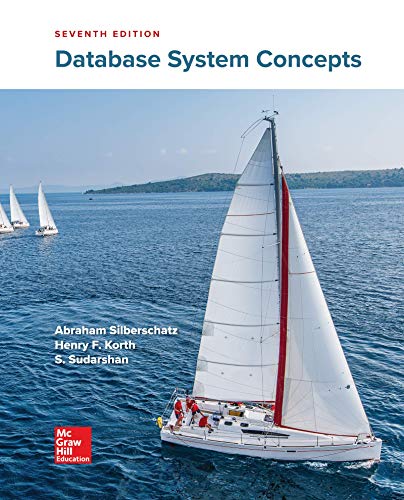
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
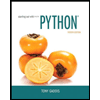
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
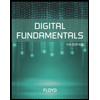
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
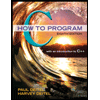
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
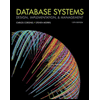
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
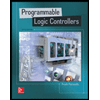
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education