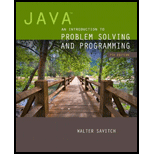
Write the method rotateRight that takes an array of integers and rotates the contents of the array to the right by two slots. Numbers that fall off the right should cycle back to the left. For example, if the input array a {1, 3, 5, 7} then the rotated array should be {5, 7,1, 3}. If the input array a {1, 2, 3} then the rotated array should be {2, 3,1}.

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Additional Engineering Textbook Solutions
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Starting Out with Python (3rd Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Programming in C
Starting Out with Java: Early Objects (6th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
- Write the following methodarrow_forwardIn Pythonarrow_forwardA void method creates an integer array of size 12. The method then ask user to input these 12 numbers and store them in the array. Then print out all the numbers that are multiples of 3 and then print out all the numbers that are multiples of 4. Example: Input a number (1/12): 3 Input a number (2/12): 5 Input a number (3/12): 7 Input a number (4/12): 8 Input a number (5/12): 2 Input a number (6/12): 3 Input a number (7/12): 10 Input a number (8/12): 6 Input a number (9/12): 2 Input a number (10/12): 7 Input a number (11/12): 12 Input a number (12/12): 9 The multiples of 3 are: 3, 3, 6, 12, 9 The multiples of 4 are: 8, 12arrow_forward
- Can you write code according to the desired conditions ?arrow_forwardWrite a program that declares an array of 15 integers and reads the values from the user. Make sure that the user doesn’t enter a value greater than 10 (if the user enters a value not in the allowed range, discard that value and read another to put into the array). Now tell the number that occur maximum number of times in the array. For example for the following 7 has maximum occurrence. 4,7,3,3,2,7,1,5,7,9,9,9,7,8,10 Use both linear and binary search.arrow_forwardWrite a method that takes two arrays as parameters. It should return a new array where each position has the sum of the two corresponding positions in the two incoming arrays. The returned array should be as long as the longer of the two passed in arrays.arrow_forward
- Write a method to read numbers from the keyboard into an array. Stop reading when the user enters the sentinel value -999. Return the number of values that were read. Do not put the sentinel in the array and do not include it in the number of values entered. Print an error message and stop reading if the user tries to enter more values than the array can hold.Write a method to print out the numbers in the array. Make sure the method only prints the array positions that were filled with the user's input. Print one number on each line.Write a method called zeros to return the number of entries in the array which contain zero.Write a method called replace which includes the parameters before and after, which are both integers. The method will change every array entry that contains the value before to contain the value after. For example, if the array contains {10,20,30,40,20,50}, before is 20 and after is 1, the array should be changed to {10,1,30,40,1,50}.Write a method called positive…arrow_forwardProblem: Write a method that will determine whether a given value can be made given an array of coin values. For example, each value in the array represents a coin with that value. An array with [1, 2, 3] represents 3 coins with the values, 1, 2, and 3. Determine whether or not these values can be used to make a desired value. The coins in the array are infinite and can only be used as many times as needed. Return true if the value can be made and false otherwise. Dynamic Programming would be handy for this problem. Data: An array containing 0 or more coin values and an amount. Output: Return true or false. Sample Data ( 1, 2, 3, 12, 5 ), 3 ( 4, 15, 16, 17, 1 ), 21 ( 1 ), 5 ( 3 ), 7 Sample Output true true true falsearrow_forwardJavaarrow_forward
- Write a program that prompts the user for an integer, then asks user to enter that many integer value. Store these values in an integer array and print the array. Reverse the array elements so that first element become the last element, the second element become the second to last element, and so on, with the old last element now first. Do not just reverse the order in which they are printed; actually, change the way they are stored in the array. Try not to create a second array; just rearrange the elements within the array you have. (Hints; Swap elements that need to change place.) When the elements have been reversed, Print the array again.arrow_forwardWrite a program that produces ten random permutations of the numbers 1 to 10. To generate a random permutation, you need to fill an array with the numbers 1 to 10 so that no two entries of the array have the same contents. You could do it by brute force, generating random values until you have a value that is not yet in the array. But that is inefficient. Instead, follow this algorithm: 1. Make a second array and fill it with the numbers 1 to 10. 2. Repeat 10 times A. Pick a random element from the second array. B. Remove it and append it to the permutation array. C. Display the permutation array (A helper method my be needed)arrow_forwardJAVA PROGRAM Write a program that asks the user to input two integers a and b. Then create an array that has all integer from a to b. Print out the array. (Don’t forget to consider the situation of a=b, a<b, and a>b.) Example 1: Input two numbers: 6 2 Array is [6, 5, 4, 3, 2] Example 2: Input two numbers: 4 8 Array is [4, 5, 6, 7, 8] Pass the above array as a parameter to a method called average and return the average and print it out in the main method. (Do not use Math library to get the average.)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
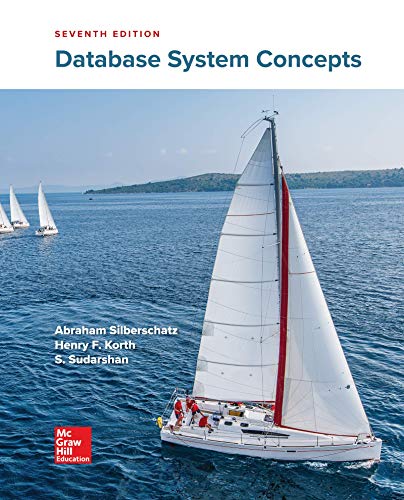
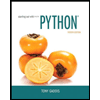
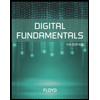
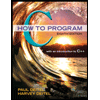
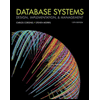
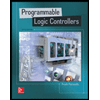