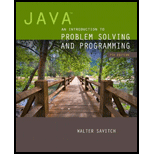
Explanation of Solution
Error in method definition “copyArray”:
- • The given method definition “copyArray” does not return an each element from the given argument array “anArray”.
- ○ Relatively, it returns a reference to the array it is given.
- • To produce a duplicate array, user would modify the statement “temp = anArray” to following statement.
for(int index = 0; index < anArray.length; index++)
temp[i] = anArray[i];
- ○ The above statement is used to returns the each element in the array “temp” using “for” loop.
Modified method definition of “copyArray”:
public static int[] copyArray(int[] anArray)
{
//Declare an array "temp"
int[] temp = new int[anArray.length];
/* Copy values of "anArray" to "temp" array using "for" loop */
for(int index = 0; index < anArray.length; index++)
//Store value of "anArray" to "temp" array
temp[index] = anArray[index];
//Display statement
System.out.println("Values in 'temp' array");
//Display the values in "temp" array
for(int index = 0; index < anArray.length; index++)
System.out.println(temp[index]);
//Return the values in "temp" array
return temp;
}
Explanation:
The above method definition is used to copy the array to another array variable.
- • Declare an array “temp”.
- • Copy the value of “anArray” to “temp” array using “for” loop.
- • Display the values in values of “temp” array using “for” loop.
Complete executable code:
The complete executable code for “copyArray” is given below:
//Import required package
import java.util.Scanner;
//Define "Main" class
class Main
{
//Define main function
public static void main(String[] args)
{
//Create an array "arr"
int[] arr = new int[5];
//Create scanner object
Scanner input = new Scanner(System...

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
- using r language Obtain a bootstrap t confidence interval estimate for the correlation statistic in Example 8.2 (law data in bootstrap).arrow_forwardusing r language Compute a jackknife estimate of the bias and the standard error of the correlation statistic in Example 8.2.arrow_forwardusing r languagearrow_forward
- using r languagearrow_forwardThe assignment here is to write an app using a database named CIT321 with a collection named students; we will provide a CSV file of the data. You need to use Vue.js to display 2 pages. You should know that this assignment is similar, all too similar in fact, to the cars4sale2 example in the lecture notes for Vue.js 2. You should study that program first. If you figure out cars4sale2, then program 6 will be extremely straightforward. It is not my intent do drop a ton of new material here in the last few days of class. The database contains 51 documents. The first rows of the CSV file look like this: sid last_name 1 Astaire first_name Humphrey CIT major hrs_attempted gpa_points 10 34 2 Bacall Katharine EET 40 128 3 Bergman Bette EET 42 97 4 Bogart Cary CIT 11 33 5 Brando James WEB 59 183 6 Cagney Marlon CIT 13 40 GPA is calculated as gpa_points divided by hrs_attempted. GPA points would have been arrived at by adding 4 points for each credit hour of A, 3 points for each credit hour of…arrow_forwardI need help to solve the following case, thank youarrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
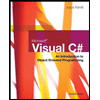
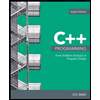
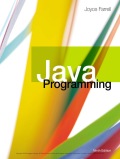
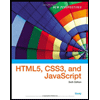
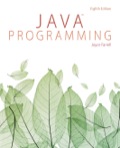