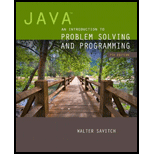
Java: An Introduction to Problem Solving and Programming (7th Edition)
7th Edition
ISBN: 9780133766264
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 7, Problem 19E
Write a sequential search of an array of integers, assuming that the array is sorted into ascending order. (Hint: Consider an array that contains the four integers 2, 4, 6, and 8. How can you tell that 5 is not in the array without comparing 5 to every integer in the array?)
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write the SQL code that permits to implement the tables: Student and Transcript. NB: Add the constraints on the attributes – keys and other.
Draw an ERD that will involve the entity types: Professor, Student, Department and Course. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Draw an ERD that represents a book in a library system. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Chapter 7 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Ch. 7.1 - What output will be produced by the following...Ch. 7.1 - What output will be produced by the following...Ch. 7.1 - What output will be produced by the following...Ch. 7.1 - Consider the following array: int [] a = new...Ch. 7.1 - What is wrong with the following code to...Ch. 7.1 - Write a complete Java program that reads 20 values...Ch. 7.2 - Write some Java code that will declare an array...Ch. 7.2 - Rewrite the method displayResults of the program...Ch. 7.2 - What output will be produced by the following...Ch. 7.2 - Give the definition of a static method called...
Ch. 7.2 - Give the definition of a static method called...Ch. 7.2 - Prob. 12STQCh. 7.2 - The following method compiles and executes but...Ch. 7.2 - Suppose that we add the following method to the...Ch. 7.3 - Prob. 15STQCh. 7.3 - Replace the last loop in Listing 7.8 with a loop...Ch. 7.3 - Suppose a is an array of values of type double....Ch. 7.3 - Suppose a is an array of values of type double...Ch. 7.3 - Prob. 19STQCh. 7.3 - Consider the partially filled array a from...Ch. 7.3 - Repeat the previous question, but this time assume...Ch. 7.3 - Write an accessor method getEntryArray for the...Ch. 7.4 - Prob. 23STQCh. 7.4 - Write the invocation of the method selectionSort...Ch. 7.4 - How would you need to change the method...Ch. 7.4 - How would you need to change the method...Ch. 7.4 - Consider an array b of int values in which a value...Ch. 7.5 - What output is produced by the following code?...Ch. 7.5 - Revise the method showTable in Listing 7.13 so...Ch. 7.5 - Write code that will fill the following array a...Ch. 7.5 - Write a void method called display such that the...Ch. 7.6 - Prob. 33STQCh. 7 - Write a program in a class NumberAboveAverage that...Ch. 7 - Write a program in a class CountFamiles that...Ch. 7 - Write a program in a class CountPoor that counts...Ch. 7 - Write a program in a class FlowerCounter that...Ch. 7 - Write a program in a class characterFrequency that...Ch. 7 - Create a class Ledger that will record the sales...Ch. 7 - Define the following methods for the class Ledger,...Ch. 7 - Write a static method isStrictlyIncreasing (double...Ch. 7 - Write a static method removeDuplicates(Character[]...Ch. 7 - Write a static method remove {int v, int [] in}...Ch. 7 - Suppose that we are selling boxes of candy for a...Ch. 7 - Create a class polynomial that is used to evaluate...Ch. 7 - Write a method beyond LastEntry (position) for the...Ch. 7 - Revise the class OneWayNoRepeatsList, as given in...Ch. 7 - Write a static method for selection sort that will...Ch. 7 - Overload the method selectionSort in Listing 7.10...Ch. 7 - Revise the method selectionSort that appears in...Ch. 7 - Prob. 18ECh. 7 - Write a sequential search of an array of integers,...Ch. 7 - Write a static method findFigure (picture,...Ch. 7 - Write a static method blur (double [] [] picture)...Ch. 7 - Write a program that reads integers, one per line,...Ch. 7 - The following code creates a small phone book. An...Ch. 7 - Write the method rotateRight that takes an array...Ch. 7 - The following code creates a ragged 2D array. The...Ch. 7 - Write a program that will read a line of text that...Ch. 7 - Prob. 2PPCh. 7 - Add a method bubbleSort to the class ArraySorter,...Ch. 7 - Add a method insertionSort to the class...Ch. 7 - The class TimeBook in Listing 7.14 is not really...Ch. 7 - Define a class called TicTacToe. An object of type...Ch. 7 - Repeat Programming Project 10 from Chapter 5 but...Ch. 7 - Prob. 8PPCh. 7 - Write a GUI application that displays a picture of...Ch. 7 - ELIZA was a program written in 1966 that parodied...Ch. 7 - Prob. 11PPCh. 7 - Create a GUI application that draws the following...Ch. 7 - Practice Program 2 used two arrays to implement a...Ch. 7 - Practice Program 5.4 asked you to define Trivia...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Write Java statements to accomplish each of the following tasks: Use one statement to decrement the variable X ...
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
In Exercises 1 through 22, determine the output displayed in the text box or list box by the lines of code. Dim...
Introduction To Programming Using Visual Basic (11th Edition)
A liter is 0.264179 gallons. Write a program that will read in the number of liters of gasoline consumed by the...
Problem Solving with C++ (10th Edition)
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
Functions make it easier for programmers to work in teams.
Starting Out with Python (4th Edition)
13. Consider the following strange, but true, units:
1 batman = 3 kilograms [kg] 1 hogshead = 63 gallons [gal]
...
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 2:21 m Ο 21% AlmaNet WE ARE HIRING Experienced Freshers Salesforce Platform Developer APPLY NOW SEND YOUR CV: Email: hr.almanet@gmail.com Contact: +91 6264643660 Visit: www.almanet.in Locations: India, USA, UK, Vietnam (Remote & Hybrid Options Available)arrow_forwardProvide a detailed explanation of the architecture on the diagramarrow_forwardhello please explain the architecture in the diagram below. thanks youarrow_forward
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
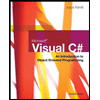
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
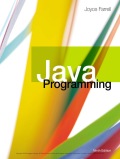
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
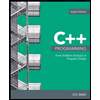
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
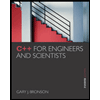
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
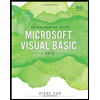
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Definition of Array; Author: Neso Academy;https://www.youtube.com/watch?v=55l-aZ7_F24;License: Standard Youtube License