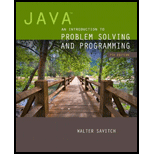
Write a static method blur (double [] [] picture) that you could use on a part of a picture file to obscure a detail such as a person's face or a license plate number. This method computes the weighted averages of the values in picture and returns them in a new two-dimensional array. To find a weighted average of a group of numbers, you count some of them more than others. Thus, you multiply each item by its weight, add these products together, and divide the result by the sum of the weights.
For each element in picture, compute the weighted average of the element and its immediate neighbors. Store the result in a new two-dimensional array in the same position that the element occupies in picture. This new array is the one the method returns.
The neighbors of an element in picture can be above, below, to the left of, and to the right of it, either vertically, horizontally, or diagonally. So each weighted average in the new array will be a combination of up to nine values from the array picture. A corner value will use only four values: itself and three neighbors. An edge value will use only six values: itself and five neighbors. But an interior value will use nine values: itself and eight neighbors. So you will need to treat the corners and edges separately from the other cells.
The weights to use are:
The element itself has the highest weight of 4, the horizontal and vertical neighbors have a weight of 2, and the diagonal neighbors have a weight of 1.
For example, suppose the values in picture are
and the new array is called result. In assigning weights, we will arbitrarily start with an element and consider neighbors in a clockwise direction. Thus, the interior value in result [1] [1] is equal to
To arrive at this equation, we started with the element at picture [1] [1] and then, beginning with the neighbor to the right, we considered neighbors in a clockwise direction. The corner value in result [0] [0] is equal to
Note that picture [0] [0] has fewer neighbors than an interior value such as picture [1] [1]. The same is true for an edge value such as picture [0] [1]. Thus, the edge value in result [0] [1] is equal to
The final array, result, would be

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Additional Engineering Textbook Solutions
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Starting Out With Visual Basic (8th Edition)
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
- Please original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forwardPlease original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forward
- What are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forwardDiscuss with appropriate examples the types of relationships in a database. Give one reference with your answer.arrow_forwardDetermine the velocity error constant (k,) for the system shown. + R(s)- K G(s) where: K=1.6 A(s+B) G(s) = as²+bs C(s) where: A 14, B =3, a =6. and b =10arrow_forward
- • Solve the problem (pls refer to the inserted image)arrow_forwardWrite .php file that saves car booking and displays feedback. There are 2 buttons, which are <Book it> <Select a date>. <Select a date> button gets an input from the user, start date and an end date. Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, it books cars for specific dates, with bookings saved. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. In the booking.json file, if the Car ID and start date and end date matches, it fails Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert).arrow_forwardWrite .php file with the html that saves car booking and displays feedback. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. There are 2 buttons, which are <Book it> <Select a date> Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, book cars for specific dates, with bookings saved. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert). And then add an additional feature that only free dates are selectable (e.g., calendar view).arrow_forward
- • Solve the problem (pls refer to the inserted image) and create line graph.arrow_forwardwho started the world wide webarrow_forwardQuestion No 1: (Topic: Systems for collaboration and social business The information systems function in business) How does Porter's competitive forces model help companies develop competitive strategies using information systems? • List and describe four competitive strategies enabled by information systems that firms can pursue. • Describe how information systems can support each of these competitive strategies and give examples.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
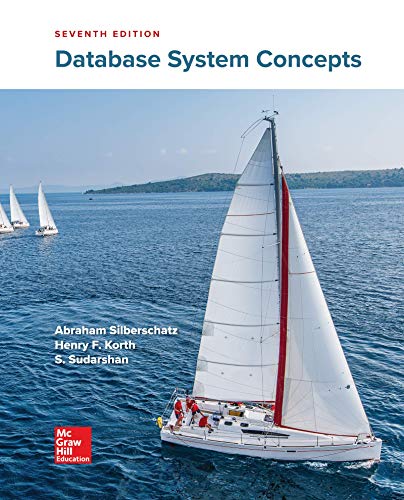
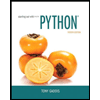
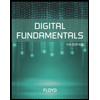
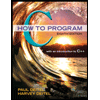
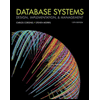
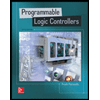