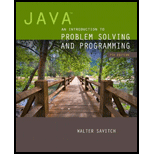
Java: An Introduction to Problem Solving and Programming (7th Edition)
7th Edition
ISBN: 9780133766264
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 7.2, Problem 10STQ
Give the definition of a static method called showArray that has an array of base type char as a single parameter and that writes one line of text to the screen consisting of the characters in the array argument written in order.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Of the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization?
Part A - Define each primary component of the information system.
Part B - Include your perspective on why your selection is most important.
Part C - Provide an example from your personal experience to support your answer.
Management Information Systems
Q2/find the transfer function C/R for the system shown in the figure
Re
ད
Chapter 7 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Ch. 7.1 - What output will be produced by the following...Ch. 7.1 - What output will be produced by the following...Ch. 7.1 - What output will be produced by the following...Ch. 7.1 - Consider the following array: int [] a = new...Ch. 7.1 - What is wrong with the following code to...Ch. 7.1 - Write a complete Java program that reads 20 values...Ch. 7.2 - Write some Java code that will declare an array...Ch. 7.2 - Rewrite the method displayResults of the program...Ch. 7.2 - What output will be produced by the following...Ch. 7.2 - Give the definition of a static method called...
Ch. 7.2 - Give the definition of a static method called...Ch. 7.2 - Prob. 12STQCh. 7.2 - The following method compiles and executes but...Ch. 7.2 - Suppose that we add the following method to the...Ch. 7.3 - Prob. 15STQCh. 7.3 - Replace the last loop in Listing 7.8 with a loop...Ch. 7.3 - Suppose a is an array of values of type double....Ch. 7.3 - Suppose a is an array of values of type double...Ch. 7.3 - Prob. 19STQCh. 7.3 - Consider the partially filled array a from...Ch. 7.3 - Repeat the previous question, but this time assume...Ch. 7.3 - Write an accessor method getEntryArray for the...Ch. 7.4 - Prob. 23STQCh. 7.4 - Write the invocation of the method selectionSort...Ch. 7.4 - How would you need to change the method...Ch. 7.4 - How would you need to change the method...Ch. 7.4 - Consider an array b of int values in which a value...Ch. 7.5 - What output is produced by the following code?...Ch. 7.5 - Revise the method showTable in Listing 7.13 so...Ch. 7.5 - Write code that will fill the following array a...Ch. 7.5 - Write a void method called display such that the...Ch. 7.6 - Prob. 33STQCh. 7 - Write a program in a class NumberAboveAverage that...Ch. 7 - Write a program in a class CountFamiles that...Ch. 7 - Write a program in a class CountPoor that counts...Ch. 7 - Write a program in a class FlowerCounter that...Ch. 7 - Write a program in a class characterFrequency that...Ch. 7 - Create a class Ledger that will record the sales...Ch. 7 - Define the following methods for the class Ledger,...Ch. 7 - Write a static method isStrictlyIncreasing (double...Ch. 7 - Write a static method removeDuplicates(Character[]...Ch. 7 - Write a static method remove {int v, int [] in}...Ch. 7 - Suppose that we are selling boxes of candy for a...Ch. 7 - Create a class polynomial that is used to evaluate...Ch. 7 - Write a method beyond LastEntry (position) for the...Ch. 7 - Revise the class OneWayNoRepeatsList, as given in...Ch. 7 - Write a static method for selection sort that will...Ch. 7 - Overload the method selectionSort in Listing 7.10...Ch. 7 - Revise the method selectionSort that appears in...Ch. 7 - Prob. 18ECh. 7 - Write a sequential search of an array of integers,...Ch. 7 - Write a static method findFigure (picture,...Ch. 7 - Write a static method blur (double [] [] picture)...Ch. 7 - Write a program that reads integers, one per line,...Ch. 7 - The following code creates a small phone book. An...Ch. 7 - Write the method rotateRight that takes an array...Ch. 7 - The following code creates a ragged 2D array. The...Ch. 7 - Write a program that will read a line of text that...Ch. 7 - Prob. 2PPCh. 7 - Add a method bubbleSort to the class ArraySorter,...Ch. 7 - Add a method insertionSort to the class...Ch. 7 - The class TimeBook in Listing 7.14 is not really...Ch. 7 - Define a class called TicTacToe. An object of type...Ch. 7 - Repeat Programming Project 10 from Chapter 5 but...Ch. 7 - Prob. 8PPCh. 7 - Write a GUI application that displays a picture of...Ch. 7 - ELIZA was a program written in 1966 that parodied...Ch. 7 - Prob. 11PPCh. 7 - Create a GUI application that draws the following...Ch. 7 - Practice Program 2 used two arrays to implement a...Ch. 7 - Practice Program 5.4 asked you to define Trivia...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Speed of Sound The following table shows the approximate speed of sound in air, water, and steel. Create an app...
Starting Out With Visual Basic (8th Edition)
A program contains the following function definition: def cube(num): return num num num Write a statement tha...
Starting Out with Python (4th Edition)
An array can be used to store large integers one digit at a time. For example, the integer 1234 could be stored...
Problem Solving with C++ (10th Edition)
Explain the term foreign key, and give an example.
Database Concepts (8th Edition)
ICA 8-20
A eutectic alloy of two metals contains the specific percentage of each metal that gives the lowest po...
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
17–1C A high-speed aircraft is cruising in still air. How does the temperature of air at the nose of the aircra...
Thermodynamics: An Engineering Approach
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Please original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forwardPlease original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forward
- What are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forwardDiscuss with appropriate examples the types of relationships in a database. Give one reference with your answer.arrow_forwardDetermine the velocity error constant (k,) for the system shown. + R(s)- K G(s) where: K=1.6 A(s+B) G(s) = as²+bs C(s) where: A 14, B =3, a =6. and b =10arrow_forward
- • Solve the problem (pls refer to the inserted image)arrow_forwardWrite .php file that saves car booking and displays feedback. There are 2 buttons, which are <Book it> <Select a date>. <Select a date> button gets an input from the user, start date and an end date. Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, it books cars for specific dates, with bookings saved. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. In the booking.json file, if the Car ID and start date and end date matches, it fails Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert).arrow_forwardWrite .php file with the html that saves car booking and displays feedback. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. There are 2 buttons, which are <Book it> <Select a date> Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, book cars for specific dates, with bookings saved. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert). And then add an additional feature that only free dates are selectable (e.g., calendar view).arrow_forward
- • Solve the problem (pls refer to the inserted image) and create line graph.arrow_forwardwho started the world wide webarrow_forwardQuestion No 1: (Topic: Systems for collaboration and social business The information systems function in business) How does Porter's competitive forces model help companies develop competitive strategies using information systems? • List and describe four competitive strategies enabled by information systems that firms can pursue. • Describe how information systems can support each of these competitive strategies and give examples.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
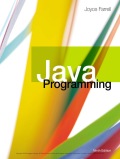
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Definition of Array; Author: Neso Academy;https://www.youtube.com/watch?v=55l-aZ7_F24;License: Standard Youtube License