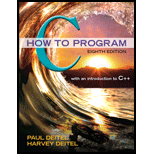
Concept explainers
(Complex Class) Create a class called Complex for performing arithmetic with complex numbers. Write a
{
}
where i is
Use double variables to represent the private data of the class. Provide a constructor that enables an object of this class to be initialized when it’s declared. The constructor should contain default values in case no initializers are provided. Provide public member functions that perform the following tasks:
- Adding two Complex numbers: The real parts are added together and the imaginary parts are added together.
- Subtracting two Complex numbers: The real part of the right operand is subtracted from the real part of the left operand, and the imaginary part of the right operand is subtracted from the imaginary part of the left operand.
- Printing Complex numbers in the form (a, b), where a is the real part and b is the imaginary part.

Want to see the full answer?
Check out a sample textbook solution
Chapter 17 Solutions
C How to Program (8th Edition)
Additional Engineering Textbook Solutions
Absolute Java (6th Edition)
Modern Database Management
Computer Science: An Overview (12th Edition)
Concepts Of Programming Languages
Starting Out with C++: Early Objects (9th Edition)
- (The Time class) Design a class named Time. The class contains: - The data fields hour, minute, and second that represent a time. - A no-arg constructor that creates a Time object for the current time. (The values of the data fields will represent the current time.) -A constructor that constructs a Time object with a specified elapsed time since midnight, January 1, 1970, in milliseconds. (The values of the data fields will represent this time.) -A constructor that constructs a Time object with the specified hour, minute, and second. - Three getter methods for the data fields hour, minute, and second, respectively. -A method named setTime (long elapseTime) that sets a new time for the object using the elapsed time. For example, if the elapsed time is 555550000 milliseconds, the hour is 10, the minute is 19, and the second is 10. Draw the UML diagram for the class and then implement the class. Write a test program that creates two Time objects (using new Time (), new Time(555550000),…arrow_forwardNonearrow_forwardUse C++arrow_forward
- 5 (The Time class) Design a class named Time. The class contains: ■ Data fields hour, minute, and second that represent a time. ■ A no-arg constructor that creates a Time object for the current time. ■ A constructor that constructs a Time object with a specified elapse time since the middle of night, Jan 1, 1970, in seconds. ■ A constructor that constructs a Time object with the specified hour, minute, and second. ■ Three get functions for the data fields hour, minute, and second. ■ A function named setTime(int elapseTime) that sets a new time for the object using the elapsed time. Draw the UML diagram for the class. Implement the class. Write a test program that creates two Time objects, one using a no-arg constructor and the other using Time (555550), and display their hour, minute, and second. (Hint: The first two constructors will extract hour, minute, and second from the elapse time. For example, if the elapse time is 555550 seconds, the hour is 10, the minute is 19, and the…arrow_forwardRewrite the calculator program using a class called calculator. Your program will keep asking the user if they want to perform more calculations or quit and will have a function displayMenu to display the different functions e.g .(1 - addition, 2- subtraction, 3- multiplication, 4- division) Your program must have appropriate constructors and member functions to initialize, access, and manipulate the data members as well as : A member function to perform the addition and return the result A member function to perform the subtraction and return the result A member function to perform the multiplication and return the result A member function to perform the division and return the resultarrow_forwardSUBJECT: OOPPROGRAMMING LANGUAGE: C++ ALSO ADD SCREENSHOTS OF OUTPUT. Write a class Distance to measure distance in meters and kilometers. The class should have appropriate constructors for initializing members to 0 as well as user provided values. The class should have display function to display the data members on screen. Write another class Time to measure time in hours and minutes. The class should have appropriate constructors for initializing members to 0 as well as user provided values. The class should have display function to display the data members on screen. Write another class which has appropriate functions for taking objects of the Distance class and Time class to store time and distance in a file. Make the data members and functions in your program const where applicablearrow_forward
- 2) Create a class called Complex for performing arithmetic with complex numbers. Write a program to test your class. Complex numbers have the form realPart + imaginaryPart * i Use double variables to represent the private data of the class. Provide a constructor that enables an object of this class to be initialized when it’s declared. The constructor should contain default values in case no initializers are provided. Provide public member functions that perform the following tasks: a) Adding two Complex numbers: The real parts are added together and the imaginary parts are added together. b) Subtracting two Complex numbers: The real part of the right operand is subtracted from the real part of the left operand, and the imaginary part of the right operand is subtracted from the imaginary part of the left operand. c) Printing Complex numbers in the form (a, b), where a is the real part and b is the imaginary part. Design: Code: Output:arrow_forwardPS: see image for question.arrow_forwardQ2) Write C++ code to create class called number. The private data members for this class are nobl (double), nob2 (double) and nob3(double). The member function for this class is: 1- (Constructor) that accepts nobl, nob2 and nob3 as arguments (inside the class). Write a main() that create two objects (h1) with initially data members (nob1 :13000, nob2:15 and nob3 :19) , (kl) with initially data members (nob1:16000, nob2:77 and nob3:2018) and (TI) with initially data members (nob1:15000, nob2:37 and nob3:2020). Finally print the information of the object that has smallest nob2 for these three objects using void display friend function:arrow_forward
- Instructions-Java Assignment is to define a class named Address. The Address class will have three private instance variables: an int named street_number a String named street_name and a String named state. Write three constructors for the Address class: an empty constructor (no input parameters) that initializes the three instance variables with default values of your choice, a constructor that takes the street values as input but defaults the state to "Arizona", and a constructor that takes all three pieces of information as input Next create a driver class named Main.java. Put public static void main here and test out your class by creating three instances of Address, one using each of the constructors. You can choose the particular address values that are used. I recommend you make them up and do not use actual addresses. Run your code to make sure it works. Next add the following public methods to the Address class and test them from main as you go: Write getters and…arrow_forwardPeople in School Objective: At the end of the activity, the students should be able to: Create a program that exhibits inheritance. Procedure: Write a simple information system that will store and display the complete information of a student, faculty, or employee. Create four (4) no-modifier classes named Person, Student, Faculty, and Employee. Create a public class named CollegeList. This class shall contain the main method. Refer to the UML Class Diagram for the names of the variables and methods. The (-) symbol represents private variables, while (+) represents public method. This should be the sequence of the program upon execution: Prompt the user to select among Employee, Faculty, or Student, by pressing E, F, or S. Ask the user to type the name and contact For Employee, ask the user to type the employee's monthly salary and the department where he/she belongs to (Ex. Registrar). Then, display name, contact number, salary, and For Faculty, ask the user to press Y if…arrow_forwardQ2) Write C++ code to create class called number. The private data members for this class are nobl (double), nob2 (double) and nob3(double). The member function for this class is: 1- (Constructor) that accepts nob1, nob2 and nob3 as arguments (inside the class). Write a main() that create two objects (hl) with initially data members (nobl :13000, nob2:15 and nob3 :19), (kl) with initially data members (nobl:16000, nob2:77 and nob3:2018) and (TI) with initially data members (nobl:15000, nob2:37 and nob3:2020). Finally print the information of the object that has smallest nob2 for these three objects using void display friend function:arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
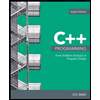