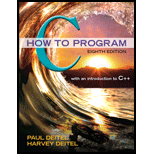
C How to Program (8th Edition)
8th Edition
ISBN: 9780133976892
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 17, Problem 17.13E
Program Plan Intro
Program Plan:
- Include the required header files.
- Create class Rectangle and Point.
- Provide a setFillCharacter function in order to draw the body of the rectangle.
- Provide a setPerimeterCharacter function in order to draw the border of the rectangle.
- Returns the rectangle inside a 25-by-25 box.
Program Description:
Program that creates a class Rectangle including a draw function which displays the rectangle inside a 25-by-25 box.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
(TicTacToe Class) Create a class TicTacToe that will enable you to write a complete programto play the game of tic-tac-toe. The class contains as private data a 3-by-3 two-dimensional arrayof integers. The constructor should initialize the empty board to all zeros. Allow two human players.Wherever the first player moves, place a 1 in the specified square. Place a 2 wherever the second player moves. Each move must be to an empty square. After each move, determine whether the gamehas been won or is a draw. If you feel ambitious, modify your program so that the computer makesthe moves for one of the players. Also, allow the player to specify whether he or she wants to go firstor second. If you feel exceptionally ambitious, develop a program that will play three-dimensionaltic-tac-toe on a 4-by-4-by-4 board. [Caution: This is an extremely challenging project that couldtake many weeks of effort!]
Q2) (Perfect Numbers) An integer number is said to be a perfect number if its factors,
including 1 (but not the number itself), sum to the number. For example, 6 is a perfect
number because 6 = 1 + 2 + 3. Write a function perfect that determines if parameter number
is a perfect number. Use this function in a program that determines and prints all the perfect
numbers between 1 and 1000. Print the factors of each perfect number to confirm that the
number is indeed perfect. Challenge the power of your computer by testing numbers much
larger than 1000.
(Geometry: MyRectangle2D class) Define the MyRectangle2D class that contains:
Two double data fields named x and y that specify the center of the rectangle with getter and setter methods. (Assume the rectangle sides are parallel to the x- or y-axis.)
The data fields width and height with getter and setter methods.
A no-arg constructor that creates a default rectangle with (0, 0) for (x, y) and 1 for both width and height.
A Constructor that creates a rectangle with the specified x, y, width, and height.
A method getArea() that returns the area of the rectangle.
A method getPerimeter() that returns the perimeter of the rectangle.
A method contains(double x, double y) that returns true if the specified point (x, y) is inside this rectangle (see Figure 10.24a ).
A method contains(MyRectangle2D r) that returns true if the specified rectangle is inside this rectangle (see Figure 10.24b ).
A method overlaps(MyRectangle2D r) that returns true if the specified rectangle overlaps with this…
Chapter 17 Solutions
C How to Program (8th Edition)
Ch. 17 - (Scope Resolution Operator) Whats the purpose of...Ch. 17 - (Enhancing Class Time) Provide a constructor thats...Ch. 17 - (Complex Class) Create a class called Complex for...Ch. 17 - (Rational Class) Create a class called Rational...Ch. 17 - Prob. 17.7ECh. 17 - Prob. 17.8ECh. 17 - Prob. 17.9ECh. 17 - Prob. 17.10ECh. 17 - (Rectangle Class) Create a class Rectangle with...Ch. 17 - (Enhancing Class Rectangle) Create a more...
Ch. 17 - Prob. 17.13ECh. 17 - (Hugelnteger Class) Create a class Hugelnteger...Ch. 17 - (TicTacToe Class) Create a class TicTacToe that...Ch. 17 - Prob. 17.16ECh. 17 - (Constructor Overloading) Can a Time class...Ch. 17 - Prob. 17.18ECh. 17 - Prob. 17.19ECh. 17 - (SavingsAccount Class) Create a SavingsAccount...Ch. 17 - Prob. 17.21ECh. 17 - (Time Class Modification) It would be perfectly...Ch. 17 - Prob. 17.23ECh. 17 - Prob. 17.24ECh. 17 - (Project: Card Shuffling and Dealing) Use the...Ch. 17 - Prob. 17.26ECh. 17 - (Project: Card Shuffling and Dealing) Modify the...Ch. 17 - (Project: Emergency Response Class) The North...
Knowledge Booster
Similar questions
- (Querying an Array of Invoice Objects) Use the class Invoice provided in the lab assignment to create an array of Invoice objects.Class Invoice includes four properties—a PartNumber (type int), a PartDescription (type string), a Quantity of the item being purchased (type int) and a Price (type decimal).Write a console application that performs the following queries on the array of Invoice objects and displays the results:a) Use LINQ to sort the Invoice objects by PartDescription.b) Use LINQ to sort the Invoice objects by Price.c) Use LINQ to select the PartDescription and Quantity and sort the results by Quantity.d) Use LINQ to select from each Invoice the PartDescription and the value of the Invoice(i.e., Quantity * Price). Name the calculated column InvoiceTotal. Order the results by Invoice value. [Hint: Use let to store the result of Quantity * Price in a new range variable total.]e) Using the results of the LINQ query in Part d, select the InvoiceTotals in the range$200 to…arrow_forward[In c#] Write a class with name Arrays . This class has an array which should be initialized by user.Write a method Sum that should sum even numbers in array and return sum. write a function with name numFind in this class with working logic as to find the mid number of an array. After finding this number calculate its factorial.Write function that should display sum and factorial.Don’t use divide operatorarrow_forwardQ3: (Tax Calculator) Develop a Java program that determines the total tax for each of four citizens. The tax rate is 10% for earnings up to 50,000 RM earned by each citizen and 15% for all earnings in excess of that ceiling. You are given a list with the citizens’ names and their earnings in a given year. Your program should input this information for each citizen, then determine and display the citizen’s total tax. Use class Scanner to input the data.arrow_forward
- (Python matplotlib or seaborn) CPU Usage We have the hourly average CPU usage for a worker's computer over the course of a week. Each row of data represents a day of the week starting with Monday. Each column of data is an hour in the day starting with 0 being midnight. Create a chart that shows the CPU usage over the week. You should be able to answer the following questions using the chart: When does the worker typically take lunch? Did the worker do work on the weekend? On which weekday did the worker start working on their computer at the latest hour? cpu_usage = [ [2, 2, 4, 2, 4, 1, 1, 4, 4, 12, 22, 23, 45, 9, 33, 56, 23, 40, 21, 6, 6, 2, 2, 3], # Monday [1, 2, 3, 2, 3, 2, 3, 2, 7, 22, 45, 44, 33, 9, 23, 19, 33, 56, 12, 2, 3, 1, 2, 2], # Tuesday [2, 3, 1, 2, 4, 4, 2, 2, 1, 2, 5, 31, 54, 7, 6, 34, 68, 34, 49, 6, 6, 2, 2, 3], # Wednesday [1, 2, 3, 2, 4, 1, 2, 4, 1, 17, 24, 18, 41, 3, 44, 42, 12, 36, 41, 2, 2, 4, 2, 4], # Thursday [4, 1, 2, 2, 3, 2, 5, 1, 2, 12, 33, 27, 43, 8,…arrow_forwardExercise 2. (Pascal's Triangle) Pascal's triangle Pn is a triangular array with n+1 rows, each listing the coefficients of the binomial expansion (x+ y)', where 0 _ "/workspace/project3 рython3 pавса1.ру 10 1 1 1 1 2 1 1 33 1 1 4 6 4 1 1 5 10 10 5 1 16 15 20 15 6 1 1 7 21 35 35 21 7 1 1 8 28 56 70 56 28 8 1 1 9 36 84 126 126 84 36 9 1 1 10 45 120 210 252 210 120 45 10 1 В равса1.ру import stdarray import stdio іпрort вys # Accept n (int) as command -line argument. ... # Setup a 2D ragged list a of integers. The list must have n + 1 rovs, vith the ith (0 <= i # <= n) row a[i] having i + 1 elements , each initialized to 1. For example, if n = 3, a should be # initialized to [[1], [1, 1], [1, 1, 1], [1, 1, 1, 1]]. a =... for i in range (...): ... # Fill the ragged list a using the formula for Pascal's triangle 1] [j - 1) + a[i - 1] [j] a [i][j] = a[i - 1] [j - 1] + a[i - 1] [j] #3 # vhere o <- i <- n and 1 <= j < i. for i in range (...): for j in range (...): ... # Write a to standard…arrow_forwardstate the statement either true or false.arrow_forward
- (Q1)This is a Data Structures problem and the programming language used is Lisp. Solve the question we detailed steps and make it concise and easy to understand. Please and thank you.arrow_forward3."""Code _Write a function validSolution/ValidateSolution/valid_solution()that accepts a 2D array representing a Sudoku board, and returns trueif it is a valid solution, or false otherwise. The cells of the sudokuboard may also contain 0's, which will represent empty cells.Boards containing one or more zeroes are considered to be invalid solutions.The board is always 9 cells by 9 cells, and every cell only contains integersfrom 0 to 9. (More info at: http://en.wikipedia.org/wiki/Sudoku)""" # Using dict/hash-tablefrom collections import defaultdict def valid_solution_hashtable(board): for i in range(len(board)): dict_row = defaultdict(int) dict_col = defaultdict(int) for j in range(len(board[0])): value_row = board[i][j] value_col = board[j][i] if not value_row or value_col == 0: return False if value_row in dict_row: return False else: dict_row[value_row] += 1.arrow_forwardTotaled columns. (Ending of sentence on attachment) please assist with Java code. Please attachment screenshot of successful output.arrow_forward
- """3. Write a function validSolution/ValidateSolution/valid_solution()that accepts a 2D array representing a Sudoku board, and returns trueif it is a valid solution, or false otherwise. The cells of the sudokuboard may also contain 0's, which will represent empty cells.Boards containing one or more zeroes are considered to be invalid solutions.The board is always 9 cells by 9 cells, and every cell only contains integersfrom 0 to 9. (More info at: http://en.wikipedia.org/wiki/Sudoku)""" # Using dict/hash-tablefrom collections import defaultdict def valid_solution_hashtable(board): for i in range(len(board)): dict_row = defaultdict(int) dict_col = defaultdict(int) for j in range(len(board[0])): value_row = board[i][j] value_col = board[j][i] if not value_row or value_col == 0: return False if value_row in dict_row: return False else: dict_row[value_row] += 1.arrow_forward7. (Array and $or) Find all documents where the categories is an array that contains either "boot" or "tent".arrow_forwardUSE PYTHON PROGRAMMING LANGUAGE(OOP) 1. (Geometry: n-sided regular polygon) An n-sided regular polygon’s sides all have the same length and all of its angles have the same degree (i.e., the polygon is both equilateral and equiangular). Design a class named RegularPolygon that contains: ■ A private int data field named n that defines the number of sides in the polygon. ■ A private float data field named the side that stores the length of the side. ■ A private float data field named x that defines the x-coordinate of the center of the polygon with a default value 0. ■ A private float data field named y that defines the y-coordinate of the center of the polygon with a default value 0. ■ A constructor that creates a regular polygon with the specified n (default 3), side (default 1), x (default 0), and y (default 0). ■ The accessor and mutator methods for all data fields. ■ The method getPerimeter() returns the perimeter of the polygon. ■ The method getArea() returns the area of the…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
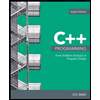
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning