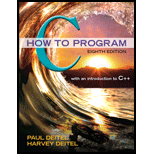
C How to Program (8th Edition)
8th Edition
ISBN: 9780133976892
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 17, Problem 17.23E
Program Plan Intro
Program plan:
- Define class Cards in header file CardH.h. Inside this class,
- Include a private data members face and suit of type int.
- Include a parametrized constructor to initialize face and suit to user defined values.
- A toString function in order to return card as string.
- Define class DeckOfCards in header file DeckOfCardsH.h. Inside this class,
- Include a private data membersdeck which is an array of Cards and currentCard of type int.
- Include a default constructor to initialize these data members.
- Include function shuffle, dealCard and more cards.
- Define a main function. Inside main function,
- Define object d of DeckOfCards class.
- Then apply function of DeckOfCards class to shuffle and deal cards.
Program Description: The following program will create a program for card shuffling and dealing with help of classes.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
12. Common
"The commonality between science and art is in trying to see profoundly - to develop
strategies of seeing and showing." -Edward Tufte
Write a Java program to find common elements between two given string arrays.
Program Description
Complete the main function that, given two arrays, arr1, arr2, with their sizes prints an
array containing the common elements in these arrays. DO NOT USE ANY JAVA BUILT-
IN ARRAY FUNCTIONS TO FIND COMMON ELEMENTS.
Constraints
• None
Input Format For Custom Testing
Sample Case 0
Sample Input For Custom Testing
5
1 1 1 1 1
3
1 2 3
Sample Output
['1']
Explanation
String value '1' is the only common element between the given two arrays.
Sample Case 1
Q2) (Perfect Numbers) An integer number is said to be a perfect number if its factors,
including 1 (but not the number itself), sum to the number. For example, 6 is a perfect
number because 6 = 1 + 2 + 3. Write a function perfect that determines if parameter number
is a perfect number. Use this function in a program that determines and prints all the perfect
numbers between 1 and 1000. Print the factors of each perfect number to confirm that the
number is indeed perfect. Challenge the power of your computer by testing numbers much
larger than 1000.
Describe how to pass an array as a parameter to a function.
Chapter 17 Solutions
C How to Program (8th Edition)
Ch. 17 - (Scope Resolution Operator) Whats the purpose of...Ch. 17 - (Enhancing Class Time) Provide a constructor thats...Ch. 17 - (Complex Class) Create a class called Complex for...Ch. 17 - (Rational Class) Create a class called Rational...Ch. 17 - Prob. 17.7ECh. 17 - Prob. 17.8ECh. 17 - Prob. 17.9ECh. 17 - Prob. 17.10ECh. 17 - (Rectangle Class) Create a class Rectangle with...Ch. 17 - (Enhancing Class Rectangle) Create a more...
Ch. 17 - Prob. 17.13ECh. 17 - (Hugelnteger Class) Create a class Hugelnteger...Ch. 17 - (TicTacToe Class) Create a class TicTacToe that...Ch. 17 - Prob. 17.16ECh. 17 - (Constructor Overloading) Can a Time class...Ch. 17 - Prob. 17.18ECh. 17 - Prob. 17.19ECh. 17 - (SavingsAccount Class) Create a SavingsAccount...Ch. 17 - Prob. 17.21ECh. 17 - (Time Class Modification) It would be perfectly...Ch. 17 - Prob. 17.23ECh. 17 - Prob. 17.24ECh. 17 - (Project: Card Shuffling and Dealing) Use the...Ch. 17 - Prob. 17.26ECh. 17 - (Project: Card Shuffling and Dealing) Modify the...Ch. 17 - (Project: Emergency Response Class) The North...
Knowledge Booster
Similar questions
- c++ onlyarrow_forwardC++ Coding: ArraysTrue and False Code function definitions for eoNum() and output(): Both eoNum() and output() are recursive functions. output() stores the even/odd value in an array. Store 0 if the element in the data array is even and store 1 if the element in the data array is odd. eoNum() displays all the values in an array to the console.arrow_forward"""3. Write a function validSolution/ValidateSolution/valid_solution()that accepts a 2D array representing a Sudoku board, and returns trueif it is a valid solution, or false otherwise. The cells of the sudokuboard may also contain 0's, which will represent empty cells.Boards containing one or more zeroes are considered to be invalid solutions.The board is always 9 cells by 9 cells, and every cell only contains integersfrom 0 to 9. (More info at: http://en.wikipedia.org/wiki/Sudoku)""" # Using dict/hash-tablefrom collections import defaultdict def valid_solution_hashtable(board): for i in range(len(board)): dict_row = defaultdict(int) dict_col = defaultdict(int) for j in range(len(board[0])): value_row = board[i][j] value_col = board[j][i] if not value_row or value_col == 0: return False if value_row in dict_row: return False else: dict_row[value_row] += 1.arrow_forward
- 4. (Prime Numbers) An integer is said to be prime if it is divisible by only 1 and itself. For example, 2, 3, 5 and 7 are prime, but 4, 6, 8 and 9 are not. Write a function called isPrime that receives an integer and determines whether the integer is prime or not. Write a test program that uses isPrime to determine and prints all the prime numbers between 1 and 1000. Display 10 numbers per line.arrow_forward(Replace strings) Write the following function that replaces the occurrence of a substring old_substring with a new substring new_substring in the string s. The function returns true if string s is changed, and otherwise, it returns false. bool replace_strings (string& s, const string& old_string, const string& new_string) Write a test program that prompts the user to enter three strings, i.e., s, old string, and new_string, and display the replaced string.arrow_forwardCODE USING C++ 1. Please Fix Me by CodeChum Admin Hi dear Programmer, I am broken. Can you please help me fix me? ?? Instructions: In the code editor, you are provided with a main() function that declares an array, sets its values, and then prints the sum of all of its values. However, there is a problem with how the array is declared. The array should be able to store 5 values. Your task is to fix the me array's declaration. Output 25arrow_forward
- pointers c structurearrow_forward(Tic-Tac-Toe) Write a program that allows two players to play the tic-tac-toe game. Your program must contain the class ticTacToe to implement a ticTacToe object. Include a 3-by-3 two-dimensional array, as a private member variable, to create the board. If needed, include additional member variables. Some of the operations on a ticTacToe object are printing the current board, getting a move, checking if a move is valid, and determining the winner after each move. Add additional operations as needed.arrow_forward(Python matplotlib or seaborn) CPU Usage We have the hourly average CPU usage for a worker's computer over the course of a week. Each row of data represents a day of the week starting with Monday. Each column of data is an hour in the day starting with 0 being midnight. Create a chart that shows the CPU usage over the week. You should be able to answer the following questions using the chart: When does the worker typically take lunch? Did the worker do work on the weekend? On which weekday did the worker start working on their computer at the latest hour? cpu_usage = [ [2, 2, 4, 2, 4, 1, 1, 4, 4, 12, 22, 23, 45, 9, 33, 56, 23, 40, 21, 6, 6, 2, 2, 3], # Monday [1, 2, 3, 2, 3, 2, 3, 2, 7, 22, 45, 44, 33, 9, 23, 19, 33, 56, 12, 2, 3, 1, 2, 2], # Tuesday [2, 3, 1, 2, 4, 4, 2, 2, 1, 2, 5, 31, 54, 7, 6, 34, 68, 34, 49, 6, 6, 2, 2, 3], # Wednesday [1, 2, 3, 2, 4, 1, 2, 4, 1, 17, 24, 18, 41, 3, 44, 42, 12, 36, 41, 2, 2, 4, 2, 4], # Thursday [4, 1, 2, 2, 3, 2, 5, 1, 2, 12, 33, 27, 43, 8,…arrow_forward
- Part 1 //Program Statment: Write a program(in c++) that first reads an integer for the array // size , then reads numbers into the array, and displays distinct numbers (i.e.// if a number appears multiple times, it is displayed only once). (Hint: Read // a number and store it to an array if it is new. If the number is already in // the array, discard it. After the input, the array contains the distinct numbers.) Part 2 //Program statment: When array is created, its size is fixed. Occasionally, you//need to add more values to an array, but the array is full. In this case , you //may create a new larger array to replace the existing array. Write a function //with the header;// // int * doubleCapacity(const int *list, int size)// //The function returns a new array that doubles the size of the parameter list.arrow_forwardC Programming Write function updateHorizontal to flip the discs of the opposing player, it should do the following a. Return type void b. Parameter list i. int rowii. int col iii. char board[ROW][COL] iv. Structure Player (i.e. player) as a pointer c. If the square to the left or right is a space, stop checking d. If the square to the left or right is the same character as the player’s character, stop checking e. If the square to the left or right is not the same character as the player’s character, flip the disc (i.e. X becomes O, O becomes X) Write function updateVertical to flip the discs of the opposing player, it should do the following a. Return type void b. Parameter list i. int rowii. int col iii. char board[ROW][COL] iv. Structure Player (i.e. player) as a pointer c. If the square above or below is a space, stop checking d. If the square above or below is the same character as the player’s character, stop checking e. If the square above or below is not the same character as…arrow_forward- array from user and print all the elements after doubuq have to separate function for doubling operation. 2arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
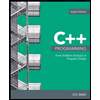
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning