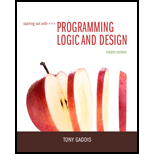
Concept explainers
Emp1oyee and ProductionWorker Classes
Design an Emp1oyeeclass that has fields for the following pieces of information:
- Employee name
- Employee number
Next, design a class named ProductionWorker that extends the Employee class. The ProductionWorker class should have fields to hold the following information:
- Shift number (an integer, such as 1, 2, or 3)
- Hourly pay rate
The workday is divided into two shifts: day and night. The shift field will hold an integer value representing the shift that the employee works. The day shift is shift 1 and the night shift is shift 2. Design the appropriate accessor and mutator methods for each class.
Once you have designed the classes, design a

Want to see the full answer?
Check out a sample textbook solution
Chapter 14 Solutions
Starting Out with Programming Logic and Design (4th Edition)
Additional Engineering Textbook Solutions
Starting Out with C++: Early Objects (9th Edition)
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Computer Science: An Overview (12th Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Starting Out with C++: Early Objects
- Problem: Employee and ProductionWorker Classes Write a python class named ProductionWorker that is a subclass of the Employee class. The ProductionWorker class should keep data attributes for the following information: • Shift number (an integer, such as 1, 2, or 3)• Hourly pay rateThe workday is divided into two shifts: day and night. The shift attribute will hold an integer value representing the shift that the employee works. The day shift is shift 1 and the night shift is shift 2. Write the appropriate accessor and mutator methods for this class. Once you have written the class, write a program that creates an object of the ProductionWorker class, and prompts the user to enter data for each of the object’s data attributes. Store the data in the object, then use the object’s accessor methods to retrieve it and display it on the screen Note: The program should be written in python. Sample Input/Output: Enter the name: Ahmed Al-AliEnter the ID number: 12345Enter the department:…arrow_forwardRetailItem Class Write a class named RetailItem that holds data about an item in a retail store. The class should have the following fields: description. The description field references a string object that holds a brief description of the item. • unitsonHand. The unitsOnHand field is an int variable that holds che number of units currently in inventory. price. The price field is a double that holds the item's retail price. Write a constructor that accepts arguments for each field, appropriate mutator methods that store values in these fields, and accessor methods that return the values in these fields. Once you have written the class, write a separate program that creates three RetaillItem objects and stores the following data in them: Description Units on Hand Price Item #1 Jacket 12 59.95 Item #2 Designer Jeans 40 34.95 Irem #3 Shirt 20 24.95arrow_forwardTrue or FalseA base class reference variable can reference an object of any class that is derived from the base class.arrow_forward
- Cruise Recreational Activities Example Recreational activities include things like aerobics, shuffle board, and swimming. Each activity is identified by an activity code and includes other information such as description. Classes are offered for each activity. A class is uniquely identified by a combination of the activity code and the day/time at which it is held. It is assumed that a specific class will never be offered for the same activity at the same day and time, although it could be offered on a different day and/or time. Other information about a class includes the enrollment limit and the current enrollment count. A class will never include more than one activity. A passenger can sign up for a class as long as there is sufficient room in the class. Passengers are identified by a unique passenger number. Other information stored about passengers includes name, address, and age. Passengers have no limit on the number and type of classes they can sign up for. When they…arrow_forwardCreate an Employee class that has properties for the following data: • Employee name • Employee number Next, create a class named ProductionWorker that is derived from the Employee class. The ProductionWorker class should have properties to hold the following data: • Shift number (an integer, such as 1, 2, or 3) • Hourly pay rate The workday is divided into two shifts: day and night. The Shift property will hold an integer value representing the shift that the employee works. The day shift is shift 1 and the night shift is shift 2. Create an application that creates an object of the ProductionWorker class and lets the user enter data for each of the object’s properties. Retrieve the object’s properties and display their values.arrow_forwardChild Class: Vegetable Write a child class called Vegetable. A vegetable is described by a name, the number of grams of sugar (as a whole number), the number of grams of sodium (as a whole number), and whether or not the vegetable is a starch. Core Class Components For the Vegetable class, write: the complete class header the instance data variables a constructor that sets the instance data variables based on parameters getters and setters; use instance data variables where appropriate a toString method that returns a text representation of a Vegetable object that includes all four characteristics of the vegetableJavaarrow_forward
- We use the _____ operator to create an instance of (object in) a particular class.arrow_forwardNewspaperSubscriber class- create an abstract class named NewspaperSubscriber with attributes to contain the subscriber’s street address and the subscription rate. Include get and set methods for both these attributes. The set method for the rate is abstract. The setAddress method must prompt the user to enter the subscriber’s address through the use of a dialog box. Create a constructor for the base class. Create a toString() method that concaternates and returns the subscriber’s street address and rate for display. Create 3 child classes named SevenDaySubscriber, Weekday Subscriber, and Weekend Subscriber. Each child class has an additional attribute called subType, which is a String, that will store the type of newspaper subscription. Create a setType method that will set the type of subscriber as follows: “Seven Day”, “Weekday”, or“Weekend”. Create a setRate method for each child class that sets the rate as follows: a SevenDaysubscriber pays R18.00 per week for his newspapers, a…arrow_forwardPet ClassDesign a class named Pet, which should have the following fields: name: The name field holds the name of a pet. type: The type field holds the type of animal that a pet is. Example values are "Dog", "Cat", and "Bird". age: The age field holds the pet’s age. The Pet class should also have the following methods: setName: The setName method stores a value in the name setType: The setType method stores a value in the type setAge: The setAge method stores a value in the age getName: The getName method returns the value of the name getType: The getType method returns the value of the type getAge: The getAge method returns the value of the age Once you have designed the class, design a program that creates an object of the class and prompts the user to enter the name, type, and age of his or her pet. This data should be stored in the object. Use the object’s accessor methods to retrieve the pet’s name, type, and age and display this data on the screen. *** I need help…arrow_forward
- Pet ClassDesign a class named Pet, which should have the following fields: name: The name field holds the name of a pet. type: The type field holds the type of animal that a pet is. Example values are "Dog", "Cat", and "Bird". age: The age field holds the pet’s age. The Pet class should also have the following methods: setName: The setName method stores a value in the name setType: The setType method stores a value in the type setAge: The setAge method stores a value in the age getName: The getName method returns the value of the name getType: The getType method returns the value of the type getAge: The getAge method returns the value of the age Once you have designed the class, design a program that creates an object of the class and prompts the user to enter the name, type, and age of his or her pet. This data should be stored in the object. Use the object’s accessor methods to retrieve the pet’s name, type, and age and display this data on the screen. The output Should be…arrow_forwardParent Class: Food Write a parent class called Food. A food is described by a name, the number of grams of sugar (as a whole number), and the number of grams of sodium (as a whole number). Core Class Components For the Food class, write: the complete class header the instance data variables a constructor that sets the instance data variables based on parameters getters and setters; use validity checking on the parameters where appropriate a toString method that returns a text representation of a Food object that includes all three characteristics of the food Class-Specific Method Write a method that calculates what percent of the daily recommended amount of sugar is contained in a food. The daily recommended amount might change, so the method takes in the daily allowance and then calculates the percentage. For example, let's say a food had 6 grams of sugar. If the daily allowance was 24 grams, the percent would be 0.25. For that same food, if the daily allowance was 36 grams, the…arrow_forwardT/F A method defined in a class can access the class' instance data without needing to pass them as parameters or declare them as local variables.arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
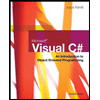
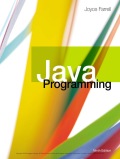