Write a Python program employee.py that computes the cumulative salary of employees based on the length of their contract and their annual merit rate.
Employee class
Write a Python program employee.py that computes the cumulative salary of employees based on the length of their contract and their annual merit rate.
Your program must include a class Employee with the following requirements:
Class attributes:
name: the name of the employee
position: the position of the employee
start_salary: the starting salary of the employee
annual_rate: the annual merit rate on the salary
contract_years: the number of years of salary
Class method:
get_cumulative_salary(): calculates and returns the cumulative salary of an employee based on the number of contract years. Round the cumulative salary to two digits after the decimal point.
Example: If start_salary = 100000, annual_rate = 5% and contract_years = 3:
Then the cumulative salary should be : 100000 + 105000 + 110250 = 315250
Outside of the class Employee, the program should have two functions:
Function name |
Function description |
Function input(s) |
Function output(s) / return value(s) |
---|---|---|---|
print_employees() |
Prints the employees' records in table format. The column spacing is 20, left-aligned. |
A list of employees objects |
The employees records |
main() |
Prompts for the number of employees. Prompts for the employee records comma-separated. Calls the print_employees() function. |
None |
- |
Starter code
class Employee:
# define constructor here
# todo
# instance method
def get_cumulative_salary(self):
"""
This function calculates the cumulative salary after
@param contract_years:
@return: cumulated_salary
"""
#todo
pass
def print_employees(employees):
"""
Prints employees records given a list of employee objects
@param employees:
@return:
"""
#todo
pass
def main():
"""
Prompts for the number of employees. Reads the employee records comma-separated. Calls the print_employees() function.
@return:
"""
number_of_employees = int(input("Enter the number of employees: "))
employees = []
# todo
print_employees(employees)
main()
Sample Output
Enter the number of employees: 4 name, position, start salary, annual rate, contract years: Anita Borg,Lead developer,250000,2.6,4 name, position, start salary, annual rate, contract years: Frances Allen,Computer Scientist,280400,3,10 name, position, start salary, annual rate, contract years: Carol Shaw,Video Game Designer,184600,5.2,6 name, position, start salary, annual rate, contract years: Radia Perlman,Network Engineer,312850,1.92,1 Name Position Start salary Annual rate Cumulative salary Anita Borg Lead developer 250000.0 2.6 1039680.39 Frances Allen Computer Scientist 280400.0 3.0 3214471.76 Carol Shaw Video Game Designer 184600.0 5.2 1261968.68 Radia Perlman Network Engineer 312850.0 1.92 312850
|

Step by step
Solved in 4 steps with 2 images

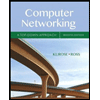
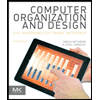
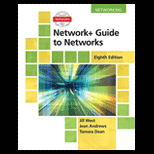
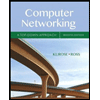
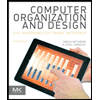
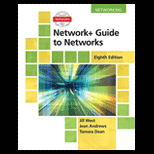
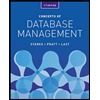
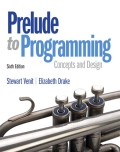
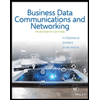