Pet Class Design a class named Pet, which should have the following fields: name: The name field holds the name of a pet. type: The type field holds the type of animal that a pet is. Example values are "Dog", "Cat", and "Bird". age: The age field holds the pet’s age. The Pet class should also have the following methods: setName: The setName method stores a value in the name setType: The setType method stores a value in the type setAge: The setAge method stores a value in the age getName: The getName method returns the value of the name getType: The getType method returns the value of the type getAge: The getAge method returns the value of the age Once you have designed the class, design a program that creates an object of the class and prompts the user to enter the name, type, and age of his or her pet. This data should be stored in the object. Use the object’s accessor methods to retrieve the pet’s name, type, and age and display this data on the screen. The output Should be similar to the picture below.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
- Pet Class
Design a class named Pet, which should have the following fields:- name: The name field holds the name of a pet.
- type: The type field holds the type of animal that a pet is. Example values are "Dog", "Cat", and "Bird".
- age: The age field holds the pet’s age.
- The Pet class should also have the following methods:
- setName: The setName method stores a value in the name
- setType: The setType method stores a value in the type
- setAge: The setAge method stores a value in the age
- getName: The getName method returns the value of the name
- getType: The getType method returns the value of the type
- getAge: The getAge method returns the value of the age
- Once you have designed the class, design a program that creates an object of the class and prompts the user to enter the name, type, and age of his or her pet. This data should be stored in the object. Use the object’s accessor methods to retrieve the pet’s name, type, and age and display this data on the screen. The output Should be similar to the picture below.
Sample Pseudocode:
// main module
Module main()
// Declare input variables
Declare String inputName, String inputType, Integer inputAge
// class variable to hold a pet
Declare Pet Animal
// create a Pet object
Set Animal = new Pet()
// Get values for a pet
Display “Enter a pet name:”
Input inputName
Animal.setName(inputName)
Display “Enter a pet type:”
Input inputType
Animal.setType(inputType)
Display “Enter a pet age:”
Input inputAge
Animal.setAge(inputAge)
// Show values for this pet
Display “The pet name is “,Animal.getName()
Display “The pet type is “,Animal.getType()
Display “The pet age is “,Animal.getAge()
End Module
Class Pet
// Fields
Private String name
Private String type
Private Integer age
// Constructor
Public Module Pet(String n, String t, Integer a)
Set name = n
Set type = t
Set age = a
End Module
// Mutators
Public Module setName(String n)
Set name = n
End Module
Public Module setType(String t)
Set type = t
End Module
Public Module setAge(Integer a)
Set age = a
End Module
// Accessors
Public Function String getName()
Return name
End Function
Public Function String getType()
Return type
End Function
Public Function getAge()
Return age
End Function

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

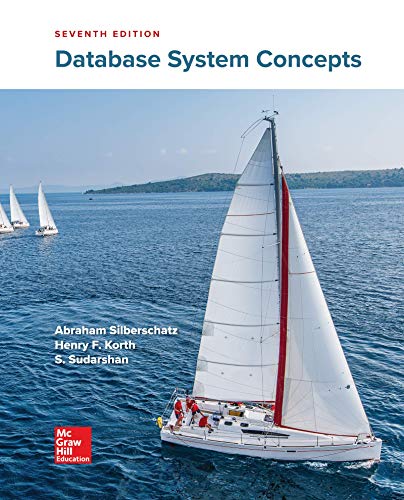
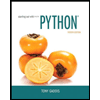
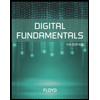
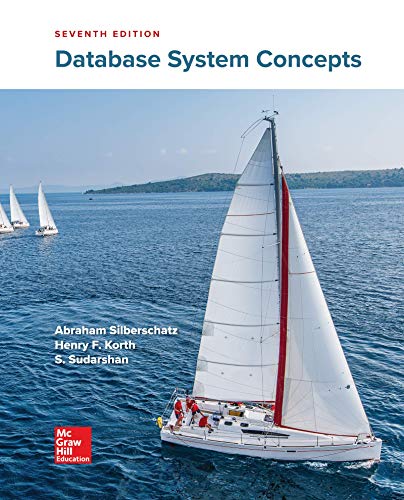
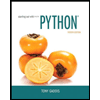
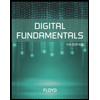
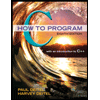
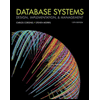
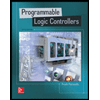