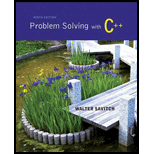
Problem Solving with C++ (9th Edition)
9th Edition
ISBN: 9780133591743
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 10.2, Problem 24STE
Program Plan Intro
Constructor initialization section
Program plan:
- In the “DayOfYear” class, declare the default and parameterized constructor in the “public” access specifier.
- Define the default constructor of class “DayOfYear”.
- Assign the value “1” to the variable “month” in the constructor initialization section.
- Assign the value “1” to the variable “day” in the constructor initialization section.
- Define the parameterized constructor of class “DayOfYear”.
- Assign parameter values to the variables “month” and “day” in the constructor initialization section.
- Call the “checkDate()” function to check the date is valid.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Describe three (3) Multiplexing techniques common for fiber optic links
Could you help me to know features of the following concepts:
- commercial CA
- memory integrity
- WMI filter
Briefly describe the issues involved in using ATM technology in Local Area Networks
Chapter 10 Solutions
Problem Solving with C++ (9th Edition)
Ch. 10.1 - Given the following structure and structure...Ch. 10.1 - Consider the following type definition: struct...Ch. 10.1 - What is the error in the following structure...Ch. 10.1 - Given the following struct definition: struct A {...Ch. 10.1 - Here is an initialization of a structure type....Ch. 10.1 - Write a definition for a structure type for...Ch. 10.1 - Prob. 7STECh. 10.1 - Prob. 8STECh. 10.1 - Give the structure definition for a type named...Ch. 10.1 - Declare a variable of type StockRecord (given in...
Ch. 10.2 - Below we have redefined the class DayOfYear from...Ch. 10.2 - Given the following class definition, write an...Ch. 10.2 - Prob. 13STECh. 10.2 - The private member function DayOfYear::checkDate...Ch. 10.2 - Suppose your program contains the following class...Ch. 10.2 - Suppose you change Self-Test Exercise 15 so that...Ch. 10.2 - Explain what public: and private: do in a class...Ch. 10.2 - a. How many public: sections are required in a...Ch. 10.2 - Give a definition for the function with the...Ch. 10.2 - Give a definition for the function with the...Ch. 10.2 - Give a definition for the function with the...Ch. 10.2 - Suppose your program contains the following class...Ch. 10.2 - How would you change the definition of the class...Ch. 10.2 - Prob. 24STECh. 10.3 - When you define an ADT as a C++ class, should you...Ch. 10.3 - When you define an ADT as a C++ class, what items...Ch. 10.3 - Suppose your friend defines an ADT as a C++ class...Ch. 10.3 - Redo the three- and two-parameter constructors in...Ch. 10.4 - How does inheritance support code reuse and make...Ch. 10.4 - Can a derived class directly access by name a...Ch. 10.4 - Suppose the class SportsCar is a derived class of...Ch. 10 - Solution to Practice Program 10.1 Redefine...Ch. 10 - Redo your definition of the class CDAccount from...Ch. 10 - Define a class for a type called CounterType. An...Ch. 10 - Write a grading program for a class with the...Ch. 10 - Redo Programming Project 1 (or do it for the first...Ch. 10 - Define a class called Month that is an abstract...Ch. 10 - Redefine the implementation of the class Month...Ch. 10 - My mother always took a little red counter to the...Ch. 10 - Write a rational number class. This problem will...Ch. 10 - Define a class called Odometer that will be used...Ch. 10 - Redo Programming Project 7 from Chapter 5 (or do...Ch. 10 - The U.S. Postal Service printed a bar code on...Ch. 10 - Consider a class Movie that contains information...
Knowledge Booster
Similar questions
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
- a) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forwardusing r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
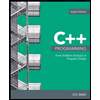
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
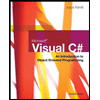
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
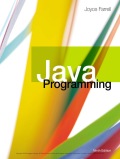
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
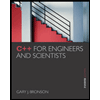
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
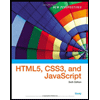
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning