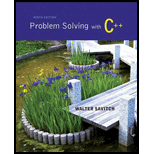
Given the following structure and structure variable declaration:
struct TermAccount { double balance; double interestRate; int term; char initial1; char initial2; }; TermAccount account; |
what is the type of each of the following? Mark any that are not correct, a. account.balance
a. account.balance
b. account.interestRate
c. TermAccount.term
d. savingsAccount.initial1
e. account.initial2
f. account

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Problem Solving with C++ (9th Edition)
Additional Engineering Textbook Solutions
Starting Out with C++: Early Objects (9th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
C How to Program (8th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Starting Out with Java: Early Objects (6th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
- Define a struct type that represents an exercise plan. The struct should store the following data: plan name (a character array of size 50) and goal calories to burn (an integer). The type should be renamed from struct exercise_plan to Exercise_plan.arrow_forwardLook at the following statement.enum Color { RED, ORANGE, GREEN, BLUE };A) What is the name of the data type declared by this statement?B) What are the enumerators for this type?C) Write a statement that defines a variable of this type and initializes it with a validvalue.arrow_forwardstruct namerec{ char last[15]; char first[15]; char middle[15]; }; struct payrecord{ int id; struct namerec name; float hours, rate; float regular, overtime; float gross, tax_withheld, net; }; Using C language. Given the above declaration, let payroll data record be stored in a structure called payrecord. Also define a type called payrecord for the structure data type that houses a payroll data record: typedef struct payrecord payrecord; This program reads data, computes payroll and prints it. Each data record is a structure, and the payroll is an array of structures. Overtime hours are 150% of the rate. (Note: Maximum regular hours for the week is 40.) Tax is withheld 15% if weekly pay is below 500, 28% if pay is below 1000, and 33% otherwise. A summary report prints the total gross pay and tax withheld. The following are the function prototypes: void readName(payrecord payroll[], int i); - reads a single name. void printName(payrecord payroll[], int i); - prints a single name. void…arrow_forward
- Q No 3. #include <string> using namespace std; class Account { public: Account(string accountName, int initialBalance) { name=accountName; if (initialBalance > 0) { balance = initialBalance; } } void deposit(int depositAmount) { if (depositAmount > 0) { balance = balance + depositAmount; } } int getBalance() const { return balance; } void setName(string accountName) { name = accountName; } string getName() const { return name; } private: string name; int balance; }; Rewrite the following code in correct form. a) Assume the following prototype of destructor is declared in class Time: void ~Time(int); b) Assume the following prototype of constructor is declared in class Employee: int Employee(string, string);…arrow_forwardIn C++ struct applianceType { string supplier; string modelNo; double cost; }; applianceType myNewAppliance; applianceType applianceList[25]; Write the C++ statements that correctly initialize the cost of each appliance in applianceList to 0.arrow_forwardstruct gameType { string title; int numPlayers; bool online; }; gameType Awesome[50]; Write the C++ statements that print out all gameType fields from Awesome whose numPlayers is > 1.arrow_forward
- struct namerec{ char last[15]; char first[15]; char middle[15]; }; struct payrecord{ int id; struct namerec name; float hours, rate; float regular, overtime; float gross, tax_withheld, net; Given the above declaration, let payroll data record be stored in a structure called payrecord. Also define a type called payrecord for the structure data type that houses a payroll data record: typedef struct payrecord payrecord; This program reads data, computes payroll and prints it. Each data record is a structure, and the payroll is an array of structures. Overtime hours are 150% of the rate. (Note: Maximum regular hours for the week is 40.) Tax is withheld 15% if weekly pay is below 500, 28% if pay is below 1000, and 33% otherwise. A summary report prints the total gross pay and tax withheld. The following are the function prototypes: void readName(payrecord payroll[], int i); - reads a single name. void printName(payrecord payroll[], int i); - prints a single name. void printSummary(double…arrow_forwardC PROGRAMMING Given code: struct record{char name[10];int age;};struct record myfriend = {“Dhang”, 24};struct record *ptrtomyfriend = &myfriend; ANSWER THE FOLLOWING: a. Write a C statement showing how to display the value of first member being pointed by ptrtomyfriend, using ptrtomyfriend.b. Write a C statement showing how to display the value of the second member being pointed by ptrtomyfriend. c. Write a C statement showing how to store new value of the members being pointed by ptrtomyfriend.arrow_forwardstruct namerec{ char last[15]; char first[15]; char middle[15]; }; struct payrecord{ int id; struct namerec name; float hours, rate; float regular, overtime; float gross, tax_withheld, net; }; Given the above declaration, let payroll data record be stored in a structure called payrecord. Also define a type called payrecord for the structure data type that houses a payroll data record: typedef struct payrecord payrecord; This program reads data, computes payroll and prints it. Each data record is a structure, and the payroll is an array of structures. Overtime hours are 150% of the rate. (Note: Maximum regular hours for the week is 40.) Tax is withheld 15% if weekly pay is below 500, 28% if pay is below 1000, and 33% otherwise. A summary report prints the total gross pay and tax withheld. The following are the function prototypes: void readName(payrecord payroll[], int i); -…arrow_forward
- Please circle True or False for each of the following statements. C++ A private data member is only accessible through its class’s member functions. TRUE FALSEarrow_forwardFind errors / syntax error. Write line numberarrow_forwardVoid functions do not return any value when they are called.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
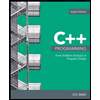
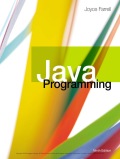