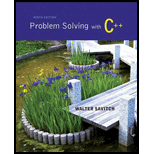
Concept explainers
Define a class called Month that is an abstract data type for a month. Your class will have one member variable of type int to represent a month (1 for January, 2 for February, and so forth). Include all the following member functions: a constructor to set the month using the first three letters in the name of the month as three arguments, a constructor to set the month using an integer as an argument (1 for January, 2 for February, and so forth), a default constructor, an input function that reads the month as an integer, an input function that reads the month as the first three letters in the name of the month, an output function that outputs the month as an integer, an output function that outputs the month as the first three letters in the name of the month, and a member function that returns the next month as a value of type Month. The input and output functions will each have one formal parameter for the stream. Embed your class definition in a test program.

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Problem Solving with C++ (9th Edition)
Additional Engineering Textbook Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Starting Out with Python (4th Edition)
Absolute Java (6th Edition)
Modern Database Management (12th Edition)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
- Rewrite the calculator program using a class called calculator. Your program will keep asking the user if they want to perform more calculations or quit and will have a function displayMenu to display the different functions e.g .(1 - addition, 2- subtraction, 3- multiplication, 4- division) Your program must have appropriate constructors and member functions to initialize, access, and manipulate the data members as well as : A member function to perform the addition and return the result A member function to perform the subtraction and return the result A member function to perform the multiplication and return the result A member function to perform the division and return the resultarrow_forwardProgram SpecificationUsing python, design a class named PersonData with the following member variables: lastName firstName address city state zip phone Write the appropriate accessor and mutator functions for these member variables. Next, design a class named CustomerData , which is derived from the PersonData class. The CustomerData class should have the following member variables: customerNumber mailingList The customerNumber variable will be used to hold a unique integer for each customer. The mailingList variable should be a bool . It will be set to true if the customer wishes to be on a mailing list, or false if the customer does not wish to be on a mail-ing list. Write appropriate accessor and mutator functions for these member variables. Next write a program which demonstrates an object of the CustomerData class in a program. Your program MUST use exception handling. You can choose how to implement the exception handling. Start your program with a welcome message Make sure…arrow_forwardWrite a full class definition for a class named Counter , and containing the following members: A data member counter of type int . A constructor that takes one int argument and assigns its value to counter . A function called increment that accepts no parameters and returns no value. increment adds one to the counter data member. A function called decrement that accepts no parameters and returns no value. decrement subtracts one to the counter data member. A function called getValue that accepts no parameters. It returns the value of the instance variable counter .arrow_forward
- Write a class Box having three private data members (width, depth, height) The class has three constructors which are having no parameter – for setting values to zero or null. having three parameters for assigning values to height, width, depth respectively. Overload the above constructor and use this keyword to set the values of width, height & depth. Provide getters/setters for data members. Write a function calculateVolume() which calculates the volume of the box. Write test Application that demonstrates the Box class by calling all the three constructors and method, creating a Create Box object, and then displaying the Box’swidth , height, length and volume .arrow_forwardPlease solution in c++arrow_forwardSUBJECT: OOPPROGRAMMING LANGUAGE: C++ ALSO ADD SCREENSHOTS OF OUTPUT. Write a class Distance to measure distance in meters and kilometers. The class should have appropriate constructors for initializing members to 0 as well as user provided values. The class should have display function to display the data members on screen. Write another class Time to measure time in hours and minutes. The class should have appropriate constructors for initializing members to 0 as well as user provided values. The class should have display function to display the data members on screen. Write another class which has appropriate functions for taking objects of the Distance class and Time class to store time and distance in a file. Make the data members and functions in your program const where applicablearrow_forward
- There are two types of data members in a class: static and non-static. Provide an example of when it might be useful to have a static data member in the actual world.arrow_forwardCreate an Employee class that includes three private data members— firstName (type string), lastName (type string), and monthlySalary (type int ).It also includes several public member functions.1. A constructor initializes the three data members. 2. A setFirstName function accepts a string parameter and does not return any data. It sets the firstName.3. A getFirstName function does not accept any parameter and returns a string. It returns the firstName.4. A setLastName function accepts a string parameter and does not return any data. It sets the lastName.5. A getLastName function does not accept any parameter and returns a string. It returns the lastName.6. A setMonthlySalary function accepts an integer parameter and does not return any data. It sets the monthlySalary. If the monthly salary is less than or equal zero, set it to 1000 and it displays the employee’s first name, last name and the inputted salary with a statement “**==The salary is set to $1000.”7. A getMonthlySalary…arrow_forwardDesign a class named Month. The class should have the following private members: • name - A string object that holds the name of a month, such as "January", "February", etc. • monthNumber - An integer variable that holds the number of the month. For example, January would be 1, February would be 2, etc. Valid values for this variable are 1 through 12. In addition, provide the following member functions: • A default constructor that sets monthNumber to 1 and name to "January." • A constructor that accepts the name of the month as an argument. It should set name to the value passed as the argument and set monthNumber to the correct value. • A constructor that accepts the number of the month as an argument. It should set monthNumber to the value passed as the argument and set name to the correct month name. • Appropriate set and get functions for the name and monthNumber member variables. • Prefix and postfix overloaded ++ operator functions that increment…arrow_forward
- Design an Account class used to represent an individual’s declining balance gift card. It contains a string for the account number, and a float value for the balance. It should include the following 7 functions: a 2-argument constructor (it accepts an account number and initial balance). a set function, one for each attribute (2 total functions, setAccount and setBalance. Each assigns an argument value to the named member variable. a get function for each attribute (2 total functions, getAccount, and getBalance). Each returns the value stored in the named member variable. a function to display the account’s information to the screen. The account number and balance value should be labelled. a function withdraw that takes a float argument and subtracts it from the balance. Write the class declaration. Member variables should NOT be accessible outside the class! Member functions should be accessible outside the class! Write the member function definitions for only the following…arrow_forwardIn c++, define a class for a type called Counter . An object of this type is used to count things. Include a default constructor that sets the counter to zero and a constructor with one argument that sets the counter to the valuespecified by its argument. Write member functions to increase the value by one (called increment ) and decreasethe value by one (called decrement ), don’t let the value go below 0. Write a member function ( print ) that printsout the value of the counter.Here’s a driver program that you should include to test your class.arrow_forwarda. Instance of a class is called, functions have same name but different sets of parameters.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
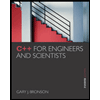
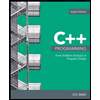
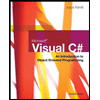