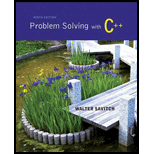
Structure in C++:
Structure is a user defined datatype that is used to combine data items of different data types.
Defining a Structure:
In order to define a structure, the “struct” statement is used. This statement defines a new data type with more than one member.
struct [structure tag] {
member definition;
member definition;
...
Member definition;
}[one or more structure variables];
Here, the “structure tag” is optional and each member definition is a normal variable definition. Specifying one or more structure variables at the end of the structure definition is also optional.
Given:
The following function declaration is given.
void readShoeRecord(ShoeType& newShoe);
//Fills newShoe with values read from the keyboard.

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Problem Solving with C++ (9th Edition)
- float ACM (void); is non-void function with no parameters Select one: O True O Falsearrow_forward13. What is the difference between a formal parameter and an argument? Group of answer choices A function’s argument is referred to as the formal argument to distinguish it from the value that is passed in during the function call. The parameter is the passed value. They are identical but using different terms. A function’s parameter is used for passing by reference. The argument is used for passing by value. A function’s parameter is referred to as the formal parameter to distinguish it from the value that is passed in during the function call. The argument is the passed value. A function’s parameter is used for passing by value. The argument is used for passing by reference.arrow_forwardhello, how would I solve this and could you please explain each step and the reason for it? Thank you so much.arrow_forward
- ***in python only*** use turtle function Define the concentricCircles function such that: It draws a series of concentric circles, where the first parameter specifies the radius of the outermost circle, and the second parameter specifies the number of circles to draw. The third and fourth parameters specify an outer color and an other color, respectively. The outer color is used for the largest (i.e., outermost) circle, and then every other circle out to the edge alternates between that color and the 'other' color. The difference between the radii of subsequent circles is always the same, and this difference is also equal to the radius of the smallest circle. Put another way: the distance between the inside and outside of each ring is the same. Define concentricCircles with 4 parameters Use def to define concentricCircles with 4 parameters Use any kind of loop Within the definition of concentricCircles with 4 parameters, use any kind of loop in at least one place. Call…arrow_forwardPYTHON You Define a Function Part 1: Write a function that takes in one or two input parameters and returns an output. The function should return the output of a one-line expression. Write at least three test cases for your function in the docstring. Make sure your function has just one line of code Part 2: Write the same function as a lambda function.arrow_forwardUH: Question 1: Answer needed in Java programming Language , version : 8 and abovearrow_forward
- Write the functions with the following headers:# Return the reversal of an integer, e.g. reverse(456) returns# 654def reverse(number):# Return true if number is a palindromedef isPalindrome(number): Use the reverse function to implement isPalindrome. A number is a palindrome if its reversal is the same as itself. Write a test program that prompts the user to enter an integer and reports whether the integer is a palindrome.arrow_forwardModify the below code according to what is asking you to do. Show your modified code in a picture, please.arrow_forwardWhat is the difference between overloading a function and redefining a function?arrow_forward
- Help and show me how to fix an error? def kwargs_to_args_decorator(*args, **kwargs): This question is meant to test your knowledge of creating a decorator that accepts an arbitrary number of positional and keyword arguments, to decorate a function that accepts an arbitrary number of positional and keyword arguments, and alters the arguments before passing them to the decorated function. When the decorated function is invoked, this decorator should modify the arguments the decorated function receives. This decorator should filter out all positional arguments passed to the decorated function, which are found in the positional arguments passed to the decorator when the decorator was initialized. It should also filter out all keyword arguments with keys that are found in the keyword arguments given to the decorator when the decorator was initialized. After performing the modifications to the arguments, the decorator should invoke the decorated function with the modified arguments and…arrow_forwardQ4/ Fill with the blank for any three of the following: 1: The function has output(s), while the subroutine has three. one, two, many, 2: The Ode45 function has a than Euler's method. in between. greater accuracy, less accuracy, 3: Given [T,Y] =(odefun, tspan, y0), Tis_____ and Y_ 5 output, input, input or output initial boundary conditions 4: Getframe means pictures. temporary, captures the current axes, none of them. output(s).arrow_forwardThis is not a graded question so please don't disregard it as if it is.Thank you in advance professor! Note: This is a THEORETICAL QUESTION and no code should be used for solving it.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
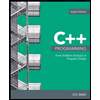