lodify a NissanLeaf subclass (as above): • Set up the constructor so that make is set to "Nissan" and model is set to "Leaf" and year is set to the year of the car. • Override the accelerate method so that it increases the speed by 5. • Override the brake method so that it decreases the speed by 2 (to a minimum of zero).
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Java. Refer to attachment. Starter code below.
public class Car
{
//attributes
private String make;
private String model;
private int year;
protected int speed;
//constructor
public Car(String make, String model, int year)
{
this.make = make;
this.model = model;
this.speed = 0;
this.year = year;
}
//Getters
public String getMake() {return make;}
public String getModel() {return model;}
public int getSpeed() {return speed;}
public int getYear() {return year;}
//Setters
public void setMake(String colour) {this.make = make;}
public void setModel(String colour){this.model = model;}
public void setSpeed(int speed) {this.speed = speed;}
public void setYear(int year) {this.year = year;}
public void accelerate() {
speed ++;
}
public void brake() {
speed --;
if (speed < 0) {speed = 0;}
}
public String toString() {
String carDetails = "Make: " + this.make
+ "\nModel: " + this.model
+ "\nYear: " + this.year
+ "\nSpeed: " + this.speed;
return carDetails;
}
}
public class NissanLeaf extends Car {
//constructor
//override accelerate
//override brake
}
import java.util.Scanner;
public class PoD
{
public static void main (String [] arg )
{
Scanner in = new Scanner( System.in );
int num = in.nextInt();
String carType = in.next();
int carYear = in.nextInt();
Car newCar;
if (carType.equals("NissanLeaf"))
{
newCar = new NissanLeaf(carYear);
}
else
{
String make = carType;
String model = in.next();
newCar = new Car(make,model,carYear);
}
for (int i=0; i< num; i++) {newCar.accelerate();}
for (int i=0; i< num/2; i++) {newCar.brake();}
System.out.println(newCar);
in.close();
}
}



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

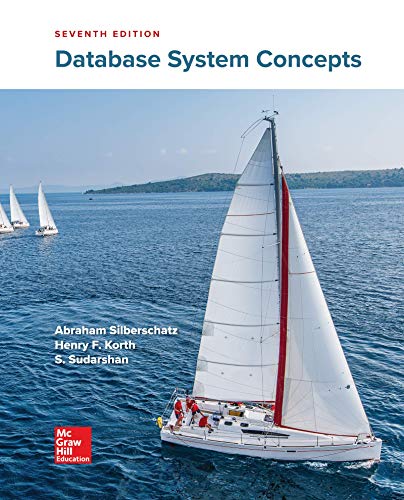
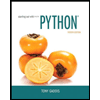
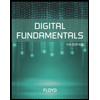
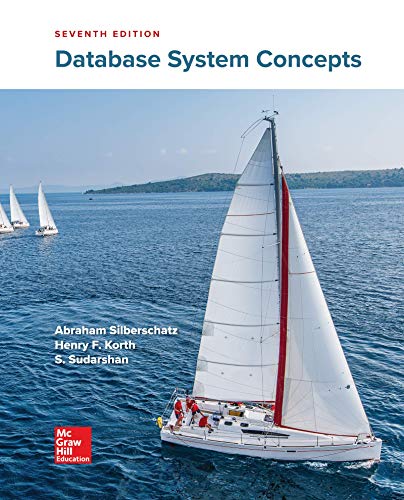
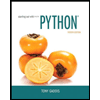
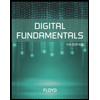
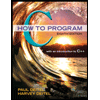
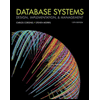
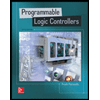