I am having trouble with the folloiwng MATLAB code. I am getting an error that says "unrecognized function or variable 'numericalPropogatorOptions". I have the aerospace toolbox and the aerospace blockset added. what add on do I have to download to use that function. How do I make this code work? % Define Keplerian Elements a = 29599.8; e = 0.0001; i = 0.9774; Omega = 1.3549; w = 0; M = 0.2645; [RECI, VECI] = Kepler2RV(a, e, i, Omega, w, M); initialState = [RECI * 1e3; VECI * 1e3]; % Initial position (m) and velocity (m/s) % Define constants mu = 3.986004418e14; % Gravitational constant (m^3/s^2) earthRadius = 6378.1363 * 1e3; % Earth radius in meters j2 = 1.08263e-3; % J2 perturbation coefficient % Define propagator options propOptions = numericalPropagatorOptions('CentralBody', 'Earth', ... 'GravitationalParameter', mu, ... 'InitialState', initialState, ... 'OutputTimeStep', 300); % Output every 300 seconds % Add perturbations addGravityModel(propOptions, 'Degree', 2, 'Order', 0); % J2 effect addDragModel(propOptions, 'AtmosphereModel', 'Exponential', ... 'ReferenceArea', 1.0, 'DragCoefficient', 2.2, ... 'Mass', 500); % Atmospheric drag addSolarRadiationPressureModel(propOptions, 'ReferenceArea', 1.0, ... 'Reflectivity', 1.3, 'Mass', 500); % SRP addThirdBodyGravity(propOptions, 'Moon'); % Third-body effect from the Moon addThirdBodyGravity(propOptions, 'Sun'); % Third-body effect from the Sun % Propagate the orbit endTime = 300 * 300; % 300 steps, each of 300 seconds [t, state] = numericalPropagator(endTime, propOptions); % Extract position and velocity for plotting position = state(:, 1:3); % Position in ECI (meters) velocity = state(:, 4:6); % Velocity in ECI (meters/second) % Plot the orbit plot3(position(:, 1), position(:, 2), position(:, 3)); xlabel('X (m)'); ylabel('Y (m)'); zlabel('Z (m)'); title('Simulated Orbit with Perturbations in ECI'); grid on; function [RECI, VECI] = Kepler2RV(a, e, i, Omega, w, M) mu = 398600.441799999971; % Earth's Standard Gravitational Parameter (GM) Eps = 2.22044604925031e-8; % Machine epsilon E = SolveKepler(M,e,Eps); % Eccentric Anomaly from Mean Anomaly M = 2*atan(sqrt((1+e)/(1-e))*tan(E/2)); % True Anomaly obtained from Eccentric Anomaly p = a*(1 - e^2); % Semiparameter (km) % Position Coordinates in Perifocal Coordinate System x = (p*cos(M)) / (1 + e*cos(M)); % x-coordinate (km) y = (p*sin(M)) / (1 + e*cos(M)); % y-coordinate (km) z = 0; % z-coordinate (km) vx = -(mu/p)^(1/2) * sin(M); % velocity in x (km/s) vy = (mu/p)^(1/2) * (e + cos(M)); % velocity in y (km/s) vz = 0; % velocity in z (km/s) % Transformation Matrix (3 Rotations) t_rot = [cos(Omega)*cos(w)-sin(Omega)*sin(w)*cos(i) ... (-1)*cos(Omega)*sin(w)-sin(Omega)*cos(w)*cos(i) ... sin(Omega)*sin(i); ... sin(Omega)*cos(w)+cos(Omega)*sin(w)*cos(i) ... (-1)*sin(Omega)*sin(w)+cos(Omega)*cos(w)*cos(i) ... (-1)*cos(Omega)*sin(i); ... sin(w)*sin(i) cos(w)*sin(i) cos(i)]; % Transformation Perifocal xyz to ECI RECI = t_rot*[x y z]'; VECI = t_rot*[vx vy vz]'; end function E = SolveKepler(M,e,Eps) % Iterative Solution of Kepler's equation (M = E-e*sin(E)) %========================================================================== % Inputs: M = Mean Anomaly (rad) % e = Eccentricity % Eps = Machine epsilon % Output: E = Eccentric Anomaly (rad) %========================================================================== E0 = M; E1 = E0 - (E0 - e*sin(E0) - M)/(1 -e*cos(E0)); while (abs(E1-E0) > Eps) E0 = E1; E1 = E0 - (E0 - e*sin(E0) - M)/(1 - e*cos(E0)); end E = E1; end
I am having trouble with the folloiwng MATLAB code. I am getting an error that says "unrecognized function or variable 'numericalPropogatorOptions". I have the aerospace toolbox and the aerospace blockset added. what add on do I have to download to use that function. How do I make this code work? % Define Keplerian Elements a = 29599.8; e = 0.0001; i = 0.9774; Omega = 1.3549; w = 0; M = 0.2645; [RECI, VECI] = Kepler2RV(a, e, i, Omega, w, M); initialState = [RECI * 1e3; VECI * 1e3]; % Initial position (m) and velocity (m/s) % Define constants mu = 3.986004418e14; % Gravitational constant (m^3/s^2) earthRadius = 6378.1363 * 1e3; % Earth radius in meters j2 = 1.08263e-3; % J2 perturbation coefficient % Define propagator options propOptions = numericalPropagatorOptions('CentralBody', 'Earth', ... 'GravitationalParameter', mu, ... 'InitialState', initialState, ... 'OutputTimeStep', 300); % Output every 300 seconds % Add perturbations addGravityModel(propOptions, 'Degree', 2, 'Order', 0); % J2 effect addDragModel(propOptions, 'AtmosphereModel', 'Exponential', ... 'ReferenceArea', 1.0, 'DragCoefficient', 2.2, ... 'Mass', 500); % Atmospheric drag addSolarRadiationPressureModel(propOptions, 'ReferenceArea', 1.0, ... 'Reflectivity', 1.3, 'Mass', 500); % SRP addThirdBodyGravity(propOptions, 'Moon'); % Third-body effect from the Moon addThirdBodyGravity(propOptions, 'Sun'); % Third-body effect from the Sun % Propagate the orbit endTime = 300 * 300; % 300 steps, each of 300 seconds [t, state] = numericalPropagator(endTime, propOptions); % Extract position and velocity for plotting position = state(:, 1:3); % Position in ECI (meters) velocity = state(:, 4:6); % Velocity in ECI (meters/second) % Plot the orbit plot3(position(:, 1), position(:, 2), position(:, 3)); xlabel('X (m)'); ylabel('Y (m)'); zlabel('Z (m)'); title('Simulated Orbit with Perturbations in ECI'); grid on; function [RECI, VECI] = Kepler2RV(a, e, i, Omega, w, M) mu = 398600.441799999971; % Earth's Standard Gravitational Parameter (GM) Eps = 2.22044604925031e-8; % Machine epsilon E = SolveKepler(M,e,Eps); % Eccentric Anomaly from Mean Anomaly M = 2*atan(sqrt((1+e)/(1-e))*tan(E/2)); % True Anomaly obtained from Eccentric Anomaly p = a*(1 - e^2); % Semiparameter (km) % Position Coordinates in Perifocal Coordinate System x = (p*cos(M)) / (1 + e*cos(M)); % x-coordinate (km) y = (p*sin(M)) / (1 + e*cos(M)); % y-coordinate (km) z = 0; % z-coordinate (km) vx = -(mu/p)^(1/2) * sin(M); % velocity in x (km/s) vy = (mu/p)^(1/2) * (e + cos(M)); % velocity in y (km/s) vz = 0; % velocity in z (km/s) % Transformation Matrix (3 Rotations) t_rot = [cos(Omega)*cos(w)-sin(Omega)*sin(w)*cos(i) ... (-1)*cos(Omega)*sin(w)-sin(Omega)*cos(w)*cos(i) ... sin(Omega)*sin(i); ... sin(Omega)*cos(w)+cos(Omega)*sin(w)*cos(i) ... (-1)*sin(Omega)*sin(w)+cos(Omega)*cos(w)*cos(i) ... (-1)*cos(Omega)*sin(i); ... sin(w)*sin(i) cos(w)*sin(i) cos(i)]; % Transformation Perifocal xyz to ECI RECI = t_rot*[x y z]'; VECI = t_rot*[vx vy vz]'; end function E = SolveKepler(M,e,Eps) % Iterative Solution of Kepler's equation (M = E-e*sin(E)) %========================================================================== % Inputs: M = Mean Anomaly (rad) % e = Eccentricity % Eps = Machine epsilon % Output: E = Eccentric Anomaly (rad) %========================================================================== E0 = M; E1 = E0 - (E0 - e*sin(E0) - M)/(1 -e*cos(E0)); while (abs(E1-E0) > Eps) E0 = E1; E1 = E0 - (E0 - e*sin(E0) - M)/(1 - e*cos(E0)); end E = E1; end
Elements Of Electromagnetics
7th Edition
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Sadiku, Matthew N. O.
ChapterMA: Math Assessment
Section: Chapter Questions
Problem 1.1MA
Related questions
Question
I am having trouble with the folloiwng MATLAB code. I am getting an error that says "unrecognized function or variable 'numericalPropogatorOptions". I have the aerospace toolbox and the aerospace blockset added. what add on do I have to download to use that function. How do I make this code work?
% Define Keplerian Elements
a = 29599.8; e = 0.0001; i = 0.9774; Omega = 1.3549; w = 0; M = 0.2645;
[RECI, VECI] = Kepler2RV(a, e, i, Omega, w, M);
initialState = [RECI * 1e3; VECI * 1e3]; % Initial position (m) and velocity (m/s)
% Define constants
mu = 3.986004418e14; % Gravitational constant (m^3/s^2)
earthRadius = 6378.1363 * 1e3; % Earth radius in meters
j2 = 1.08263e-3; % J2 perturbation coefficient
% Define propagator options
propOptions = numericalPropagatorOptions('CentralBody', 'Earth', ...
'GravitationalParameter', mu, ...
'InitialState', initialState, ...
'OutputTimeStep', 300); % Output every 300 seconds
% Add perturbations
addGravityModel(propOptions, 'Degree', 2, 'Order', 0); % J2 effect
addDragModel(propOptions, 'AtmosphereModel', 'Exponential', ...
'ReferenceArea', 1.0, 'DragCoefficient', 2.2, ...
'Mass', 500); % Atmospheric drag
addSolarRadiationPressureModel(propOptions, 'ReferenceArea', 1.0, ...
'Reflectivity', 1.3, 'Mass', 500); % SRP
addThirdBodyGravity(propOptions, 'Moon'); % Third-body effect from the Moon
addThirdBodyGravity(propOptions, 'Sun'); % Third-body effect from the Sun
% Propagate the orbit
endTime = 300 * 300; % 300 steps, each of 300 seconds
[t, state] = numericalPropagator(endTime, propOptions);
% Extract position and velocity for plotting
position = state(:, 1:3); % Position in ECI (meters)
velocity = state(:, 4:6); % Velocity in ECI (meters/second)
% Plot the orbit
plot3(position(:, 1), position(:, 2), position(:, 3));
xlabel('X (m)'); ylabel('Y (m)'); zlabel('Z (m)');
title('Simulated Orbit with Perturbations in ECI');
grid on;
function [RECI, VECI] = Kepler2RV(a, e, i, Omega, w, M)
mu = 398600.441799999971; % Earth's Standard Gravitational Parameter (GM)
Eps = 2.22044604925031e-8; % Machine epsilon
E = SolveKepler(M,e,Eps); % Eccentric Anomaly from Mean Anomaly
M = 2*atan(sqrt((1+e)/(1-e))*tan(E/2)); % True Anomaly obtained from Eccentric Anomaly
p = a*(1 - e^2); % Semiparameter (km)
% Position Coordinates in Perifocal Coordinate System
x = (p*cos(M)) / (1 + e*cos(M)); % x-coordinate (km)
y = (p*sin(M)) / (1 + e*cos(M)); % y-coordinate (km)
z = 0; % z-coordinate (km)
vx = -(mu/p)^(1/2) * sin(M); % velocity in x (km/s)
vy = (mu/p)^(1/2) * (e + cos(M)); % velocity in y (km/s)
vz = 0; % velocity in z (km/s)
% Transformation Matrix (3 Rotations)
t_rot = [cos(Omega)*cos(w)-sin(Omega)*sin(w)*cos(i) ...
(-1)*cos(Omega)*sin(w)-sin(Omega)*cos(w)*cos(i) ...
sin(Omega)*sin(i); ...
sin(Omega)*cos(w)+cos(Omega)*sin(w)*cos(i) ...
(-1)*sin(Omega)*sin(w)+cos(Omega)*cos(w)*cos(i) ...
(-1)*cos(Omega)*sin(i); ...
sin(w)*sin(i) cos(w)*sin(i) cos(i)];
% Transformation Perifocal xyz to ECI
RECI = t_rot*[x y z]';
VECI = t_rot*[vx vy vz]';
end
function E = SolveKepler(M,e,Eps)
% Iterative Solution of Kepler's equation (M = E-e*sin(E))
%==========================================================================
% Inputs: M = Mean Anomaly (rad)
% e = Eccentricity
% Eps = Machine epsilon
% Output: E = Eccentric Anomaly (rad)
%==========================================================================
E0 = M;
E1 = E0 - (E0 - e*sin(E0) - M)/(1 -e*cos(E0));
while (abs(E1-E0) > Eps)
E0 = E1;
E1 = E0 - (E0 - e*sin(E0) - M)/(1 - e*cos(E0));
end
E = E1;
end
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
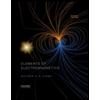
Elements Of Electromagnetics
Mechanical Engineering
ISBN:
9780190698614
Author:
Sadiku, Matthew N. O.
Publisher:
Oxford University Press
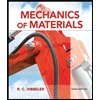
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:
9780134319650
Author:
Russell C. Hibbeler
Publisher:
PEARSON
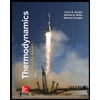
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:
9781259822674
Author:
Yunus A. Cengel Dr., Michael A. Boles
Publisher:
McGraw-Hill Education
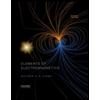
Elements Of Electromagnetics
Mechanical Engineering
ISBN:
9780190698614
Author:
Sadiku, Matthew N. O.
Publisher:
Oxford University Press
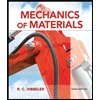
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:
9780134319650
Author:
Russell C. Hibbeler
Publisher:
PEARSON
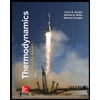
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:
9781259822674
Author:
Yunus A. Cengel Dr., Michael A. Boles
Publisher:
McGraw-Hill Education
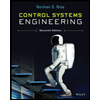
Control Systems Engineering
Mechanical Engineering
ISBN:
9781118170519
Author:
Norman S. Nise
Publisher:
WILEY
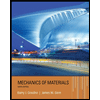
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:
9781337093347
Author:
Barry J. Goodno, James M. Gere
Publisher:
Cengage Learning
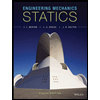
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:
9781118807330
Author:
James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:
WILEY