After compiling these files I get the follwoing error: IntCollection.cpp:107:51: error: no ‘IntCollection& IntCollection::operator<<(int)’ member function declared in class ‘IntCollection’ IntCollection &IntCollection::operator<<(int value) ^ In file included from collectionMain.cpp:2:0: IntCollection.cpp:107:51: error: no ‘IntCollection& IntCollection::operator<<(int)’ member function declared in class ‘IntCollection’ IntCollection &IntCollection::operator<<(int value) ^ Can you please help me to get eliminate this error so I can compile it? Thank you. Two files were typed and the .h file was screenshoted along with the error. FILE: IntCollection.cpp #include "IntCollection.h" #include using namespace std; IntCollection::IntCollection() { //initialize member data to reflect an empty IntCollection size = 0; capacity = 0; data = NULL; } //Descructor: program is the memory deallocated with delete [] IntCollection::~IntCollection() { delete[] data; } //The copy constructor should perform a deep copy of the argument object, //i.e.it should construct an IntCollection with the same size and capacity as the argument, //with its own complete copy of the argument's data array. IntCollection::IntCollection(const IntCollection &c) { size = c.size; capacity = c.capacity; data = c.data; for (int i = 0; i < c.size; i++) { data[i] = c.data[i]; } } //create a new, bigger buffer, copy the current data to it, delete //the old buffer, and point our data pointer to the new buffer void IntCollection::addCapacity() { int *newData; capacity += CHUNK_SIZE; newData = new int[capacity]; for (int i = 0; i < size; i++) newData[i] = data[i]; delete[] data; data = newData; } void IntCollection::add(int value) { //first, allocate more memory if we need to if (size == capacity) addCapacity(); //now, add the data to our array and increment size data[size++] = value; } int IntCollection::get(int index) { if (index < 0 || index >= size) { cout << "ERROR: get() trying to access index out of range.\n"; exit(1); } return data[index]; } int IntCollection::getSize() { return size; } //The assignment operator should also perform a deep copy of the argument object. IntCollection &IntCollection::operator=(const IntCollection &c) { size = c.size; capacity = c.capacity; data = c.data; return *this; } //The "is equals" operator returns true //if the argument object has the same size as the receiving object, // and the values in both objects’ data arrays are identical. bool IntCollection::operator==(const IntCollection &c) { if ((size == c.size) && (capacity == c.capacity)) { for (int i = 0; i < size; i++) { if (data[i] != c.data[i]) { return false; } } } else { return false; } return true; } //The "is equals" operator returns true //if the argument object has the same size as the receiving object, // and the values in both objects’ data arrays are identical. bool IntCollection::operator==(const IntCollection &c) { if ((size == c.size) && (capacity == c.capacity)) { for (int i = 0; i < size; i++) { if (data[i] != c.data[i]) { return false; } } } else { return false; } return true; } //The insertion operator should add the int parameter into the receiving IntCollection. //The functionality is the same as the add() function, i.e.add ints to the collection. IntCollection &IntCollection::operator << (int value) { add(value); return *this; } FILE: IntCollection.h #ifndef INTCOLLECTION_H #define INTCOLLECTION_H const int CHUNK_SIZE=5; // allocate memory in chunks of ints of this size class IntCollection { private: int size; // the number of ints currently stored in the int collection int capacity; // the total number of elements available for stoarge in the data array int* data; // a pointer to the dynamically allocated data array void addCapacity(); // a private member function to allocate more memory if necessary public: IntCollection(); // constructor ~IntCollection(); // destructor, to be added! IntCollection(const IntCollection &c); // copy constructor, to be added! void add(int value); int get(int index); int getSize(); IntCollection& operator=(const IntCollection &c); // to be added! bool operator==(const IntCollection &c); // to be added! //IntCollection& operator<<(IntCollection &a,int value); // to be added! friend IntCollection& operator<<(IntCollection& out, int ); }; #endif
IntCollection.cpp:107:51: error: no ‘IntCollection& IntCollection::operator<<(int)’ member function declared in class ‘IntCollection’
IntCollection &IntCollection::operator<<(int value)
^
In file included from collectionMain.cpp:2:0:
IntCollection.cpp:107:51: error: no ‘IntCollection& IntCollection::operator<<(int)’ member function declared in class ‘IntCollection’
IntCollection &IntCollection::operator<<(int value)
^
FILE: IntCollection.h
#ifndef INTCOLLECTION_H
#define INTCOLLECTION_H
const int CHUNK_SIZE=5; // allocate memory in chunks of ints of this size
class IntCollection
{
private:
int size; // the number of ints currently stored in the int collection
int capacity; // the total number of elements available for stoarge in the data array
int* data; // a pointer to the dynamically allocated data array
void addCapacity(); // a private member function to allocate more memory if necessary
public:
IntCollection(); // constructor
~IntCollection(); // destructor, to be added!
IntCollection(const IntCollection &c); // copy constructor, to be added!
void add(int value);
int get(int index);
int getSize();
IntCollection& operator=(const IntCollection &c); // to be added!
bool operator==(const IntCollection &c); // to be added!
//IntCollection& operator<<(IntCollection &a,int value); // to be added!
friend IntCollection& operator<<(IntCollection& out, int );
};
#endif


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

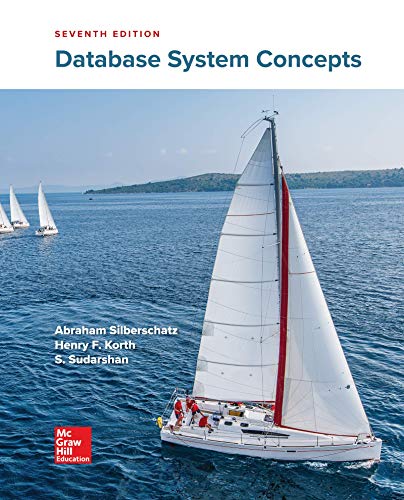
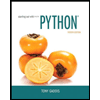
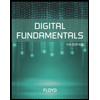
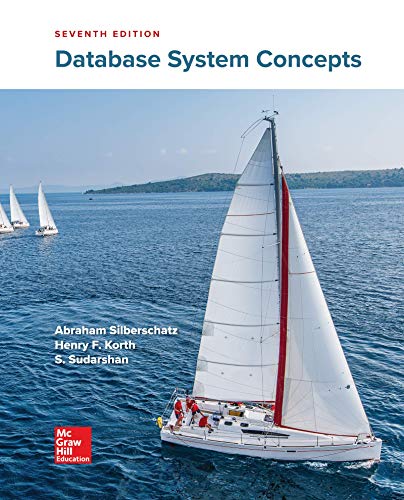
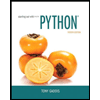
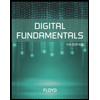
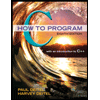
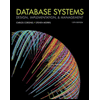
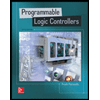