1. Copy the previous program to a new file. #include using namespace std; class Vehicle { private: string brand; public: Vehicle() { this->brand = "TBD"; } Vehicle(string brand) { this->brand = brand; } void output() { cout << "Brand: " << this->brand << endl; } string getBrand() const { return brand; } void setBrand(const string &brand_) { brand = brand_; } }; int main() { Vehicle a, b("BMW"); a.output(); b.output(); a.setBrand("Tesla"); cout << a.getBrand() << endl; }
1. Copy the previous program to a new file.
#include <iostream>
using namespace std;
class Vehicle
{
private:
string brand;
public:
Vehicle()
{
this->brand = "TBD";
}
Vehicle(string brand)
{
this->brand = brand;
}
void output()
{
cout << "Brand: " << this->brand << endl;
}
string getBrand() const { return brand; }
void setBrand(const string &brand_) { brand = brand_; }
};
int main()
{
Vehicle a, b("BMW");
a.output();
b.output();
a.setBrand("Tesla");
cout << a.getBrand() << endl;
}
that's my previous code
2. Create a class named Car derived from Vehicle.
a. Private data member: weight — dynamic member int
b. Implement default and two argument constructors
c. Redefine output function
d. Mutator and accessor function for weight
3. Create a class named Boat derived from Vehicle.
a. Private data member: hullLength — dynamic member int
b. Implement default and two argument constructors
c. Redefine output function
d. Mutator and accessor function for hullLength
Use following main() to test your function.
int main(){
Car a,b("Honda",2000);
a.output(); // Brand:TBD Weight: 0
b.output(); // Brand:Honda Weight: 2000
b.setBrand("Tesla");
b.setWeight(3000);
b.output(); // Brand:Tesla Weight: 3000
Boat c,d("Baja",100);
c.output(); // Brand:TBD hullLength: 0
d.output(); // Brand:Baja hullLength: 100
d.setBrand("Bertram");
d.sethullLength(60);
d.output(); // Brand:Bertram hullLength: 60
}
Output from main:
Brand:TBD Weight: 0
Brand:Honda Weight: 2000
Brand:Tesla Weight: 3000
Brand:TBD hullLength: 0
Brand:Baja hullLength: 100
Brand:Bertram hullLength: 60

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

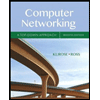
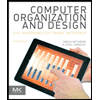
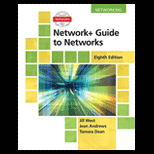
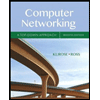
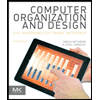
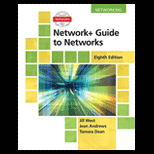
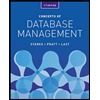
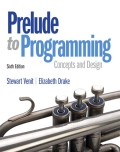
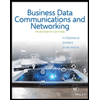