C PROGRAMMING HELP I need help fixing an old assignment of mine. The point of the script is to read the pasted/attached Number_new.txt file's contents, meant to represent a classes' final grades out of 100 percent, and print an .exe screen as well as another .txt file that repeats the grade number with a letter grade and a PASS or FAIL next to it. At the end it needs to say how many students passed and how many failed. Nothing too complicated with the grading, just 90.0 and up is an A, 80.0 to 89.99 is a B, etc. And of course, anything below 60.0 is an F/FAIL. Please NO IOSTREAM.H OR CACIO.H! I cant use those header files. Below is the script: #include #include #define THEFILE "Number_new.txt" int main(){ float r0[10]; float rr[10]; FILE *fp; char line[81]; fp = fopen(THEFILE, "Number_new.txt"); if (fp != NULL) { /* The file is open. */ printf("%7s %7s %7s %7s %7s %7s %7s %7s %7s %7s\n", "0", "0.01", "0.02","0.03", "0.04", "0.05","0.06", "0.07", "0.08","0.09"); while (fgets(line, sizeof(line), fp)) { sscanf (line, "%f %f %f %f %f %f %f %f %f %f\n", &r0[0], &r0[1], &r0[2], &r0[3], &r0[4], &r0[5], &r0[6], &r0[7], &r0[8], &r0[9]); //sscanf (line, "%7f", &rr[0]); //printf() printf("%0.5f %0.5f %0.5f %0.5f %0.5f %0.5f %0.5f %0.5f %0.5f %0.5f\n", r0[0], r0[1], r0[2], r0[3], r0[4], r0[5], r0[6], r0[7], r0[8], r0[9]); //printf("% 0.5f", rr[0]); } } else { /* File could not be opened. */ printf ("The file could not be opened.\n"); return 1; /* Exit the function/application. */ } if (fclose(fp) != 0) { printf ("The file could not be closed.\n"); } getchar(); /* Pause. */ return 0; } And the .txt file's contents pasted: 96.23 85.87 95.14 98.82 71.38 80.10 52.16 93.12 83.30 94.75 53.51 79.74 53.05 79.70 61.71 92.01 95.30 96.40 97.66 96.36 97.90 96.97 89.24 90.38 90.11 87.66 98.43 81.57 79.29 75.78 37.86 62.55 2.75 18.24 26.38 29.81 47.96 47.35 28.27 20.51 28.81 23.72 50.50 30.48 65.96 85.39 0.74 40.22 95.00 43.99 54.59 70.54 97.48 79.01 98.68 82.13 72.73 48.82 18.39 22.99 70.35 60.32 89.73 49.39 7.13 83.61 63.43 22.87 17.33 82.09 93.23 70.58 30.92 75.32 28.09 72.11 37.12 92.63 26.82 48.48 91.54 77.28 83.94 1.89 95.92 99.49 91.43 14.64 23.81 21.07 67.01 7.50 72.65 76.54 48.36 96.38 66.76 10.94 53.61 81.72 42.53 73.54 93.49 53.14 91.50 92.01 42.55 71.70 90.48 22.66 74.91 84.74 21.81 96.56 82.70 62.30 88.50 73.52 42.54 58.22 49.20 74.93 61.28 95.20 59.84 81.17 50.26 34.46 13.56 21.61 64.71 91.23 75.10 63.00 17.54 94.01 88.25 70.43 56.71 97.88 88.28 47.35 38.21 68.46 96.50 73.00 95.78 24.69 19.34 50.59 85.01 97.33 47.15 53.68 71.52 90.67 82.31 21.01 49.17 76.62 97.58 11.96 81.07 98.07 31.49 30.56 83.61 88.35 91.22 93.98 50.38 81.35 91.22 82.12 87.33 53.58 58.76 93.02 73.30 78.98 99.28 87.50 98.96 57.69 65.05 31.60 18.34 10.23 53.73 32.19 88.42 96.82 87.13 77.71 76.65 85.44 78.09 89.98 77.57 95.70 Thank you for your help!
C PROGRAMMING HELP
I need help fixing an old assignment of mine.
The point of the script is to read the pasted/attached Number_new.txt file's contents, meant to represent a classes' final grades out of 100 percent, and print an .exe screen as well as another .txt file that repeats the grade number with a letter grade and a PASS or FAIL next to it.
At the end it needs to say how many students passed and how many failed.
Nothing too complicated with the grading, just 90.0 and up is an A, 80.0 to 89.99 is a B, etc. And of course, anything below 60.0 is an F/FAIL.
Please NO IOSTREAM.H OR CACIO.H! I cant use those header files.
Below is the script:
#include<stdio.h>
#include<math.h>
#define THEFILE "Number_new.txt"
int main(){
float r0[10];
float rr[10];
FILE *fp;
char line[81];
fp = fopen(THEFILE, "Number_new.txt");
if (fp != NULL) { /* The file is open. */
printf("%7s %7s %7s %7s %7s %7s %7s %7s %7s %7s\n", "0", "0.01", "0.02","0.03", "0.04", "0.05","0.06", "0.07", "0.08","0.09");
while (fgets(line, sizeof(line), fp)) {
sscanf (line, "%f %f %f %f %f %f %f %f %f %f\n", &r0[0], &r0[1], &r0[2], &r0[3], &r0[4], &r0[5], &r0[6], &r0[7], &r0[8], &r0[9]);
//sscanf (line, "%7f", &rr[0]);
//printf()
printf("%0.5f %0.5f %0.5f %0.5f %0.5f %0.5f %0.5f %0.5f %0.5f %0.5f\n", r0[0], r0[1], r0[2], r0[3], r0[4], r0[5], r0[6], r0[7], r0[8], r0[9]);
//printf("% 0.5f", rr[0]);
}
} else { /* File could not be opened. */
printf ("The file could not be opened.\n");
return 1; /* Exit the function/application. */
}
if (fclose(fp) != 0) {
printf ("The file could not be closed.\n");
}
getchar(); /* Pause. */
return 0;
}
And the .txt file's contents pasted:
96.23 85.87 95.14 98.82 71.38
80.10 52.16 93.12 83.30 94.75
53.51 79.74 53.05 79.70 61.71
92.01 95.30 96.40 97.66 96.36
97.90 96.97 89.24 90.38 90.11
87.66 98.43 81.57 79.29 75.78
37.86 62.55 2.75 18.24 26.38
29.81 47.96 47.35 28.27 20.51
28.81 23.72 50.50 30.48 65.96
85.39 0.74 40.22 95.00 43.99
54.59 70.54 97.48 79.01 98.68
82.13 72.73 48.82 18.39 22.99
70.35 60.32 89.73 49.39 7.13
83.61 63.43 22.87 17.33 82.09
93.23 70.58 30.92 75.32 28.09
72.11 37.12 92.63 26.82 48.48
91.54 77.28 83.94 1.89 95.92
99.49 91.43 14.64 23.81 21.07
67.01 7.50 72.65 76.54 48.36
96.38 66.76 10.94 53.61 81.72
42.53 73.54 93.49 53.14 91.50
92.01 42.55 71.70 90.48 22.66
74.91 84.74 21.81 96.56 82.70
62.30 88.50 73.52 42.54 58.22
49.20 74.93 61.28 95.20 59.84
81.17 50.26 34.46 13.56 21.61
64.71 91.23 75.10 63.00 17.54
94.01 88.25 70.43 56.71 97.88
88.28 47.35 38.21 68.46 96.50
73.00 95.78 24.69 19.34 50.59
85.01 97.33 47.15 53.68 71.52
90.67 82.31 21.01 49.17 76.62
97.58 11.96 81.07 98.07 31.49
30.56 83.61 88.35 91.22 93.98
50.38 81.35 91.22 82.12 87.33
53.58 58.76 93.02 73.30 78.98
99.28 87.50 98.96 57.69 65.05
31.60 18.34 10.23 53.73 32.19
88.42 96.82 87.13 77.71 76.65
85.44 78.09 89.98 77.57 95.70
Thank you for your help!

Step by step
Solved in 7 steps with 3 images

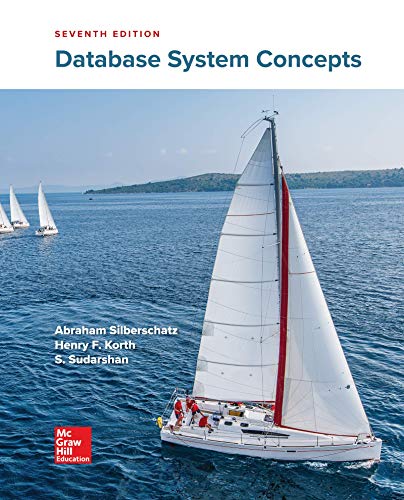
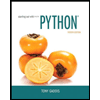
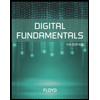
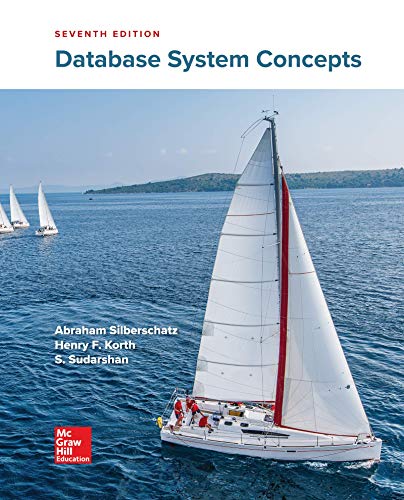
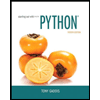
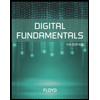
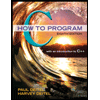
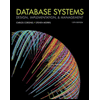
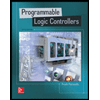