C++ Coding Help (Operators: new delete) Assign pointer myGas with a new Gas object. Call myGas's Read() to read the object's fields. Then, call myGas's Print() to output the values of the fields. Finally, delete myGas. Ex: If the input is 14 45, then the output is: Gas's volume: 14 Gas's temperature: 45 Gas with volume 14 and temperature 45 is deallocated.
C++ Coding Help (Operators: new delete)
Assign pointer myGas with a new Gas object. Call myGas's Read() to read the object's fields. Then, call myGas's Print() to output the values of the fields. Finally, delete myGas.
Ex: If the input is 14 45, then the output is:
Gas's volume: 14 Gas's temperature: 45 Gas with volume 14 and temperature 45 is deallocated.
#include <iostream>
using namespace std;
class Gas {
public:
Gas();
void Read();
void Print();
~Gas();
private:
int volume;
int temperature;
};
Gas::Gas() {
volume = 0;
temperature = 0;
}
void Gas::Read() {
cin >> volume;
cin >> temperature;
}
void Gas::Print() {
cout << "Gas's volume: " << volume << endl;
cout << "Gas's temperature: " << temperature << endl;
}
Gas::~Gas() { // Covered in section on Destructors.
cout << "Gas with volume " << volume << " and temperature " << temperature << " is deallocated." << endl;
}
int main() {
Gas* myGas = nullptr;
/* CODE HELP SOLUTION GOES HERE */
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

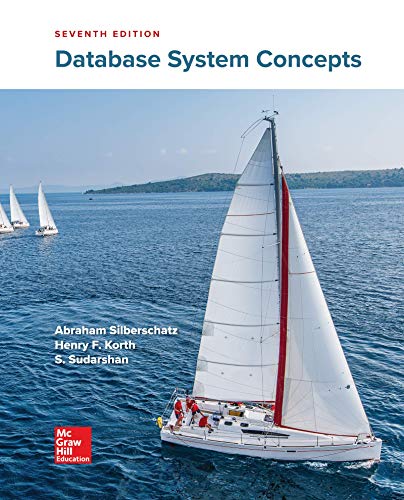
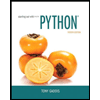
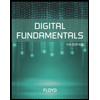
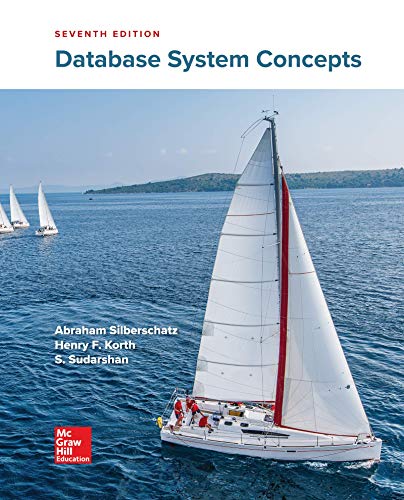
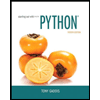
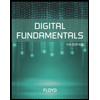
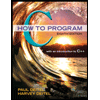
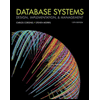
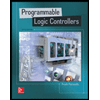