Write in C++ Sam is making a list of his favorite Pokemon. However, he changes his mind a lot. Help Sam write a function insertAfter() that takes five parameters and inserts the name of a Pokemon right after a specific index. Function specifications Name: insertAfter() Parameters (Your function should accept these parameters IN THIS ORDER): input_strings string: The array containing strings num_elements int: The number of elements that are currently stored in the array arr_size int: The number of elements that can be stored in the array index int: The location to insert a new string. Note that the new string should be inserted after this location. string_to_insert string: The new string to be inserted into the array Return Value: bool: true: If the string is successfully inserted into the array false: If the array is full If the index value exceeds the size of the array
Write in C++ Sam is making a list of his favorite Pokemon. However, he changes his mind a lot. Help Sam write a function insertAfter() that takes five parameters and inserts the name of a Pokemon right after a specific index. Function specifications Name: insertAfter() Parameters (Your function should accept these parameters IN THIS ORDER): input_strings string: The array containing strings num_elements int: The number of elements that are currently stored in the array arr_size int: The number of elements that can be stored in the array index int: The location to insert a new string. Note that the new string should be inserted after this location. string_to_insert string: The new string to be inserted into the array Return Value: bool: true: If the string is successfully inserted into the array false: If the array is full If the index value exceeds the size of the array
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write in C++
Sam is making a list of his favorite Pokemon. However, he changes his mind a lot. Help Sam write a function insertAfter() that takes five parameters and inserts the name of a Pokemon right after a specific index.
Function specifications
- Name: insertAfter()
- Parameters (Your function should accept these parameters IN THIS ORDER):
- input_strings string: The array containing strings
- num_elements int: The number of elements that are currently stored in the array
- arr_size int: The number of elements that can be stored in the array
- index int: The location to insert a new string. Note that the new string should be inserted after this location.
- string_to_insert string: The new string to be inserted into the array
- Return Value: bool:
- true: If the string is successfully inserted into the array
- false:
- If the array is full
- If the index value exceeds the size of the array
![## Function for Inserting a String in a List
Sam is making a list of his favorite Pokémon. However, he changes his mind a lot. Help Sam write a function `insertAfter()` that takes five parameters and inserts the name of a Pokémon right after a specific index.
### Function Specifications
- **Name:** `insertAfter()`
- **Parameters (Your function should accept these parameters IN THIS ORDER):**
- **input_strings** `string[]`: The array containing strings.
- **num_elements** `int`: The number of elements that are currently stored in the array.
- **arr_size** `int`: The number of elements that can be stored in the array.
- **index** `int`: The location to insert a new string. Note that the new string should be inserted *after* this location.
- **string_to_insert** `string`: The new string to be inserted into the array.
- **Return Value:** `bool`
- `true`: If the string is successfully inserted into the array.
- `false`:
- If the array is full.
- If the index value exceeds the size of the array.
### Sample Run 1: Function Call
```cpp
int size = 5;
string input_strings[size] = {"pikachu", "meowth", "snorlax"};
int num_elements = 3;
int index = 2;
string string_to_insert = "clefairy";
// result contains the value returned by insertAfter
bool result = insertAfter(input_strings, num_elements, size, index, string_to_insert);
// print result
cout << "Function returned value: " << result << endl;
// print array contents
for (int i = 0; i < size; i++) {
cout << input_strings[i] << endl;
}
```
### Explanation of Sample Code
- **Initialization:**
- An array `input_strings` is initialized with a capacity of 5, containing "pikachu", "meowth", and "snorlax".
- `num_elements` is set to 3, indicating the current number of items in the array.
- `index` is set to 2, specifying the insertion point.
- `string_to_insert` is set to "clefairy".
- **Function Call:**
- `insertAfter` is called with the array and parameters, returning a boolean value stored](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb1d14486-aab8-45fa-9185-3d15b7af8b4b%2F95d11a29-ef0c-470f-b152-8e47862d1254%2Fu8bov8_processed.png&w=3840&q=75)
Transcribed Image Text:## Function for Inserting a String in a List
Sam is making a list of his favorite Pokémon. However, he changes his mind a lot. Help Sam write a function `insertAfter()` that takes five parameters and inserts the name of a Pokémon right after a specific index.
### Function Specifications
- **Name:** `insertAfter()`
- **Parameters (Your function should accept these parameters IN THIS ORDER):**
- **input_strings** `string[]`: The array containing strings.
- **num_elements** `int`: The number of elements that are currently stored in the array.
- **arr_size** `int`: The number of elements that can be stored in the array.
- **index** `int`: The location to insert a new string. Note that the new string should be inserted *after* this location.
- **string_to_insert** `string`: The new string to be inserted into the array.
- **Return Value:** `bool`
- `true`: If the string is successfully inserted into the array.
- `false`:
- If the array is full.
- If the index value exceeds the size of the array.
### Sample Run 1: Function Call
```cpp
int size = 5;
string input_strings[size] = {"pikachu", "meowth", "snorlax"};
int num_elements = 3;
int index = 2;
string string_to_insert = "clefairy";
// result contains the value returned by insertAfter
bool result = insertAfter(input_strings, num_elements, size, index, string_to_insert);
// print result
cout << "Function returned value: " << result << endl;
// print array contents
for (int i = 0; i < size; i++) {
cout << input_strings[i] << endl;
}
```
### Explanation of Sample Code
- **Initialization:**
- An array `input_strings` is initialized with a capacity of 5, containing "pikachu", "meowth", and "snorlax".
- `num_elements` is set to 3, indicating the current number of items in the array.
- `index` is set to 2, specifying the insertion point.
- `string_to_insert` is set to "clefairy".
- **Function Call:**
- `insertAfter` is called with the array and parameters, returning a boolean value stored
![### Educational Website Content
#### Code Output
```plaintext
Output
Function returned value: 1
pikachu
meowth
snorlax
clefairy
```
This section represents the output from a program execution, displaying a list of characters and the function's returned value.
---
#### Sample Run 2 Function Call
Below is a code snippet demonstrating a sample function call in C++:
```cpp
int size = 5;
string input_strings[size] = {"caterpie", "eevee"};
int num_elements = 2;
int index = 0;
string string_to_insert = "bulbasaur";
// result contains the value returned by insertAfter
bool result = insertAfter(input_strings, num_elements, size, index, string_to_insert);
// print result
cout << "Function returned value: " << result << endl;
// print array contents
for(int i = 0; i < size; i++)
{
cout << input_strings[i] << endl;
}
```
**Description:**
- An array `input_strings` is defined with an initial size of 5 and pre-populated with two elements, `"caterpie"` and `"eevee"`.
- The `string_to_insert`, `"bulbasaur"`, will be inserted into the array.
- The `insertAfter` function attempts to insert the specified string at the given `index`.
- The program then outputs the result of the function call and prints out the contents of the `input_strings` array.
#### Output of the Sample Run 2
```plaintext
Output
Function returned value: 1
caterpie
bulbasaur
eevee
```
The function successfully inserts `"bulbasaur"` after `"caterpie"` and returns a value of 1, indicating success. The printed array shows the updated order. This demonstrates basic array manipulation and function use in C++.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb1d14486-aab8-45fa-9185-3d15b7af8b4b%2F95d11a29-ef0c-470f-b152-8e47862d1254%2Fa3xv8zj_processed.png&w=3840&q=75)
Transcribed Image Text:### Educational Website Content
#### Code Output
```plaintext
Output
Function returned value: 1
pikachu
meowth
snorlax
clefairy
```
This section represents the output from a program execution, displaying a list of characters and the function's returned value.
---
#### Sample Run 2 Function Call
Below is a code snippet demonstrating a sample function call in C++:
```cpp
int size = 5;
string input_strings[size] = {"caterpie", "eevee"};
int num_elements = 2;
int index = 0;
string string_to_insert = "bulbasaur";
// result contains the value returned by insertAfter
bool result = insertAfter(input_strings, num_elements, size, index, string_to_insert);
// print result
cout << "Function returned value: " << result << endl;
// print array contents
for(int i = 0; i < size; i++)
{
cout << input_strings[i] << endl;
}
```
**Description:**
- An array `input_strings` is defined with an initial size of 5 and pre-populated with two elements, `"caterpie"` and `"eevee"`.
- The `string_to_insert`, `"bulbasaur"`, will be inserted into the array.
- The `insertAfter` function attempts to insert the specified string at the given `index`.
- The program then outputs the result of the function call and prints out the contents of the `input_strings` array.
#### Output of the Sample Run 2
```plaintext
Output
Function returned value: 1
caterpie
bulbasaur
eevee
```
The function successfully inserts `"bulbasaur"` after `"caterpie"` and returns a value of 1, indicating success. The printed array shows the updated order. This demonstrates basic array manipulation and function use in C++.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
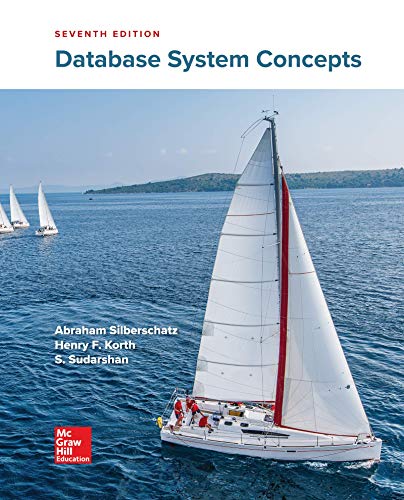
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
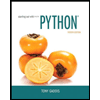
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
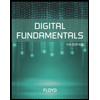
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
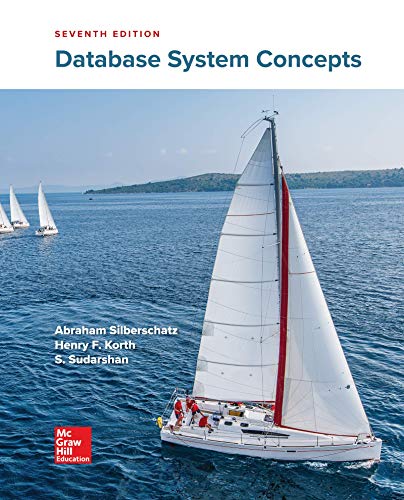
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
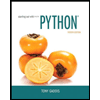
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
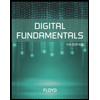
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
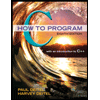
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
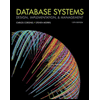
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
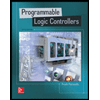
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education