What am I doing wrong on this and how can I fix it? #include #include using namespace std; //declare Student Class class Student { //private member of class private: string Full_Name; float Student_GPA; int Student_Rank; //public class members public: //function to get student data void setStudentData(string studentName,float studentGpa,int studentRank) { this->Full_Name = studentName; this->Student_GPA = studentGpa; this->Student_Rank = studentRank; } //return student data function string getStudentData(string &studentName, float &studentGpa, int &StudentRank) { return Full_Name; return Student_GPA; return Student_Rank; } }; int main() { int Number_Student; //prompt user to enter total number of student cout << "Please input the total students: "; cin >> Number_Student; Student Info[Number_Student]; //variable declaration string studentName[20]; float studentGpa; int studentRank; //prompt user to enter name GPA and rank for (int i = 0; i < Number_Student; i++) { cout << "\nPlease enter the information for the student " << i + 1; cout << "\nStudent Name: "; cin.get(); getline(cin >> ws, studentName[i]); cout << "GPA: "; cin >> studentGpa; cout << "Student Rank(Year): "; cin >> studentRank; Info[i].setStudentData(studentName, studentGpa, studentRank); } //Displays the Winner of the Scholarship contest cout << "\nThe winner of the scholarship contest is: "; float highest = -1; int index = -1; for (int i = 0; i < 3; i++) { info[i].getStudentData(studentName, studentGpa, studentRank); if (highest < studentGpa) { index = i; highest = studentGpa; } } Info[index].getStudentData(studentName, studentGpa, studentRank); cout << "\nThe winner of the scholarship contest is: " << endl; cout << "Name: " << studentName << endl; cout << "GPA: " << studentGpa << endl; cout << "Rank: " << studentRank << endl; cout << "Congratulations " << studentName << "!\n" << endl; cout << "You will get your scholarship funds deposited in your student account"; system("pause"); return 0; }
What am I doing wrong on this and how can I fix it?
#include <iostream>
#include <string>
using namespace std;
//declare Student Class
class Student
{
//private member of class
private:
string Full_Name;
float Student_GPA;
int Student_Rank;
//public class members
public:
//function to get student data
void setStudentData(string studentName,float studentGpa,int studentRank)
{
this->Full_Name = studentName;
this->Student_GPA = studentGpa;
this->Student_Rank = studentRank;
}
//return student data function
string getStudentData(string &studentName, float &studentGpa, int &StudentRank)
{
return Full_Name;
return Student_GPA;
return Student_Rank;
}
};
int main()
{
int Number_Student;
//prompt user to enter total number of student
cout << "Please input the total students: ";
cin >> Number_Student;
Student Info[Number_Student];
//variable declaration
string studentName[20];
float studentGpa;
int studentRank;
//prompt user to enter name GPA and rank
for (int i = 0; i < Number_Student; i++)
{
cout << "\nPlease enter the information for the student " << i + 1;
cout << "\nStudent Name: ";
cin.get();
getline(cin >> ws, studentName[i]);
cout << "GPA: ";
cin >> studentGpa;
cout << "Student Rank(Year): ";
cin >> studentRank;
Info[i].setStudentData(studentName, studentGpa, studentRank);
}
//Displays the Winner of the Scholarship contest
cout << "\nThe winner of the scholarship contest is: ";
float highest = -1;
int index = -1;
for (int i = 0; i < 3; i++)
{
info[i].getStudentData(studentName, studentGpa, studentRank);
if (highest < studentGpa)
{
index = i;
highest = studentGpa;
}
}
Info[index].getStudentData(studentName, studentGpa, studentRank);
cout << "\nThe winner of the scholarship contest is: " << endl;
cout << "Name: " << studentName << endl;
cout << "GPA: " << studentGpa << endl;
cout << "Rank: " << studentRank << endl;
cout << "Congratulations " << studentName << "!\n" << endl;
cout << "You will get your scholarship funds deposited in your student account";
system("pause");
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

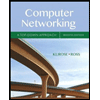
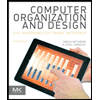
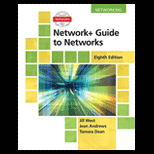
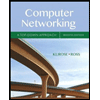
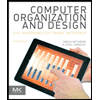
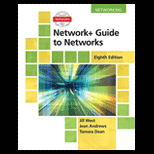
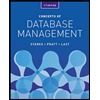
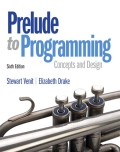
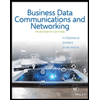