C++ Please submit just one file for the classes and main to test the classes. Just create the classes before main and submit only one cpp file. Do not create separate header files. See Screenshoot I am getting serveral errors :( //Chapter 15 Class Inheritance #include #include #include using namespace std; // Function Prototype void display(TeamLeader); void setName(string n); //double getMon_Bonus(TeamLeader:: mon_Bonus); // Define Empolyee Class class Employee { // Decalre variables public: string empName; string empNumber; string empHireDate; public: Employee() { empName = ""; empNumber = ""; empHireDate = ""; } //Constructor Employee(string empName, string empNumber, string empDate) { //initalize the variables empName = empName; empNumber = empNumber; empHireDate = empHireDate; } // set mutator void setName(string n) { empName = n; } void setNumber(string number) { empName = number; } void setHireDate(string d) { empName = d; } //Accessor string getName() const { return empName; } string getempNumber() { return empNumber; } string getHireDate() const { return empHireDate; } }; // Dervied class Production Worker class ProductionWorker : public Employee { // Declare variables private: int shift; double hourlyPay; public: ProductionWorker():Employee() { shift = 0; hourlyPay = 0.0; } // Constructor ProductionWorker(string en = "", string n = "", string hd = "", int empShift = 0, double empPayRate = 0.0) { //initalize the variables en = empName; n = empNumber; hd = empHireDate; shift = empShift; empPayRate = hourlyPay; } //Mutators to SET the shift void setShift(int s) { shift = s; } void setRate (double r) { hourlyPay = r; } //Accessor to GEt the shift number int getShiftNum() const { return shift; } //Get Shift Name string getShiftName() const { //Return 1 as Day Shift if (shift == 1) return "Day"; //Return 2 as Night shift else if (shift == 2) return "Night"; else return "invaild"; } // to GET the rate double getRate() const { return hourlyPay; } }; // Derived class from ProductionWorker class TeamLeader : public ProductionWorker { //access specifiers and declare variables private: double mon_Bonus;// Monthly Bonus double req_training;//Required training hours double comp_training;//Compeleted training hours public: TeamLeader() :ProductionWorker() { mon_Bouns = 0.0; req_training = 0.0; comp_training = 0.0; } TeamLeader(string empname, string empNumber, string empDate, int empShift, double empPayRate, double empBonus, double empReqTraining, double empCompTraining) :ProductionWorker(empName, empNumber, empDate, empShift, empPayRate) { mon_Bonus = empBonus; req_training = empReqTraining; comp_training = empCompTraining; } //Mutators to set the monthly bonus void setmon_Bonus (double bonus) { mon_Bonus = bonus; } void setReq_raining(double req_tn) { req_training = req_tn; } void setComp_Training(double comp_tn) { comp_training = comp_tn; } //Accessoer to get the monthly bonus double getMon_Bonus() const { return mon_Bonus; } double getComp_Training() const { return comp_training; } double getReq_Training() const { return req_training; } }; int main() { //Create TeamLeader object TeamLeader leader(" Sam Anderson ", " 145 ", " 10/22/2014 ", 1, 14.00, 700.0, 25.0, 16.5); //Call the fuction display(leader); system("pause") return 0; } //Function defintion to display Team Leaders info void display(TeamLeader emp) { cout << setprecision(2) << fixed << showpoint; //Display name cout << "Name: " << emp.getName() << endl; // Display the employee number cout << "HireDate " << emp.getHireDate() << endl; //Display Hire Date cout << "Employee Number: " << emp.getempNumber << endl; //Display shift cout << "Shift: " << emp.getShiftName() << endl; //Display Shift Number cout << "Shift number: " << emp.getShiftNum() << endl; //Display Pay Rate cout << "Pay Rate: " << emp.getRate() << endl; //Dispaly Maonthly Bonus cout << "Monthly bonus:$ " << emp.getmon_bonus() << endl; cout << setpercision(1); //display Emplotee required training cout << "Required Training houres: " << emp.getreq_Training() << endl; //display completed traing hours cout<< "Completed Training Hours: " <
C++
Please submit just one file for the classes and main to test the classes. Just create the classes before main and submit only one cpp file. Do not create separate header files.
See Screenshoot
I am getting serveral errors :(
//Chapter 15 Class Inheritance
#include <iostream>
#include <string>
#include <iomanip>
using namespace std;
// Function Prototype
void display(TeamLeader);
void setName(string n);
//double getMon_Bonus(TeamLeader:: mon_Bonus);
// Define Empolyee Class
class Employee
{
// Decalre variables
public:
string empName;
string empNumber;
string empHireDate;
public:
Employee()
{
empName = "";
empNumber = "";
empHireDate = "";
}
//Constructor
Employee(string empName, string empNumber, string empDate)
{
//initalize the variables
empName = empName;
empNumber = empNumber;
empHireDate = empHireDate;
}
// set mutator
void setName(string n)
{
empName = n;
}
void setNumber(string number)
{
empName = number;
}
void setHireDate(string d)
{
empName = d;
}
//Accessor
string getName() const
{
return empName;
}
string getempNumber()
{
return empNumber;
}
string getHireDate() const
{
return empHireDate;
}
};
// Dervied class Production Worker
class ProductionWorker : public Employee
{
// Declare variables
private:
int shift;
double hourlyPay;
public:
ProductionWorker():Employee()
{
shift = 0;
hourlyPay = 0.0;
}
// Constructor
ProductionWorker(string en = "", string n = "", string hd = "", int empShift = 0, double empPayRate = 0.0)
{ //initalize the variables
en = empName;
n = empNumber;
hd = empHireDate;
shift = empShift;
empPayRate = hourlyPay;
}
//Mutators to SET the shift
void setShift(int s)
{
shift = s;
}
void setRate (double r)
{
hourlyPay = r;
}
//Accessor to GEt the shift number
int getShiftNum() const
{
return shift;
}
//Get Shift Name
string getShiftName() const
{
//Return 1 as Day Shift
if (shift == 1)
return "Day";
//Return 2 as Night shift
else if (shift == 2)
return "Night";
else
return "invaild";
}
// to GET the rate
double getRate() const
{
return hourlyPay;
}
};
// Derived class from ProductionWorker
class TeamLeader : public ProductionWorker
{
//access specifiers and declare variables
private:
double mon_Bonus;// Monthly Bonus
double req_training;//Required training hours
double comp_training;//Compeleted training hours
public:
TeamLeader() :ProductionWorker()
{
mon_Bouns = 0.0;
req_training = 0.0;
comp_training = 0.0;
}
TeamLeader(string empname, string empNumber, string empDate, int empShift,
double empPayRate, double empBonus, double empReqTraining, double empCompTraining)
:ProductionWorker(empName, empNumber, empDate, empShift, empPayRate)
{
mon_Bonus = empBonus;
req_training = empReqTraining;
comp_training = empCompTraining;
}
//Mutators to set the monthly bonus
void setmon_Bonus (double bonus)
{
mon_Bonus = bonus;
}
void setReq_raining(double req_tn)
{
req_training = req_tn;
}
void setComp_Training(double comp_tn)
{
comp_training = comp_tn;
}
//Accessoer to get the monthly bonus
double getMon_Bonus() const
{
return mon_Bonus;
}
double getComp_Training() const
{
return comp_training;
}
double getReq_Training() const
{
return req_training;
}
};
int main()
{
//Create TeamLeader object
TeamLeader leader(" Sam Anderson ", " 145 ", " 10/22/2014 ", 1, 14.00, 700.0, 25.0, 16.5);
//Call the fuction
display(leader);
system("pause")
return 0;
}
//Function defintion to display Team Leaders info
void display(TeamLeader emp)
{
cout << setprecision(2) << fixed << showpoint;
//Display name
cout << "Name: " << emp.getName() << endl;
// Display the employee number
cout << "HireDate " << emp.getHireDate() << endl;
//Display Hire Date
cout << "Employee Number: " << emp.getempNumber << endl;
//Display shift
cout << "Shift: " << emp.getShiftName() << endl;
//Display Shift Number
cout << "Shift number: " << emp.getShiftNum() << endl;
//Display Pay Rate
cout << "Pay Rate: " << emp.getRate() << endl;
//Dispaly Maonthly Bonus
cout << "Monthly bonus:$ " << emp.getmon_bonus() << endl;
cout << setpercision(1);
//display Emplotee required training
cout << "Required Training houres: " << emp.getreq_Training() << endl;
//display completed traing hours
cout<< "Completed Training Hours: " <<emp.getcomp_Training()<<endl:
}


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

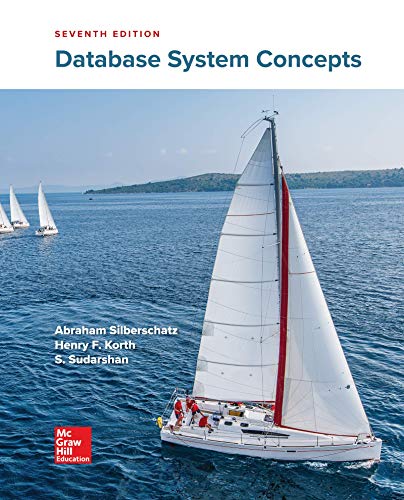
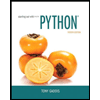
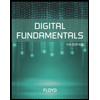
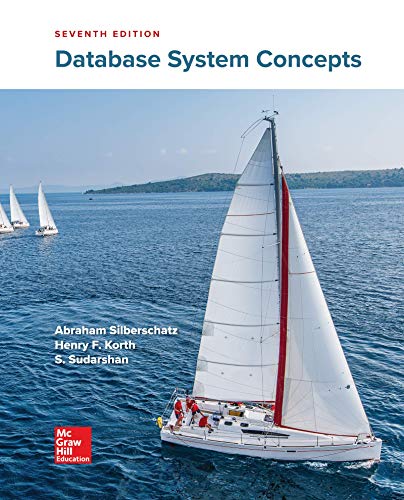
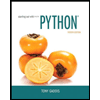
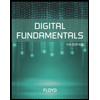
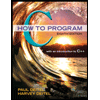
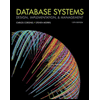
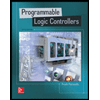