I'm trying to make this program that will output the values of my function display under the subclass items (Circle, Square, Cube) into a file, but when I have a function (display) with multiple lines of return/ print statements the file will show "None" in place of any of the data. class Shape: def __init__(self): self.__color = "red" def set_color(self, color): self.__color = color def get_color(self): return self.__color def find_area(self): pass def find_volume(self): pass def display(self): print(self.__color) class Circle(Shape): def __init__(self): super().__init__() self.__radius = 1 def set_radius(self, radius): self.__radius = radius def get_radius(self): return self.__radius def find_area(self): return 3.14 * self.__radius ** 2 def display(self): print("Type: Circle") super().display() print("Area:", self.find_area()) class Square(Shape): def __init__(self): super().__init__() self.__side = 2.3 def set_side(self, side): self.__side = side def get_side(self): return self.__side def find_area(self): return self.__side ** 2 def display(self): print("Type: Square") super().display() print("Area:", self.find_area()) class Cube(Shape): def __init__(self): super().__init__() self.__length = 2 self.__width = 2 self.__height = 2 def set_length(self, length): self.__length = length def set_width(self, width): self.__width = width def set_height(self, height): self.__height = height def get_length(self): return self.__length def get_width(self): return self.__width def get_height(self): return self.__height def find_volume(self): return self.__length * self.__width * self.__height def display(self): print("Type: Cube") super().display() print("Volume:", self.find_volume()) circles = 0 squares = 0 cubes = 0 shapes = [] for i in range(15): rand = random.randint(1, 3) if rand == 1: shapes.append(Circle()) elif rand == 2: shapes.append(Square()) else: shapes.append(Cube()) for shape in shapes: if isinstance(shape, Circle): circles += 1 elif isinstance(shape, Square): squares += 1 else: cubes += 1 print() # Prompts user for file name file_name = input("Enter file name: ") with open((file_name + ".txt"), "w") as f: # Writes results to text file for shape in shapes: if isinstance(shape,Circle): shape_name='Circle' if isinstance(shape,Square): shape_name='Square' else: shape_name='Cube' f.write('{}, Display{}, Area: {:.2f}\n'.format(shape_name, shape.display(),shape.find_area())) f.write("Results:\n") f.write("Circles: {}\n".format(circles)) f.write("Squares: {}\n".format(squares)) f.write("Cubes: {}\n".format(cubes))
I'm trying to make this program that will output the values of my function display under the subclass items (Circle, Square, Cube) into a file, but when I have a function (display) with multiple lines of return/ print statements the file will show "None" in place of any of the data.
class Shape:
def __init__(self):
self.__color = "red"
def set_color(self, color):
self.__color = color
def get_color(self):
return self.__color
def find_area(self):
pass
def find_volume(self):
pass
def display(self):
print(self.__color)
class Circle(Shape):
def __init__(self):
super().__init__()
self.__radius = 1
def set_radius(self, radius):
self.__radius = radius
def get_radius(self):
return self.__radius
def find_area(self):
return 3.14 * self.__radius ** 2
def display(self):
print("Type: Circle")
super().display()
print("Area:", self.find_area())
class Square(Shape):
def __init__(self):
super().__init__()
self.__side = 2.3
def set_side(self, side):
self.__side = side
def get_side(self):
return self.__side
def find_area(self):
return self.__side ** 2
def display(self):
print("Type: Square")
super().display()
print("Area:", self.find_area())
class Cube(Shape):
def __init__(self):
super().__init__()
self.__length = 2
self.__width = 2
self.__height = 2
def set_length(self, length):
self.__length = length
def set_width(self, width):
self.__width = width
def set_height(self, height):
self.__height = height
def get_length(self):
return self.__length
def get_width(self):
return self.__width
def get_height(self):
return self.__height
def find_volume(self):
return self.__length * self.__width * self.__height
def display(self):
print("Type: Cube")
super().display()
print("Volume:", self.find_volume())
circles = 0
squares = 0
cubes = 0
shapes = []
for i in range(15):
rand = random.randint(1, 3)
if rand == 1:
shapes.append(Circle())
elif rand == 2:
shapes.append(Square())
else:
shapes.append(Cube())
for shape in shapes:
if isinstance(shape, Circle):
circles += 1
elif isinstance(shape, Square):
squares += 1
else:
cubes += 1
print()
# Prompts user for file name
file_name = input("Enter file name: ")
with open((file_name + ".txt"), "w") as f:
# Writes results to text file
for shape in shapes:
if isinstance(shape,Circle):
shape_name='Circle'
if isinstance(shape,Square):
shape_name='Square'
else:
shape_name='Cube'
f.write('{}, Display{}, Area: {:.2f}\n'.format(shape_name, shape.display(),shape.find_area()))
f.write("Results:\n")
f.write("Circles: {}\n".format(circles))
f.write("Squares: {}\n".format(squares))
f.write("Cubes: {}\n".format(cubes))


Step by step
Solved in 2 steps with 1 images

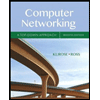
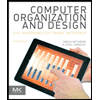
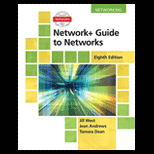
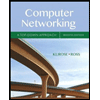
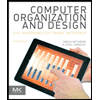
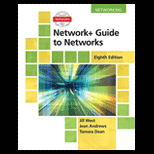
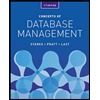
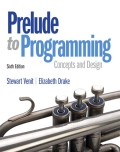
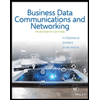