Please solve in C++ /* * CECS 2223, Computer Programming II Laboratory * Winter 2021, Sec. 05 * Date: November 24, 2021 * Topic: OOD Review * File name: Box.cpp * This file defines the Box class * You are required to define all methods declared in Box.h * Name: [YOUR NAME HERE], ID#[YOUR ID NUMBER HERE] */ // write the include statements required // The get and set methods DO NOT prompt the user for values // or include any cout statements // The getSurfaceArea methods computes the surface area of the box, // that is the sum of the areas of each side. // The printBox method is to output values formatted // to be displayed in a table, with 10 spaces per column. // The values to be displayed are: // height, width, length, area, and volume. // All values must be printed in the same line. Solve in C++ Please /* * CECS 2223, Computer Programming II Laboratory * Winter 2021, Sec. 05 * Date: November 24, 2021 * Topic: OOD Review * File name: Box.h * This file declares a class named Box * Name: [YOUR NAME HERE], ID#[YOUR ID NUMBER HERE] */ // This file contains errors, find and correct them! // write the include statements required // write the using declaration class Box{ private: int height = 0; int width; int length; public: Box(); void setHeight(int); void setWidth(int); void setLength(int); int getHeight(); int getWidth(); int getLength(); int getSurfaceArea(); int getVolume(); void printBox(); } /* * CECS 2223, Computer Programming II Laboratory * Winter 2021, Sec. 05 * Date: November 24, 2021 * Topic: OOD Review * File name: Box.cpp * This file defines the Box class * You are required to define all methods declared in Box.h * Name: [YOUR NAME HERE], ID#[YOUR ID NUMBER HERE] */ // write the include statements required // The get and set methods DO NOT prompt the user for values // or include any cout statements // The getSurfaceArea methods computes the surface area of the box, // that is the sum of the areas of each side. // The printBox method is to output values formatted // to be displayed in a table, with 10 spaces per column. // The values to be displayed are: // height, width, length, area, and volume. // All values must be printed in the same line. /* * CECS 2223, Computer Programming II Laboratory * Winter 2021, Sec. 05 * Date: November 24, 2021 * Topic: OOD Review * File name: lab01.cpp * This file implements the Box class * Follow the instructions and completed the C++ code. * Name: [YOUR NAME HERE], ID#[YOUR ID NUMBER HERE] */ // write the required include statement // the main function returns an integer value, should be 0 int main(){ // declare a Box object, named box1, use the default constructor // assign a height of 5, width of 10 and a length of 20 to box1 // declare another Box object, named box2, whose height is 7, // width is 5, and length is 10 // declare a Box object, named box3, whose height is 3, width is 4, // and length is 12 // declare a Box object, named box4, whose height is 20, width is 10, // and length is 5 cout << "The boxes created have the following dimensions:\n"; // table headers print a header line in which each column has a width of 10 // The column headers are:"HEIGHT","WIDTH","LENGTH","AREA","VOLUME" // using printBox method, print the dimensions for each box object // write a statement which prints the phrase // "Program developed by [YOUR NAME], ID#[YOUR ID NUMBER]" // where the square brackets and the text within is substitued with // your personal information. system("pause"); // for Visual Studio use only return 0; // this statement MUST be included at the end of main }
Please solve in C++ /* * CECS 2223, Computer Programming II Laboratory * Winter 2021, Sec. 05 * Date: November 24, 2021 * Topic: OOD Review * File name: Box.cpp * This file defines the Box class * You are required to define all methods declared in Box.h * Name: [YOUR NAME HERE], ID#[YOUR ID NUMBER HERE] */ // write the include statements required // The get and set methods DO NOT prompt the user for values // or include any cout statements // The getSurfaceArea methods computes the surface area of the box, // that is the sum of the areas of each side. // The printBox method is to output values formatted // to be displayed in a table, with 10 spaces per column. // The values to be displayed are: // height, width, length, area, and volume. // All values must be printed in the same line. Solve in C++ Please /* * CECS 2223, Computer Programming II Laboratory * Winter 2021, Sec. 05 * Date: November 24, 2021 * Topic: OOD Review * File name: Box.h * This file declares a class named Box * Name: [YOUR NAME HERE], ID#[YOUR ID NUMBER HERE] */ // This file contains errors, find and correct them! // write the include statements required // write the using declaration class Box{ private: int height = 0; int width; int length; public: Box(); void setHeight(int); void setWidth(int); void setLength(int); int getHeight(); int getWidth(); int getLength(); int getSurfaceArea(); int getVolume(); void printBox(); } /* * CECS 2223, Computer Programming II Laboratory * Winter 2021, Sec. 05 * Date: November 24, 2021 * Topic: OOD Review * File name: Box.cpp * This file defines the Box class * You are required to define all methods declared in Box.h * Name: [YOUR NAME HERE], ID#[YOUR ID NUMBER HERE] */ // write the include statements required // The get and set methods DO NOT prompt the user for values // or include any cout statements // The getSurfaceArea methods computes the surface area of the box, // that is the sum of the areas of each side. // The printBox method is to output values formatted // to be displayed in a table, with 10 spaces per column. // The values to be displayed are: // height, width, length, area, and volume. // All values must be printed in the same line. /* * CECS 2223, Computer Programming II Laboratory * Winter 2021, Sec. 05 * Date: November 24, 2021 * Topic: OOD Review * File name: lab01.cpp * This file implements the Box class * Follow the instructions and completed the C++ code. * Name: [YOUR NAME HERE], ID#[YOUR ID NUMBER HERE] */ // write the required include statement // the main function returns an integer value, should be 0 int main(){ // declare a Box object, named box1, use the default constructor // assign a height of 5, width of 10 and a length of 20 to box1 // declare another Box object, named box2, whose height is 7, // width is 5, and length is 10 // declare a Box object, named box3, whose height is 3, width is 4, // and length is 12 // declare a Box object, named box4, whose height is 20, width is 10, // and length is 5 cout << "The boxes created have the following dimensions:\n"; // table headers print a header line in which each column has a width of 10 // The column headers are:"HEIGHT","WIDTH","LENGTH","AREA","VOLUME" // using printBox method, print the dimensions for each box object // write a statement which prints the phrase // "Program developed by [YOUR NAME], ID#[YOUR ID NUMBER]" // where the square brackets and the text within is substitued with // your personal information. system("pause"); // for Visual Studio use only return 0; // this statement MUST be included at the end of main }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Please solve in C++
/*
* CECS 2223, Computer Programming II Laboratory
* Winter 2021, Sec. 05
* Date: November 24, 2021
* Topic: OOD Review
* File name: Box.cpp
* This file defines the Box class
* You are required to define all methods declared in Box.h
* Name: [YOUR NAME HERE], ID#[YOUR ID NUMBER HERE]
*/
// write the include statements required
// The get and set methods DO NOT prompt the user for values
// or include any cout statements
// The getSurfaceArea methods computes the surface area of the box,
// that is the sum of the areas of each side.
// The printBox method is to output values formatted
// to be displayed in a table, with 10 spaces per column.
// The values to be displayed are:
// height, width, length, area, and volume.
// All values must be printed in the same line.
Solve in C++ Please
/*
* CECS 2223, Computer Programming II Laboratory
* Winter 2021, Sec. 05
* Date: November 24, 2021
* Topic: OOD Review
* File name: Box.h
* This file declares a class named Box
* Name: [YOUR NAME HERE], ID#[YOUR ID NUMBER HERE]
*/
// This file contains errors, find and correct them!
// write the include statements required
// write the using declaration
class Box{
private:
int height = 0;
int width;
int length;
public:
Box();
void setHeight(int);
void setWidth(int);
void setLength(int);
int getHeight();
int getWidth();
int getLength();
int getSurfaceArea();
int getVolume();
void printBox();
}
/*
* CECS 2223, Computer Programming II Laboratory
* Winter 2021, Sec. 05
* Date: November 24, 2021
* Topic: OOD Review
* File name: Box.cpp
* This file defines the Box class
* You are required to define all methods declared in Box.h
* Name: [YOUR NAME HERE], ID#[YOUR ID NUMBER HERE]
*/
// write the include statements required
// The get and set methods DO NOT prompt the user for values
// or include any cout statements
// The getSurfaceArea methods computes the surface area of the box,
// that is the sum of the areas of each side.
// The printBox method is to output values formatted
// to be displayed in a table, with 10 spaces per column.
// The values to be displayed are:
// height, width, length, area, and volume.
// All values must be printed in the same line.
/*
* CECS 2223, Computer Programming II Laboratory
* Winter 2021, Sec. 05
* Date: November 24, 2021
* Topic: OOD Review
* File name: lab01.cpp
* This file implements the Box class
* Follow the instructions and completed the C++ code.
* Name: [YOUR NAME HERE], ID#[YOUR ID NUMBER HERE]
*/
// write the required include statement
// the main function returns an integer value, should be 0
int main(){
// declare a Box object, named box1, use the default constructor
// assign a height of 5, width of 10 and a length of 20 to box1
// declare another Box object, named box2, whose height is 7,
// width is 5, and length is 10
// declare a Box object, named box3, whose height is 3, width is 4,
// and length is 12
// declare a Box object, named box4, whose height is 20, width is 10,
// and length is 5
cout << "The boxes created have the following dimensions:\n";
// table headers print a header line in which each column has a width of 10
// The column headers are:"HEIGHT","WIDTH","LENGTH","AREA","VOLUME"
// using printBox method, print the dimensions for each box object
// write a statement which prints the phrase
// "Program developed by [YOUR NAME], ID#[YOUR ID NUMBER]"
// where the square brackets and the text within is substitued with
// your personal information.
system("pause"); // for Visual Studio use only
return 0; // this statement MUST be included at the end of main
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
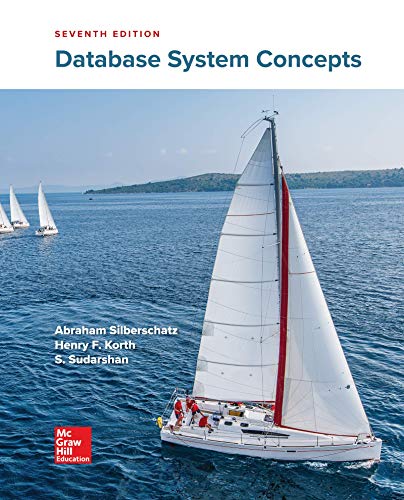
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
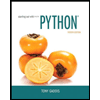
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
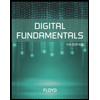
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
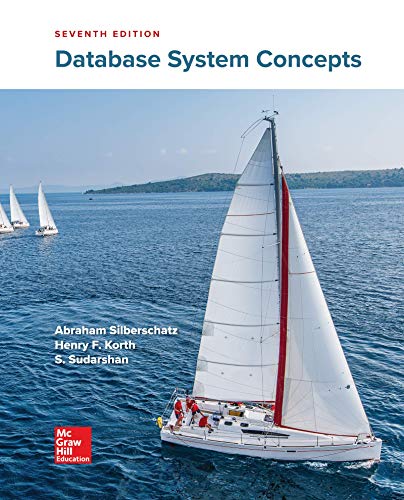
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
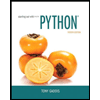
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
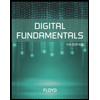
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
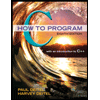
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
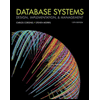
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
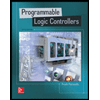
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education