Bank Program This c++ assignment uses the same account class created in Lab #4 with a more generally useful interface. It reads commands from a bank.txt file. Your program must process the following four commands. Create account amount Deposit account amount Withdraw account amount Balance account In all of the above commands, account is an integer and amount is a double. The following program behavior is required – 1. Valid account numbers are 1-9. This requires the program to have an array of references to Account objects. 2. If the first word on any line contains a command other than the four listed above, an error message should be displayed and that line ignored. 3. The create command creates a new account object with the given account number and initial balance. If an account already exists with that number an error message should be displayed and the command ignored. 4. The Deposit and Withdraw commands perform the indicated operation on an existing account. If no account with that number has been created, an error message is displayed and the command ignored. 5. The Balance command displays the balance of the requested account. No change to the account occurs. If no account with that number has been created, an error message is displayed and the command ignored. Previous account class used: #ifndef Account_h #define Account_h using namespace std; class Account { private: int id; double balance; public: //default constructor Account() { id = 0; balance = 0; } //overload constructor Account(int newID, double initialBalance) { this->id = newID; this->balance = initialBalance; } //sets id void setId(int newID) { this->id = newID; } //returns id int getId() { return this->id; } //sets balance void setBalance(double newBalance) { this->balance = newBalance; } //returns balance double getBalance() { return this->balance; } //withdraws from balance void withdraw(double amount) { this->balance = this->balance - amount; } //adds to balance void deposit(double amount) { this->balance = this->balance + amount; } }; #endif ------ bank.txt: Create 1 1000.01 Create 2 2000.02 Create 3 3000.03 Deposit 1 11.11 Deposit 2 22.22 Withdraw 4 5000.00 Create 4 4000.04 Withdraw 1 0.10 Balance 2 Withdraw 2 0.20 Deposit 3 33.33 Withdraw 4 0.40 Bad Command 65 Balance 1 Balance 2 Balance 3 Balance 4 -------- Sample output: put in as a jpg:
Bank Program
This c++ assignment uses the same account class created in Lab #4 with a more generally useful interface. It reads commands from a bank.txt file. Your program must process the following four commands.
Create account amount
Deposit account amount
Withdraw account amount
Balance account
In all of the above commands, account is an integer and amount is a double.
The following program behavior is required –
1. Valid account numbers are 1-9. This requires the program to have an array of references to Account objects.
2. If the first word on any line contains a command other than the four listed above, an error message should be displayed and that line ignored.
3. The create command creates a new account object with the given account number and initial balance. If an account already exists with that number an error message should be displayed and the command ignored.
4. The Deposit and Withdraw commands perform the indicated operation on an existing account. If no account with that number has been created, an error message is displayed and the command ignored.
5. The Balance command displays the balance of the requested account. No change to the account occurs. If no account with that number has been created, an error message is displayed and the command ignored.
Previous account class used:
#ifndef Account_h
#define Account_h
using namespace std;
class Account
{
private:
int id;
double balance;
public:
//default constructor
Account()
{
id = 0;
balance = 0;
}
//overload constructor
Account(int newID, double initialBalance)
{
this->id = newID;
this->balance = initialBalance;
}
//sets id
void setId(int newID)
{
this->id = newID;
}
//returns id
int getId()
{
return this->id;
}
//sets balance
void setBalance(double newBalance)
{
this->balance = newBalance;
}
//returns balance
double getBalance()
{
return this->balance;
}
//withdraws from balance
void withdraw(double amount)
{
this->balance = this->balance - amount;
}
//adds to balance
void deposit(double amount)
{
this->balance = this->balance + amount;
}
};
#endif
------
bank.txt:
Create 1 1000.01
Create 2 2000.02
Create 3 3000.03
Deposit 1 11.11
Deposit 2 22.22
Withdraw 4 5000.00
Create 4 4000.04
Withdraw 1 0.10
Balance 2
Withdraw 2 0.20
Deposit 3 33.33
Withdraw 4 0.40
Bad Command 65
Balance 1
Balance 2
Balance 3
Balance 4
--------
Sample output: put in as a jpg:


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

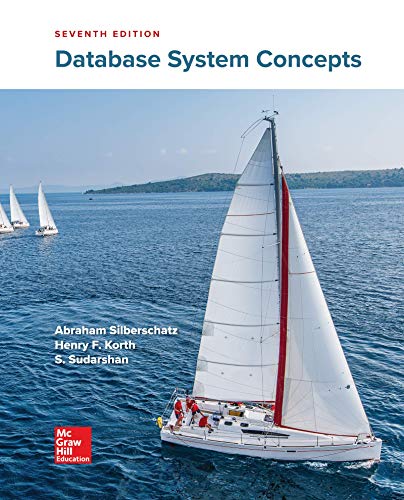
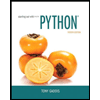
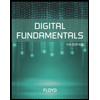
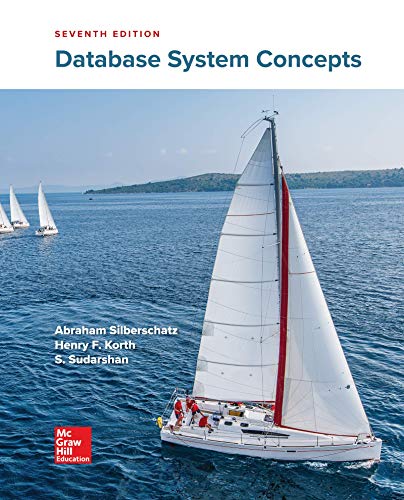
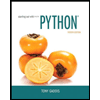
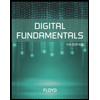
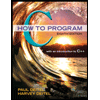
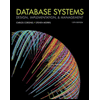
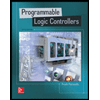