Convert output function of Vehicle to abstract class.
1. Copy the previous program to a new file.
#include <iostream>
using namespace std;
class Vehicle
{
private:
string brand;
public:
Vehicle()
{
this->brand = "TBD";
}
Vehicle(string brand)
{
this->brand = brand;
}
void output()
{
cout << "Brand: " << this->brand << endl;
}
string getBrand() const
{
return brand;
}
void setBrand(const string &brand_)
{
brand = brand_;
}
};
class Car:public Vehicle
{
private:
int *weight; //declaring dynamic variable
public:
Car():Vehicle() //calling Vehicle default constructor
{
weight=new int; //allocate memory
*weight=0; //initialize weight to 0
}
Car(string b, int w):Vehicle(b) //invokes Vehicle parameterized constructor
{
weight=new int; //allocate memory
*weight=w; //sets weight
}
void output()
{
cout << "Brand: " << getBrand() << " Weight: " << *weight << endl; //prints Brand and weight
}
void setWeight(int w)
{
*weight=w; //sets weight
}
int getWeight()
{
return *weight; //returns weight
}
};
class Boat:public Vehicle
{
private:
int *hullLength; //declaring dynamic variable
public:
Boat():Vehicle() //calling Vehicle default constructor
{
hullLength=new int; //allocate memory
*hullLength=0; //initialize hullLength to 0
}
Boat(string b, int h):Vehicle(b) //calling parameterized constructor
{
hullLength=new int; //allocate memory
*hullLength=h; //set hullLength
}
void output()
{
cout << "Brand: " << getBrand() << " hullLength: " << *hullLength << endl; //prints Brand and hullLength
}
void sethullLength(int h)
{
*hullLength=h; //sets hullLength
}
int gethullLength()
{
return *hullLength; //returns hullLength
}
};
int main()
{
Car a,b("Honda",2000);
a.output(); // Brand:TBD Weight: 0
b.output(); // Brand:Honda Weight: 2000
b.setBrand("Tesla");
b.setWeight(3000);
b.output(); // Brand:Tesla Weight: 3000
Boat c,d("Baja",100);
c.output(); // Brand:TBD hullLength: 0
d.output(); // Brand:Baja hullLength: 100
d.setBrand("Bertram");
d.sethullLength(60);
d.output(); // Brand:Bertram hullLength: 60
}
2. Convert output function of Vehicle to abstract class.
3. Override output function of Car and Boat class.
Use following main() to test your function.
int main(){
Vehicle *a;
a = new Car("Honda",2000);
a->output(); // Brand:Honda Weight: 2000
a = new Boat("Baja",100);
a->output(); // Brand:Baja hullLength: 100
}
Output from main:
Brand: Honda Weight: 2000
Brand: Baja hullLength: 100

Step by step
Solved in 3 steps with 1 images

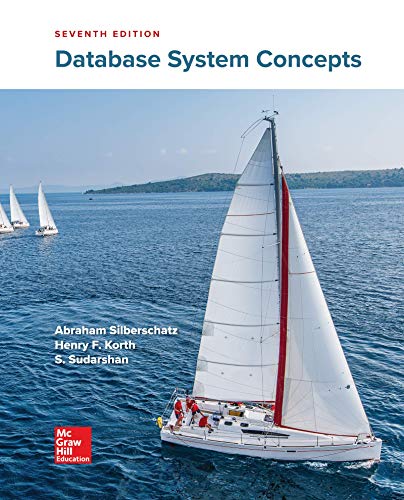
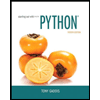
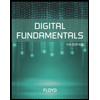
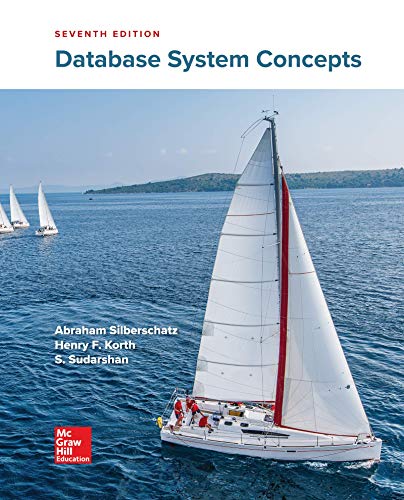
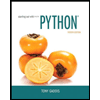
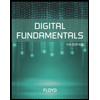
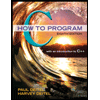
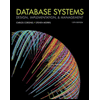
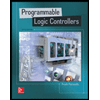