Given main(), build a struct called BankAccount that manages checking and savings accounts. The struct has three data members: a customer name (string), the customer's savings account balance (double), and the customer's checking account balance (double). Assume customer name has a maximum length of 20.
Given main(), build a struct called BankAccount that manages checking and savings accounts. The struct has three data members: a customer name (string), the customer's savings account balance (double), and the customer's checking account balance (double). Assume customer name has a maximum length of 20.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Given main(), build a struct called BankAccount that manages checking and savings accounts. The struct has three data members: a customer name (string), the customer's savings account balance (double), and the customer's checking account balance (double). Assume customer name has a maximum length of 20.
![J
Given main(), build a struct called BankAccount that manages checking and savings accounts. The struct has three data members: a
customer name (string), the customer's savings account balance (double), and the customer's checking account balance (double). Assume
customer name has a maximum length of 20.
Implement the BankAccount struct and related function declarations in BankAccount.h, and implement the related function definitions in
BankAccount.c as listed below:
BankAccount InitBankAccount(char* new Name, double amt1, double amt2) - set the customer name to parameter newName, set the
checking account balance to parameter amt1 and set the savings account balance to parameter amt2. (amt stands for amount)
• BankAccount SetName(char* newName, BankAccount account) - set the customer name
void GetName(char* customerName, BankAccount account) - return the customer name in customerName
• BankAccount SetChecking(double amt, BankAccount account) - set the checking account balance to parameter amt
• double GetChecking (BankAccount account) - return the checking account balance
• BankAccount SetSavings(double amt, BankAccount account) - set the savings account balance to parameter amt
• double GetSavings(BankAccount account) - return the savings account balance
• BankAccount DepositChecking (double amt, BankAccount account) - add parameter amt to the checking account balance (only if
positive)
• BankAccount DepositSavings(double amt, BankAccount account) - add parameter amt to the savings account balance (only if
positive)
BankAccount WithdrawChecking (double amt, BankAccount account) - subtract parameter amt from the checking account balance
(only if positive)
BankAccount WithdrawSavings(double amt, BankAccount account) - subtract parameter amt from the savings account balance (only
if positive)
• BankAccount Transfer ToSavings(double amt, BankAccount account) - subtract parameter amt from the checking account balance
and add to the savings account balance (only if positive)
Current file: main.c
File is marked as read only
1 #include <stdio.h>
2 #include <string.h>
3
4 #include "BankAccount.h"
5
6 int main() {
BankAccount account = InitBankAccount ("Mickey", 500.00, 1000.00);
char name[20];
6789 N
10 account = SetChecking (500, account);
11 account = SetSavings (500, account);
12 account = WithdrawSavings (100, account);
13 account WithdrawChecking (100, account);
14 account = Transfer ToSavings (300, account);
15
448599272
16 GetName (name, account);
17 printf("%s\n", name);
printf("$%.2f\n", GetChecking (account));
19 printf("$%.2f\n", GetSavings (account));
18
20
21 return 0;
22](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5779ee7a-d811-43a6-ba75-7d41285b789d%2Fe661c6d5-0940-4227-bb86-8d4e7118c420%2Frq9kfy_processed.png&w=3840&q=75)
Transcribed Image Text:J
Given main(), build a struct called BankAccount that manages checking and savings accounts. The struct has three data members: a
customer name (string), the customer's savings account balance (double), and the customer's checking account balance (double). Assume
customer name has a maximum length of 20.
Implement the BankAccount struct and related function declarations in BankAccount.h, and implement the related function definitions in
BankAccount.c as listed below:
BankAccount InitBankAccount(char* new Name, double amt1, double amt2) - set the customer name to parameter newName, set the
checking account balance to parameter amt1 and set the savings account balance to parameter amt2. (amt stands for amount)
• BankAccount SetName(char* newName, BankAccount account) - set the customer name
void GetName(char* customerName, BankAccount account) - return the customer name in customerName
• BankAccount SetChecking(double amt, BankAccount account) - set the checking account balance to parameter amt
• double GetChecking (BankAccount account) - return the checking account balance
• BankAccount SetSavings(double amt, BankAccount account) - set the savings account balance to parameter amt
• double GetSavings(BankAccount account) - return the savings account balance
• BankAccount DepositChecking (double amt, BankAccount account) - add parameter amt to the checking account balance (only if
positive)
• BankAccount DepositSavings(double amt, BankAccount account) - add parameter amt to the savings account balance (only if
positive)
BankAccount WithdrawChecking (double amt, BankAccount account) - subtract parameter amt from the checking account balance
(only if positive)
BankAccount WithdrawSavings(double amt, BankAccount account) - subtract parameter amt from the savings account balance (only
if positive)
• BankAccount Transfer ToSavings(double amt, BankAccount account) - subtract parameter amt from the checking account balance
and add to the savings account balance (only if positive)
Current file: main.c
File is marked as read only
1 #include <stdio.h>
2 #include <string.h>
3
4 #include "BankAccount.h"
5
6 int main() {
BankAccount account = InitBankAccount ("Mickey", 500.00, 1000.00);
char name[20];
6789 N
10 account = SetChecking (500, account);
11 account = SetSavings (500, account);
12 account = WithdrawSavings (100, account);
13 account WithdrawChecking (100, account);
14 account = Transfer ToSavings (300, account);
15
448599272
16 GetName (name, account);
17 printf("%s\n", name);
printf("$%.2f\n", GetChecking (account));
19 printf("$%.2f\n", GetSavings (account));
18
20
21 return 0;
22

Transcribed Image Text:1 /* TODO: Type your header file guards and include directives here. */
2
N346
4 /* Type your code here. */
Current file: BankAccount.h
5
Current file: BankAccount.c
1 /* TODO: Type your header file guards and include directives here. */
2
3
4 /* Type your code here. */
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 6 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
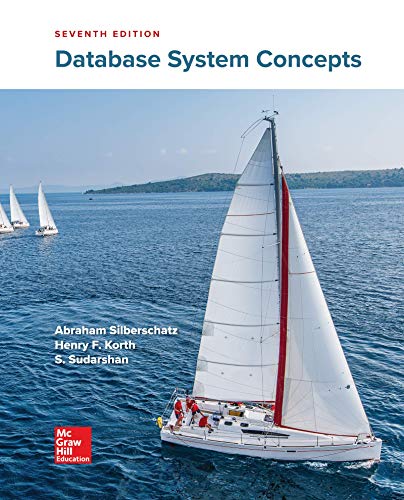
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
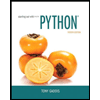
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
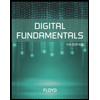
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
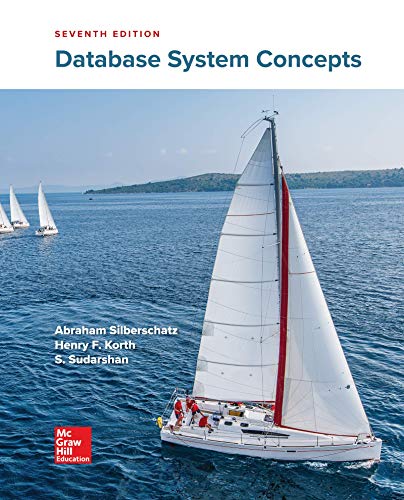
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
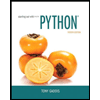
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
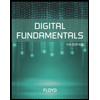
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
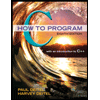
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
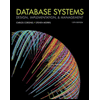
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
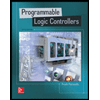
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education