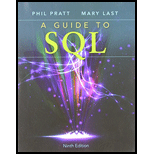
Concept explainers
a.
Stored procedures:
- A procedure is a collection of procedural and SQL statements.
- A procedure may have input parameter or output parameter or sometimes both the parameters.
- It is declared with a unique name with a unit of procedural code using the proprietary RDBMS and it is invoked by a host language library routine.
Syntax for stored procedure:
CREATE FUNCTION fun_name(argument IN data-type)RETRUN data-type[IS]
BEGIN
PL/SQL statements;
Return (value or expression);
END;

Explanation of Solution
Query to create stored procedure:
CREATE OR REPLACE PROCEDURE DISP_OWNER (I_OWNER_NUM IN OWNER.OWNER_NUM%TYPE) AS
I_FIRST_NAME OWNER.FIRST_NAME%TYPE;
I_LAST_NAME OWNER.LAST_NAME%TYPE;
BEGIN
SELECT FIRST_NAME, LAST_NAME
INTO I_FIRST_NAME, I_LAST_NAME
FROM OWNER
WHERE OWNER_NUM = I_OWNER_NUM;
DBMS_OUTPUT.PUT_LINE(I_OWNER_NUM);
DBMS_OUTPUT.PUT_LINE(I_FIRST_NAME);
DBMS_OUTPUT.PUT_LINE(I_LAST_NAME);
END;
/
Explanation:
- The above query is used to create a procedure named “DISP_OWNER” to select the records in the “OWNER” table.
- Change the “OWNER_NUM” into “I_OWNER_NUM” and place the “FIRST_NAME” and “LAST_NAME” values into “I_FIRST_NAME” and “I_LAST_NAME”.
- After placing these values, display the “I_OWNER_NUM”, “I_FIRST_NAME”, and “I_LAST_NAME” from the “OWNER” table.
- Once the stored procedure is created, it needs to be executed.
Query to view the owner name and ID:
BEGIN
DISP_OWNER ('AN175');
END;
The above query is used to view the owner ID, first name, and last name for the number “AN175”.
Output:
AN175
Bill
Anderson
Explanation of Solution
b.
Query to create stored procedure:
CREATE OR REPLACE PROCEDURE DISP_CONDO_UNIT
(I_CONDO_ID IN CONDO_UNIT.CONDO_ID%TYPE) AS
I_LOCATION_NUM CONDO_UNIT.LOCATION_NUM%TYPE;
I_UNIT_NUM CONDO_UNIT.UNIT_NUM%TYPE;
I_OWNER_NUM CONDO_UNIT.OWNER_NUM%TYPE;
I_FIRST_NAME OWNER.FIRST_NAME%TYPE;
I_LAST_NAME OWNER.LAST_NAME%TYPE;
BEGIN
SELECT LOCATION_NUM, UNIT_NUM, CONDO_UNIT.OWNER_NUM, FIRST_NAME, LAST_NAME
INTO I_LOCATION_NUM, I_UNIT_NUM, I_OWNER_NUM, I_FIRST_NAME, I_LAST_NAME
FROM CONDO_UNIT, OWNER
WHERE CONDO_UNIT.OWNER_NUM = OWNER.OWNER_NUM
AND CONDO_ID = I_CONDO_ID;
DBMS_OUTPUT.PUT_LINE(I_CONDO_ID);
DBMS_OUTPUT.PUT_LINE(I_LOCATION_NUM);
DBMS_OUTPUT.PUT_LINE(I_UNIT_NUM);
DBMS_OUTPUT.PUT_LINE(I_OWNER_NUM);
DBMS_OUTPUT.PUT_LINE(I_FIRST_NAME);
DBMS_OUTPUT.PUT_LINE(I_LAST_NAME);
END;
/
Explanation:
- The above query is used to create a procedure named “DISP_CONDO_UNIT” to select the records in the “CONDO_UNIT” and “OWNER” tables.
- Change the “CONDO_ID” into “I_CONDO_ID” and place the “LOCATION_NUM”, “UNIT_NUM”, “OWNER_NUM”, “FIRST_NAME”, and “LAST_NAME” values into “I_LOCATION_NUM”, “I_UNIT_NUM”, “I_OWNER_NUM”, “I_FIRST_NAME”, and “I_ LAST_NAME”.
- After placing these values, display the “I_CONDO_ID”, I_LOCATION_NUM”, “I_UNIT_NUM”, “I_OWNER_NUM”, “I_FIRST_NAME”, and “I_ LAST_NAME” from the “CONDO_UNIT” and “OWNER” tables.
- Once the stored procedure is created, it needs to be executed.
Query to view a result:
BEGIN
DISP_CONDO_UNIT(3);
END;
The above query is used to view the condo Id, location number, unit number, owner number, first name and last name for the condo id “3”.

Output:
3
1
306
AN175
Bill
Anderson
Explanation of Solution
c.
Query to insert the value:
CREATE OR REPLACE PROCEDURE ADD_OWNER
(I_OWNER_NUM IN OWNER.OWNER_NUM%TYPE,
I_LAST_NAME IN OWNER.LAST_NAME%TYPE,
I_FIRST_NAME IN OWNER.FIRST_NAME%TYPE,
I_ADDRESS IN OWNER.ADDRESS%TYPE,
I_CITY IN OWNER.CITY%TYPE,
I_STATE IN OWNER.STATE%TYPE,
I_POSTAL_CODE IN OWNER.POSTAL_CODE%TYPE) AS
BEGIN
INSERT INTO OWNER (OWNER_NUM, LAST_NAME, FIRST_NAME, ADDRESS, CITY, STATE, POSTAL_CODE)
VALUES
(I_OWNER_NUM, I_LAST_NAME, I_FIRST_NAME, I_ADDRESS, I_CITY, I_STATE,
I_POSTAL_CODE);
END;
/
Explanation:
The above query is used to create a stored procedure named “ADD_OWNER” to insert the new record in the “OWNER” table. Once the stored procedure is created, it needs to be executed.
Query to execute the stored procedure:
BEGIN
ADD_OWNER ('AZ245', 'William', 'John', '125 North St.', 'Mills', 'SC', 29707);
END;
After executing the above query, the new record is inserted into the table “OWNER”.

Output:
Query to view the contents in “OWNER” table is as follows:
SELECT * FROM OWNER;
Screenshot of output
Explanation of Solution
d.
Query to update stored procedure:
CREATE OR REPLACE PROCEDURE CHG_OWNER
(I_OWNER_NUM IN OWNER.OWNER_NUM%TYPE,
I_LAST_NAME IN OWNER.LAST_NAME%TYPE) AS
BEGIN
UPDATE OWNER
SET LAST_NAME = I_LAST_NAME
WHERE OWNER_NUM = I_OWNER_NUM;
END;
/
Explanation:
The above query is used to create a stored procedure named “CHG_OWNER” to update the last name of the owner whose number is stored in “I_OWNER_NUM” to the value presently found in “I_OWNER_NUM”, it needs to be executed.
Query to execute the stored procedure:
BEGIN
CHG_OWNER ('AZ245', 'Andrew');
END;
After executing the above query, the last name is changed in the table “OWNER”.

Output:
Query to view the contents in “OWNER” table is as follows:
SELECT * FROM OWNER;
Screenshot of output
Explanation of Solution
e.
Query to delete the value:
CREATE OR REPLACE PROCEDURE DELETE_OWNER
(I_OWNER_NUM IN OWNER.OWNER_NUM%TYPE) AS
BEGIN
DELETE
FROM OWNER
WHERE OWNER_NUM = I_OWNER_NUM;
END;
/
Explanation:
- The above query is used to create a procedure named “DELETE_OWNER” to delete a record in the “OWNER” table.
- Once the record is deleted, a procedure should create owner number as a parameter.
- Once the stored procedure is created, it needs to be executed.
Query to execute the stored procedure:
BEGIN
DELETE_OWNER ('AZ245');
END;
The above query is used to delete the order number AZ245.

Output:
Query to view the contents in “OWNER” table after deleting the order number AZ245 as follows:
SELECT * FROM OWNER;
Screenshot of output
Want to see more full solutions like this?
Chapter 8 Solutions
A Guide to SQL
- The following is code for a disc golf program written in C++: // player.h #ifndef PLAYER_H #define PLAYER_H #include <string> #include <iostream> class Player { private: std::string courses[20]; // Array of course names int scores[20]; // Array of scores int gameCount; // Number of games played public: Player(); // Constructor void CheckGame(int playerId, const std::string& courseName, int gameScore); void ReportPlayer(int playerId) const; }; #endif // PLAYER_H // player.cpp #include "player.h" #include <iomanip> Player::Player() : gameCount(0) {} void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) { for (int i = 0; i < gameCount; ++i) { if (courses[i] == courseName) { // If course has been played, then check for minimum score if (gameScore < scores[i]) { scores[i] = gameScore; // Update to new minimum…arrow_forwardIn this assignment, you will implement a multi-threaded program (using C/C++) that will check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome numbers are numbers that their decimal representation can be read from left to right and from right to left (e.g. 12321, 5995, 1234321). The program will create T worker threads to check for prime and palindrome numbers in the given range (T will be passed to the program with the Linux command line). Each of the threads works on a part of the numbers within the range. Your program should have some global shared variables: • numOfPrimes: which will track the total number of prime numbers found by all threads. numOfPalindroms: which will track the total number of palindrome numbers found by all threads. numOfPalindromic Primes: which will count the numbers that are BOTH prime and palindrome found by all threads. TotalNums: which will count all the processed numbers in the range. In addition, you need to have arrays…arrow_forwardHow do you distinguish between hardware and a software problem? Discuss theprocedure for troubleshooting any hardware or software problem. give one reference with your answer.arrow_forward
- You are asked to explain what a computer virus is and if it can affect computer’shardware or software. How do you protect your computer against virus? give one reference with your answer.arrow_forwardDistributed Systems: Consistency Models fer to page 45 for problems on data consistency. structions: Compare different consistency models (e.g., strong, eventual, causal) for distributed databases. Evaluate the trade-offs between availability and consistency in a given use case. Propose the most appropriate model for the scenario and explain your reasoning. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440AZF/view?usp=sharing]arrow_forwardOperating Systems: Deadlock Detection fer to page 25 for problems on deadlock concepts. structions: • Given a system resource allocation graph, determine if a deadlock exists. If a deadlock exists, identify the processes and resources involved. Suggest strategies to prevent or resolve the deadlock and explain their trade-offs. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forward
- Artificial Intelligence: Heuristic Evaluation fer to page 55 for problems on Al search algorithms. tructions: Given a search problem, propose and evaluate a heuristic function. Compare its performance to other heuristics based on search cost and solution quality. Justify why the chosen heuristic is admissible and/or consistent. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 75 for graph-related problems. Instructions: • Implement a greedy graph coloring algorithm for the given graph. • Demonstrate the steps to assign colors while minimizing the chromatic number. • Analyze the time complexity and limitations of the approach. Link [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 150 for problems on socket programming. Instructions: • Develop a client-server application using sockets to exchange messages. • Implement both TCP and UDP communication and highlight their differences. • Test the program under different network conditions and analyze results. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward
- Refer to page 80 for problems on white-box testing. Instructions: • Perform control flow testing for the given program, drawing the control flow graph (CFG). • Design test cases to achieve statement, branch, and path coverage. • Justify the adequacy of your test cases using the CFG. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qo Hazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 10 for problems on parsing. Instructions: • Design a top-down parser for the given grammar (e.g., recursive descent or LL(1)). • Compute the FIRST and FOLLOW sets and construct the parsing table if applicable. • Parse a sample input string and explain the derivation step-by-step. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 20 for problems related to finite automata. Instructions: • Design a deterministic finite automaton (DFA) or nondeterministic finite automaton (NFA) for the given language. • Minimize the DFA and show all steps, including state merging. • Verify that the automaton accepts the correct language by testing with sample strings. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward
- A Guide to SQLComputer ScienceISBN:9781111527273Author:Philip J. PrattPublisher:Course Technology PtrDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781285196145Author:Steven, Steven Morris, Carlos Coronel, Carlos, Coronel, Carlos; Morris, Carlos Coronel and Steven Morris, Carlos Coronel; Steven Morris, Steven Morris; Carlos CoronelPublisher:Cengage Learning
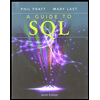
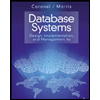