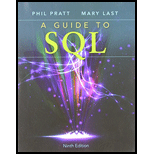
Concept explainers
a.
Stored procedures:
- When user expects to running a particular query often, user can expand total performance by saving the query in a file called a stored procedure.
- The stored procedure is located on the server.
- The DBMS compiles the stored procedure and creates an execution plan, which is the well-organized method of finding the results.
- It is a procedure which contains collection of procedural and SQL statements.
Syntax for stored procedure:
CREATE FUNCTION fun_name(argument IN data-type)RETRUN data-type[IS]
BEGIN
PL/SQL statements;
Return (value or expression);
END;

Explanation of Solution
Query to create stored procedure:
CREATE OR REPLACE PROCEDURE DISP_GUIDE (I_GUIDE_NUM IN GUIDE.GUIDE_NUM%TYPE) AS
I_LAST_NAME GUIDE.LAST_NAME%TYPE;
I_FIRST_NAME GUIDE.FIRST_NAME%TYPE;
BEGIN
SELECT LAST_NAME, FIRST_NAME
INTO I_LAST_NAME, I_FIRST_NAME
FROM GUIDE
WHERE GUIDE_NUM = I_GUIDE_NUM;
DBMS_OUTPUT.PUT_LINE(I_GUIDE_NUM);
DBMS_OUTPUT.PUT_LINE(RTRIM(I_FIRST_NAME)||' '||RTRIM(I_LAST_NAME));
END;
/
Explanation:
- The above query is used to create a procedure named “DISP_GUIDE” to select the records in the “GUIDE” table.
- Change the “GUIDE_NUM” into “I_GUIDE_NUM” and place the “LAST_NAME” and “FIRST_NAME” values into “I_LAST_NAME” and “I_FIRST_NAME”.
- After placing these values, display the “I_GUIDE_NUM” and “I_FIRST_NAME” and “I_LAST_NAME” with separated by a space from the “GUIDE” table.
- Once the stored procedure is created, it needs to be executed.
Query to view the guide number and its first name and last name of guide with space:
BEGIN
DISP_GUIDE ('GZ01');
END;
The above query is used to view the guide number and its first name and last name with separated by a space.
Output:
GZ01
Zach Gregory
Explanation of Solution
b.
Query to create stored procedure:
CREATE OR REPLACE PROCEDURE DISP_RESERVATION_INFO (I_RESERVATION_ID IN RESERVATION.RESERVATION_ID%TYPE) AS
I_NUM_PERSONS RESERVATION.NUM_PERSONS%TYPE;
I_CUSTOMER_NUM CUSTOMER.CUSTOMER_NUM%TYPE;
I_LAST_NAME CUSTOMER.LAST_NAME%TYPE;
BEGIN
SELECT NUM_PERSONS, CUSTOMER.CUSTOMER_NUM, LAST_NAME
INTO I_NUM_PERSONS, I_CUSTOMER_NUM, I_LAST_NAME
FROM RESERVATION, CUSTOMER
WHERE RESERVATION.CUSTOMER_NUM = CUSTOMER.CUSTOMER_NUM
AND RESERVATION_ID = I_RESERVATION_ID;
DBMS_OUTPUT.PUT_LINE(I_NUM_PERSONS);
DBMS_OUTPUT.PUT_LINE(I_CUSTOMER_NUM);
DBMS_OUTPUT.PUT_LINE(I_LAST_NAME);
END;
/
Explanation:
- The above query is used to create a procedure named “DISP_RESERVATION_INFO” to select the records in the “CUSTOMER” and “RESERVATION” tables.
- Change the “RESERVATION_ID” into “I_RESERVATION_ID” and place the “NUM_PERSONS”, “CUSTOMER_NUM”, and “LAST_NAME” values into “I_NUM_PERSONS”, “I_CUSTOMER_NUM”, and “I_LAST_NAME”.
- After placing these values, display the “I_NUM_PERSONS”, “I_CUSTOMER_NUM”, and “I_LAST_NAME” from the “CUSTOMER” and “RESERVATION” tables.
- Once the stored procedure is created, it needs to be executed.
Query to view the number of persons, customer number and customer last name:
BEGIN
DISP_RESERVATION_INFO (1600020);
END;
The above query is used to view the number of persons, customer number and customer last name for the reservation ID “1600020”.

Output:
2
124
Busa
Explanation of Solution
c.
Query to insert the value:
CREATE OR REPLACE PROCEDURE ADD_GUIDE
(I_GUIDE_NUM IN GUIDE.GUIDE_NUM%TYPE,
I_LAST_NAME IN GUIDE.LAST_NAME%TYPE,
I_FIRST_NAME IN GUIDE.FIRST_NAME%TYPE) AS
BEGIN
INSERT INTO GUIDE (GUIDE_NUM, LAST_NAME, FIRST_NAME)
VALUES
(I_GUIDE_NUM, I_LAST_NAME, I_FIRST_NAME);
END;
/
Explanation:
The above query is used to create a stored procedure named “ADD_GUIDE” to insert the new record in the “GUIDE” table. Once the stored procedure is created, it needs to be executed.
Query to execute the stored procedure:
BEGIN
ADD_GUIDE ('QR01', 'John', 'Merry');
END;
After executing the above query, the new record is inserted into the table “GUIDE”.

Output:
Query to view the contents in “GUIDE” table is as follows:
SELECT * FROM GUIDE;
Screenshot of output
Explanation of Solution
d.
Query to update stored procedure:
CREATE OR REPLACE PROCEDURE CHANGE_GUIDE_LASTNAME
(I_GUIDE_NUM IN GUIDE.GUIDE_NUM%TYPE,
I_LAST_NAME GUIDE.LAST_NAME%TYPE) AS
BEGIN
UPDATE GUIDE
SET LAST_NAME = I_LAST_NAME
WHERE GUIDE_NUM = I_GUIDE_NUM;
END;
/
Explanation:
The above query is used to create a stored procedure named “CHANGE_GUIDE_LASTNAME” to change the last name of the guide whose number is stored in “I_GUIDE_NUM” to the value presently found in “I_LAST_NAME”, it needs to be executed.
Executing the stored procedure:
The content of “GUIDE” table before creating the procedure is given below:
Query to view the contents in “GUIDE” table is as follows:
SELECT * FROM GUIDE;
Screenshot of output
Query to execute the stored procedure:
BEGIN
CHANGE_GUIDE_LASTNAME ('GZ01', 'Rose');
END;
/
After executing the above query, the last name is changed for the guide number “GZ01” in the “GUIDE” table.

Output:
Query to view the contents in “GUIDE” table is as follows:
SELECT * FROM GUIDE;
Screenshot of output
Explanation of Solution
e.
Query to delete the value:
CREATE OR REPLACE PROCEDURE DELETE_GUIDE_RECORD
(I_GUIDE_NUM IN GUIDE.GUIDE_NUM%TYPE) AS
BEGIN
DELETE
FROM GUIDE
WHERE GUIDE_NUM = I_GUIDE_NUM;
END;
/
Explanation:
- The above query is used to create a procedure named “DELETE_GUIDE_RECORD” to delete a record in the “GUIDE” table.
- Once the record is deleted, a procedure should create guide number as a parameter.
- Once the stored procedure is created, it needs to be executed.
Executing the stored procedure:
The content of “GUIDE” table before creating the procedure is given below:
Query to view the contents in “GUIDE” table is as follows:
SELECT * FROM GUIDE;
Screenshot of output
Query to execute the stored procedure:
BEGIN
DELETE_GUIDE_RECORD ('QR01');
END;
The above query is used to delete the record of guide number ‘QR01’.

Output:
Query to view the contents in “GUIDE” table after deleting the guide number ‘QR01’ as follows:
SELECT * FROM GUIDE;
Screenshot of output
Want to see more full solutions like this?
Chapter 8 Solutions
A Guide to SQL
- Suppose that the two Rank methods below are added to the Skip List class on Blackboard. public int Rank(T item) Returns the rank of the given item. public T Rank(int i) Returns the item with the given rank i. Requirements 1. Describe in a separate Design Document what additional data is needed and how that data is used to support an expected time complexity of O(log n) for each of the Rank methods. Show as well that the methods Insert and Remove can efficiently maintain this data as items are inserted and removed. (7 marks) 2. Re-implement the methods Insert and Remove of the Skip List class to maintain the augmented data in expected O(log n) time. Using the Contains method, ensure that added items are distinct. (6 marks) 3. Implement the two Rank methods. (8 marks) 4. Test your new methods thoroughly. Include your test cases and results in a Test Document. (4 marks)arrow_forwardWhy do we need official standards for copper cable and fiber-optic cable? What happens without the standard?arrow_forwardWhat is the difference between physical connection (Physical topology) and logical connection (Logical topology)? Why are both necessary?arrow_forward
- There are two network models and name them while providing a couple of advantages and disadvantages for each network model.arrow_forwardhttps://docs.google.com/document/d/1lk0DgaWfVezagyjAEskyPoe9Ciw3J2XUH_HQfnWSmwU/edit?usp=sharing use the link to answer the question below b) As part of your listed data elements, define the following metadata for each: Data/FieldType, Field Size, and any possible constraint/s or needed c) Identify and describe the relationship/s among the tables. Please provide an example toillustrate Referential Integrity and explain why it is essential for data credibility. I have inserted the data elements below for referencearrow_forwardWhy do we use NAT and PAT technologies? What happens without them? What is the major difference between NAT and PAT (Port Address Translation)? please answer it in the simplest way as possiblearrow_forward
- What is the difference between physical connection (Physical topology) and logical connection (Logical topology)? Why are both necessary? Why do we need the Seven-Layer OSI model? What will happen If we don’t have it? Why do we need official standards for copper cable and fiber-optic cable? What happens without the standard? please answer in the simplest way as possiblearrow_forwardSuppose that the MinGap method below is added to the Treap class on Blackboard. public int MinGap( ) Returns the absolute difference between the two closest numbers in the treap. For example, if the numbers are {2, 5, 7, 11, 12, 15, 20} then MinGap would returns 1, the absolute difference between 11 and 12. Requirements 1. Describe in a separate Design Document what additional data is needed and how that data is used to support an time complexity of O(1) for the MinGap method. Show as well that the methods Add and Remove can efficiently maintain this data as items are added and removed. (6 marks) 2. Re-implement the methods Add and Remove of the Treap class to maintain the augmented data in expected O(log n) time. (6 marks) 3. Implement the MinGap method. (4 marks) 4. Test your new method thoroughly. Include your test cases and results in a Test Document. (4 marks)arrow_forwardSuppose that the two Rank methods below are added to the Skip List class on Blackboard. public int Rank(T item) Returns the rank of the given item. public T Rank(int i) Returns the item with the given rank i. Requirements 1. Describe in a separate Design Document what additional data is needed and how that data is used to support an expected time complexity of O(log n) for each of the Rank methods. Show as well that the methods Insert and Remove can efficiently maintain this data as items are inserted and removed. (7 marks) 2. Re-implement the methods Insert and Remove of the Skip List class to maintain the augmented data in expected O(log n) time. Using the Contains method, ensure that added items are distinct. (6 marks) 3. Implement the two Rank methods. (8 marks) 4. Test your new methods thoroughly. Include your test cases and results in a Test Document. (4 marks)arrow_forward
- https://docs.google.com/document/d/1lk0DgaWfVezagyjAEskyPoe9Ciw3J2XUH_HQfnWSmwU/edit?usp=sharing use the link to answer the question below b) As part of your listed data elements, define the following metadata for each: Data/FieldType, Field Size, and any possible constraint/s or needed c) Identify and describe the relationship/s among the tables. Please provide an example toillustrate Referential Integrity and explain why it is essential for data credibility. I have inserted the data elements below for referencearrow_forwardHighlight the main differences between Computer Assisted Coding and Alone Coding with their similaritiesarrow_forwardSuppose that the MinGap method below is added to the Treap class on Blackboard. public int MinGap ( ) Returns the absolute difference between the two closest numbers in the treap. For example, if the numbers are {2, 5, 7, 11, 12, 15, 20} then MinGap would returns 1, the absolute difference between 11 and 12. Requirements 1. Describe in a separate Design Document what additional data is needed and how that data is used to support an time complexity of O(1) for the MinGap method. Show as well that the methods Add and Remove can efficiently maintain this data as items are added and removed. (6 marks) 2. Re-implement the methods Add and Remove of the Treap class to maintain the augmented data in expected O(log n) time. (6 marks) 3. Implement the MinGap method. (4 marks) 4. Test your new method thoroughly. Include your test cases and results in a Test Document. (4 marks)arrow_forward
- A Guide to SQLComputer ScienceISBN:9781111527273Author:Philip J. PrattPublisher:Course Technology PtrDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781285196145Author:Steven, Steven Morris, Carlos Coronel, Carlos, Coronel, Carlos; Morris, Carlos Coronel and Steven Morris, Carlos Coronel; Steven Morris, Steven Morris; Carlos CoronelPublisher:Cengage LearningDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781305627482Author:Carlos Coronel, Steven MorrisPublisher:Cengage Learning
- Np Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
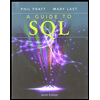
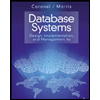
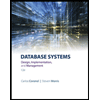
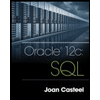