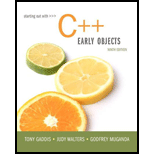
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 8, Problem 36RQE
A)
Program Plan Intro
Array:
An array is a data structure which stores multiple values of same types of data; the array values are stored in continuous memory locations.
- Size of an array has been declared inside a square bracket, which is named as size declarator.
- The size declarator must be declared as an integer with a value greater than “0” and the number inside the brackets represents the number of elements that the array can hold.
Structure array:
The structure needs an array if the items needs to be grouped together.
- Array is a “derived” data type and it contains similar elements.
- The array index starts with 0.
- If the size of the array is 4, then the index values are “0”, “1”, “2”, and “3”.
Syntax:
struct_name array_name[size];
Example:
Book name[20];
Explanation:
“Book” is a structure name, “name” is an array name, and the value “20” is the “size” of an array.
B)
Program Plan Intro
Array of Objects:
Object is an instance of the class. Objects of the class can also be given in the form of an array, in which the array values are passed into an overloaded constructor.
Syntax: classname object[size];
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Exercise
docID document text
docID document text
1
hot chocolate cocoa beans
7
sweet sugar
2345
9
cocoa ghana africa
8
sugar cane brazil
beans harvest ghana
9
sweet sugar beet
cocoa butter
butter truffles
sweet chocolate
10
sweet cake icing
11
cake black forest
Clustering by k-means, with preprocessing tokenization, term weighting TFIDF.
Manhattan Distance. Number of cluster is 2. Centroid docID 2 and docID 9.
Change the following code so that there is always at least one way to get from the left corner to the top right, but the labyrinth is still randomized.
The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. Take care that the player and the dragon cannot start off on walls. Also the dragon starts off from a randomly chosen position
public class Labyrinth { private final int size; private final Cell[][] grid;
public Labyrinth(int size) { this.size = size; this.grid = new Cell[size][size]; generateLabyrinth(); }
private void generateLabyrinth() { Random rand = new Random(); for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { // Randomly create walls and paths grid[i][j] = new Cell(rand.nextBoolean()); } } // Ensure start and end are…
Change the following code so that it checks the following 3 conditions:
1. there is no space between each cells (imgs)
2. even if it is resized, the components wouldn't disappear
3. The GameGUI JPanel takes all the JFrame space, so that there shouldn't be extra space appearing in the frame other than the game.
Main():
Labyrinth labyrinth = new Labyrinth(10);
Player player = new Player(9, 0); Dragon dragon = new Dragon(9, 9);
JFrame frame = new JFrame("Labyrinth Game"); GameGUI gui = new GameGUI(labyrinth, player, dragon);
frame.add(gui); frame.setSize(600, 600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true);
public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; //labyrinth, player, dragon are just public classes
private final ImageIcon playerIcon = new ImageIcon("data/images/player.png");…
Chapter 8 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 8.3 - Define the following arrays: A) empNum, a 100...Ch. 8.3 - Prob. 8.2CPCh. 8.3 - Prob. 8.3CPCh. 8.3 - Assume a program includes the following two...Ch. 8.3 - What is array bounds checking? Does C++ perform...Ch. 8.3 - What is the output of the following code? int...Ch. 8.3 - Complete the following program skeleton so it will...Ch. 8.7 - Define the following arrays: A) ages, a 10-element...Ch. 8.7 - Indicate if each of the following array...Ch. 8.7 - Prob. 8.10CP
Ch. 8.7 - Given the following array definition: int values...Ch. 8.7 - Prob. 8.12CPCh. 8.7 - Prob. 8.13CPCh. 8.7 - What is the output of the following code? const...Ch. 8.9 - Write a typedef statement that makes the name...Ch. 8.9 - Prob. 8.16CPCh. 8.9 - What is the output of the following program...Ch. 8.9 - The following program segments, when completed,...Ch. 8.11 - Prob. 8.19CPCh. 8.11 - Prob. 8.20CPCh. 8.11 - Prob. 8.21CPCh. 8.11 - Prob. 8.22CPCh. 8.11 - Prob. 8.23CPCh. 8.11 - Fill in the empty table below so it shows the...Ch. 8.11 - Write a function called displayArray7. The...Ch. 8.11 - Prob. 8.26CPCh. 8.12 - Prob. 8.27CPCh. 8.12 - Write definition statements for the following...Ch. 8.12 - Define gators to be an empty vector of ints and...Ch. 8.13 - True or false: The default constructor is the only...Ch. 8.13 - True or false: All elements in an array of objects...Ch. 8.13 - What will the following program display on the...Ch. 8.13 - Complete the following program so that it defines...Ch. 8.13 - Add two constructors to the Product structure...Ch. 8.13 - Prob. 8.35CPCh. 8.13 - Prob. 8.36CPCh. 8.13 - Prob. 8.37CPCh. 8.13 - Write the definition for an array of five Product...Ch. 8.13 - Write a structure declaration called Measurement...Ch. 8.13 - Write a structure declaration called Destination ,...Ch. 8.13 - Define an array of 20 Destination structures (see...Ch. 8 - The ________ indicates the number of elements, or...Ch. 8 - The size declarator must be a(n) _______ with a...Ch. 8 - Prob. 3RQECh. 8 - Prob. 4RQECh. 8 - The number inside the brackets of an array...Ch. 8 - C++ has no array ________ checking, which means...Ch. 8 - Prob. 7RQECh. 8 - If a numeric array is partially initialized, the...Ch. 8 - If the size declarator of an array definition is...Ch. 8 - Prob. 10RQECh. 8 - Prob. 11RQECh. 8 - Prob. 12RQECh. 8 - Arrays are never passed to functions by _______...Ch. 8 - To pass an array to a function, pass the ________...Ch. 8 - A(n) ________ array is like several arrays of the...Ch. 8 - Its best to think of a two -dimensional array as...Ch. 8 - Prob. 17RQECh. 8 - Prob. 18RQECh. 8 - When a two -dimensional array is passed to a...Ch. 8 - When you pass the name of an array as an argument...Ch. 8 - Look at the following array definition. int values...Ch. 8 - Given the following array definition: int values...Ch. 8 - Prob. 23RQECh. 8 - Assume that array1 and array2 are both 25-element...Ch. 8 - Prob. 25RQECh. 8 - How do you establish a parallel relationship...Ch. 8 - Look at the following array definition. double...Ch. 8 - Prob. 28RQECh. 8 - Prob. 29RQECh. 8 - Prob. 30RQECh. 8 - Prob. 31RQECh. 8 - The following code totals the values in each of...Ch. 8 - Prob. 33RQECh. 8 - Prob. 34RQECh. 8 - In a program you need to store the identification...Ch. 8 - Prob. 36RQECh. 8 - Prob. 37RQECh. 8 - Prob. 38RQECh. 8 - Each of the following functions contains errors....Ch. 8 - Soft Skills Diagrams are an important means of...Ch. 8 - Perfect Scores 1. Write a modular program that...Ch. 8 - Larger Than n Create a program with a function...Ch. 8 - Roman Numeral Converter Write a program that...Ch. 8 - Chips and Salsa Write a program that lets a maker...Ch. 8 - Monkey Business A local zoo wants to keep track of...Ch. 8 - Rain or Shine An amateur meteorologist wants to...Ch. 8 - Lottery Write a program that simulates a lottery....Ch. 8 - Rainfall Statistics Write a modular program that...Ch. 8 - Lo Shu Magic Square The Lo Shu Magic Square is a...Ch. 8 - Baseball Champions This challenge uses two files...Ch. 8 - Chips and Salsa Version 2 Revise Programming...Ch. 8 - Stats Class and Rainfall Statistics Create a Stats...Ch. 8 - Stats Class and Track Statistics Write a client...Ch. 8 - Prob. 14PCCh. 8 - Drivers License Exam The State Department of Motor...Ch. 8 - Array of Payro11 Objects Design a PayRoll class...Ch. 8 - Drink Machine Simulator Create a class that...Ch. 8 - Bin Manager Class Design and write an object...Ch. 8 - Tic-Tac-Toe Game Write a modular program that...Ch. 8 - Theater Ticket Sales Create a TicketManager class...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Make the following game user friendly with GUI, with some simple graphics. The GUI should be in another seperate class, with some ImageIcon, and Game class should be added into the pane. The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return…arrow_forwardDiscuss the negative and positive impacts or information technology in the context of your society. Provide two references along with with your answerarrow_forwardA cylinder of diameter 10 cm rotates concentrically inside another hollow cylinder of inner diameter 10.1 cm. Both cylinders are 20 cm long and stand with their axis vertical. The annular space is filled with oil. If a torque of 100 kg cm is required to rotate the inner cylinder at 100 rpm, determine the viscosity of oil. Ans. μ= 29.82poisearrow_forward
- Make the following game user friendly with GUI, with some simple graphics The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return isWall; } public void setWall(boolean isWall) { this.isWall = isWall; } @Override public String toString() {…arrow_forwardPlease original work What are four of the goals of information lifecycle management think they are most important to data warehousing, Why do you feel this way, how dashboards can be used in the process, and provide a real life example for each. Please cite in text references and add weblinksarrow_forwardThe following is code for a disc golf program written in C++: // player.h #ifndef PLAYER_H #define PLAYER_H #include <string> #include <iostream> class Player { private: std::string courses[20]; // Array of course names int scores[20]; // Array of scores int gameCount; // Number of games played public: Player(); // Constructor void CheckGame(int playerId, const std::string& courseName, int gameScore); void ReportPlayer(int playerId) const; }; #endif // PLAYER_H // player.cpp #include "player.h" #include <iomanip> Player::Player() : gameCount(0) {} void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) { for (int i = 0; i < gameCount; ++i) { if (courses[i] == courseName) { // If course has been played, then check for minimum score if (gameScore < scores[i]) { scores[i] = gameScore; // Update to new minimum…arrow_forward
- In this assignment, you will implement a multi-threaded program (using C/C++) that will check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome numbers are numbers that their decimal representation can be read from left to right and from right to left (e.g. 12321, 5995, 1234321). The program will create T worker threads to check for prime and palindrome numbers in the given range (T will be passed to the program with the Linux command line). Each of the threads works on a part of the numbers within the range. Your program should have some global shared variables: • numOfPrimes: which will track the total number of prime numbers found by all threads. numOfPalindroms: which will track the total number of palindrome numbers found by all threads. numOfPalindromic Primes: which will count the numbers that are BOTH prime and palindrome found by all threads. TotalNums: which will count all the processed numbers in the range. In addition, you need to have arrays…arrow_forwardHow do you distinguish between hardware and a software problem? Discuss theprocedure for troubleshooting any hardware or software problem. give one reference with your answer.arrow_forwardYou are asked to explain what a computer virus is and if it can affect computer’shardware or software. How do you protect your computer against virus? give one reference with your answer.arrow_forward
- Distributed Systems: Consistency Models fer to page 45 for problems on data consistency. structions: Compare different consistency models (e.g., strong, eventual, causal) for distributed databases. Evaluate the trade-offs between availability and consistency in a given use case. Propose the most appropriate model for the scenario and explain your reasoning. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440AZF/view?usp=sharing]arrow_forwardOperating Systems: Deadlock Detection fer to page 25 for problems on deadlock concepts. structions: • Given a system resource allocation graph, determine if a deadlock exists. If a deadlock exists, identify the processes and resources involved. Suggest strategies to prevent or resolve the deadlock and explain their trade-offs. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardArtificial Intelligence: Heuristic Evaluation fer to page 55 for problems on Al search algorithms. tructions: Given a search problem, propose and evaluate a heuristic function. Compare its performance to other heuristics based on search cost and solution quality. Justify why the chosen heuristic is admissible and/or consistent. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
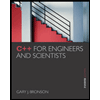
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
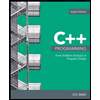
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
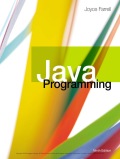
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT