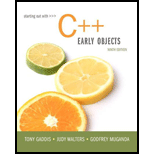
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 8, Problem 14PC
Program Plan Intro
Character Converter Class
Program Plan:
- Include required header files.
- Declare a class named “CharConverter”.
- Inside “public” access specifier,
- Declare the functions “uppercase ()” and “properWords ()”.
- Inside “public” access specifier,
- Give function definition for “uppercase ()”.
- Loop from 0 through the length of the string.
- Check if the letter is from “a” to “z”.
- Subtract “32” from the ASCII value of the letter in order to convert the letter to uppercase.
- Check if the letter is from “a” to “z”.
- Loop from 0 through the length of the string.
- Give function definition for “properWords ()”.
- Check if the length of the string is equal to 0.
- Return the statement.
- Check if the character is from “a” to “z”.
- Subtract “32” from the ASCII value of the first letter in order to convert that letter to uppercase.
- Loop from 1 through the length of the string.
- Check if the letter appear after a space are from “a” to “z”.
- Subtract “32” from the ASCII value of the letter in order to convert the letter to uppercase.
- Check if the letter appear after a space are from “a” to “z”.
- Check if the length of the string is equal to 0.
- Define a function “main ()”.
- Declare required variables “sentence” and “choice”.
- Create an object “converter” for the class.
- Do until the user enters other than the character “y” or “Y”.
- Get a sentence from the user and store it in a variable “sentence”.
- Call the function “properWords ()” and print the final result.
- Call the function “uppercase ()” and print the final result.
- Return the statement.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Why I need ?
Here are two diagrams. Make them very explicit, similar to Example Diagram 3 (the Architecture of MSCTNN).
graph LR subgraph Teacher_Model_B [Teacher Model (Pretrained)] Input_Teacher_B[Input C (Complete Data)] --> Teacher_Encoder_B[Transformer Encoder T] Teacher_Encoder_B --> Teacher_Prediction_B[Teacher Prediction y_T] Teacher_Encoder_B --> Teacher_Features_B[Internal Features F_T] end subgraph Student_B_Model [Student Model B (Handles Missing Labels)] Input_Student_B[Input C (Complete Data)] --> Student_B_Encoder[Transformer Encoder E_B] Student_B_Encoder --> Student_B_Prediction[Student B Prediction y_B] end subgraph Knowledge_Distillation_B [Knowledge Distillation (Student B)] Teacher_Prediction_B -- Logits Distillation Loss (L_logits_B) --> Total_Loss_B Teacher_Features_B -- Feature Alignment Loss (L_feature_B) --> Total_Loss_B Partial_Labels_B[Partial Labels y_p] -- Prediction Loss (L_pred_B) --> Total_Loss_B Total_Loss_B -- Backpropagation -->…
Please provide me with the output image of both of them . below are the diagrams code
I have two diagram :
first diagram code
graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder --> Student_A_Features[Student A Features F_A] end subgraph Knowledge_Distillation_A [Knowledge Distillation (Student A)] Teacher_Prediction -- Logits Distillation Loss (L_logits_A) --> Total_Loss_A Teacher_Features -- Feature Alignment Loss (L_feature_A) --> Total_Loss_A Ground_Truth_A[Ground Truth y_gt] -- Prediction Loss (L_pred_A)…
Chapter 8 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 8.3 - Define the following arrays: A) empNum, a 100...Ch. 8.3 - Prob. 8.2CPCh. 8.3 - Prob. 8.3CPCh. 8.3 - Assume a program includes the following two...Ch. 8.3 - What is array bounds checking? Does C++ perform...Ch. 8.3 - What is the output of the following code? int...Ch. 8.3 - Complete the following program skeleton so it will...Ch. 8.7 - Define the following arrays: A) ages, a 10-element...Ch. 8.7 - Indicate if each of the following array...Ch. 8.7 - Prob. 8.10CP
Ch. 8.7 - Given the following array definition: int values...Ch. 8.7 - Prob. 8.12CPCh. 8.7 - Prob. 8.13CPCh. 8.7 - What is the output of the following code? const...Ch. 8.9 - Write a typedef statement that makes the name...Ch. 8.9 - Prob. 8.16CPCh. 8.9 - What is the output of the following program...Ch. 8.9 - The following program segments, when completed,...Ch. 8.11 - Prob. 8.19CPCh. 8.11 - Prob. 8.20CPCh. 8.11 - Prob. 8.21CPCh. 8.11 - Prob. 8.22CPCh. 8.11 - Prob. 8.23CPCh. 8.11 - Fill in the empty table below so it shows the...Ch. 8.11 - Write a function called displayArray7. The...Ch. 8.11 - Prob. 8.26CPCh. 8.12 - Prob. 8.27CPCh. 8.12 - Write definition statements for the following...Ch. 8.12 - Define gators to be an empty vector of ints and...Ch. 8.13 - True or false: The default constructor is the only...Ch. 8.13 - True or false: All elements in an array of objects...Ch. 8.13 - What will the following program display on the...Ch. 8.13 - Complete the following program so that it defines...Ch. 8.13 - Add two constructors to the Product structure...Ch. 8.13 - Prob. 8.35CPCh. 8.13 - Prob. 8.36CPCh. 8.13 - Prob. 8.37CPCh. 8.13 - Write the definition for an array of five Product...Ch. 8.13 - Write a structure declaration called Measurement...Ch. 8.13 - Write a structure declaration called Destination ,...Ch. 8.13 - Define an array of 20 Destination structures (see...Ch. 8 - The ________ indicates the number of elements, or...Ch. 8 - The size declarator must be a(n) _______ with a...Ch. 8 - Prob. 3RQECh. 8 - Prob. 4RQECh. 8 - The number inside the brackets of an array...Ch. 8 - C++ has no array ________ checking, which means...Ch. 8 - Prob. 7RQECh. 8 - If a numeric array is partially initialized, the...Ch. 8 - If the size declarator of an array definition is...Ch. 8 - Prob. 10RQECh. 8 - Prob. 11RQECh. 8 - Prob. 12RQECh. 8 - Arrays are never passed to functions by _______...Ch. 8 - To pass an array to a function, pass the ________...Ch. 8 - A(n) ________ array is like several arrays of the...Ch. 8 - Its best to think of a two -dimensional array as...Ch. 8 - Prob. 17RQECh. 8 - Prob. 18RQECh. 8 - When a two -dimensional array is passed to a...Ch. 8 - When you pass the name of an array as an argument...Ch. 8 - Look at the following array definition. int values...Ch. 8 - Given the following array definition: int values...Ch. 8 - Prob. 23RQECh. 8 - Assume that array1 and array2 are both 25-element...Ch. 8 - Prob. 25RQECh. 8 - How do you establish a parallel relationship...Ch. 8 - Look at the following array definition. double...Ch. 8 - Prob. 28RQECh. 8 - Prob. 29RQECh. 8 - Prob. 30RQECh. 8 - Prob. 31RQECh. 8 - The following code totals the values in each of...Ch. 8 - Prob. 33RQECh. 8 - Prob. 34RQECh. 8 - In a program you need to store the identification...Ch. 8 - Prob. 36RQECh. 8 - Prob. 37RQECh. 8 - Prob. 38RQECh. 8 - Each of the following functions contains errors....Ch. 8 - Soft Skills Diagrams are an important means of...Ch. 8 - Perfect Scores 1. Write a modular program that...Ch. 8 - Larger Than n Create a program with a function...Ch. 8 - Roman Numeral Converter Write a program that...Ch. 8 - Chips and Salsa Write a program that lets a maker...Ch. 8 - Monkey Business A local zoo wants to keep track of...Ch. 8 - Rain or Shine An amateur meteorologist wants to...Ch. 8 - Lottery Write a program that simulates a lottery....Ch. 8 - Rainfall Statistics Write a modular program that...Ch. 8 - Lo Shu Magic Square The Lo Shu Magic Square is a...Ch. 8 - Baseball Champions This challenge uses two files...Ch. 8 - Chips and Salsa Version 2 Revise Programming...Ch. 8 - Stats Class and Rainfall Statistics Create a Stats...Ch. 8 - Stats Class and Track Statistics Write a client...Ch. 8 - Prob. 14PCCh. 8 - Drivers License Exam The State Department of Motor...Ch. 8 - Array of Payro11 Objects Design a PayRoll class...Ch. 8 - Drink Machine Simulator Create a class that...Ch. 8 - Bin Manager Class Design and write an object...Ch. 8 - Tic-Tac-Toe Game Write a modular program that...Ch. 8 - Theater Ticket Sales Create a TicketManager class...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- I'm reposting my question again please make sure to avoid any copy paste from the previous answer because those answer did not satisfy or responded to the need that's why I'm asking again The knowledge distillation part is not very clear in the diagram. Please create two new diagrams by separating the two student models: First Diagram (Student A - Missing Values): Clearly illustrate the student training process. Show how knowledge distillation happens between the teacher and Student A. Explain what the teacher teaches Student A (e.g., handling missing values) and how this teaching occurs (e.g., through logits, features, or attention). Second Diagram (Student B - Missing Labels): Similarly, detail the training process for Student B. Clarify how knowledge distillation works between the teacher and Student B. Specify what the teacher teaches Student B (e.g., dealing with missing labels) and how the knowledge is transferred. Since these are two distinct challenges…arrow_forwardThe knowledge distillation part is not very clear in the diagram. Please create two new diagrams by separating the two student models: First Diagram (Student A - Missing Values): Clearly illustrate the student training process. Show how knowledge distillation happens between the teacher and Student A. Explain what the teacher teaches Student A (e.g., handling missing values) and how this teaching occurs (e.g., through logits, features, or attention). Second Diagram (Student B - Missing Labels): Similarly, detail the training process for Student B. Clarify how knowledge distillation works between the teacher and Student B. Specify what the teacher teaches Student B (e.g., dealing with missing labels) and how the knowledge is transferred. Since these are two distinct challenges (missing values vs. missing labels), they should not be combined in the same diagram. Instead, create two separate diagrams for clarity. For reference, I will attach a second image…arrow_forwardNote : please avoid using AI answer the question by carefully reading it and provide a clear and concise solutionHere is a clear background and explanation of the full method, including what each part is doing and why. Background & Motivation Missing values: Some input features (sensor channels) are missing for some samples due to sensor failure or corruption. Missing labels: Not all samples have a ground-truth RUL value. For example, data collected during normal operation is often unlabeled. Most traditional deep learning models require complete data and full labels. But in our case, both are incomplete. If we try to train a model directly, it will either fail to learn properly or discard valuable data. What We Are Doing: Overview We solve this using a Teacher–Student knowledge distillation framework: We train a Teacher model on a clean and complete dataset where both inputs and labels are available. We then use that Teacher to teach two separate Student models: Student A learns…arrow_forward
- Here is a clear background and explanation of the full method, including what each part is doing and why. Background & Motivation Missing values: Some input features (sensor channels) are missing for some samples due to sensor failure or corruption. Missing labels: Not all samples have a ground-truth RUL value. For example, data collected during normal operation is often unlabeled. Most traditional deep learning models require complete data and full labels. But in our case, both are incomplete. If we try to train a model directly, it will either fail to learn properly or discard valuable data. What We Are Doing: Overview We solve this using a Teacher–Student knowledge distillation framework: We train a Teacher model on a clean and complete dataset where both inputs and labels are available. We then use that Teacher to teach two separate Student models: Student A learns from incomplete input (some sensor values missing). Student B learns from incomplete labels (RUL labels missing…arrow_forwardhere is a diagram code : graph LR subgraph Inputs [Inputs] A[Input C (Complete Data)] --> TeacherModel B[Input M (Missing Data)] --> StudentA A --> StudentB end subgraph TeacherModel [Teacher Model (Pretrained)] C[Transformer Encoder T] --> D{Teacher Prediction y_t} C --> E[Internal Features f_t] end subgraph StudentA [Student Model A (Trainable - Handles Missing Input)] F[Transformer Encoder S_A] --> G{Student A Prediction y_s^A} B --> F end subgraph StudentB [Student Model B (Trainable - Handles Missing Labels)] H[Transformer Encoder S_B] --> I{Student B Prediction y_s^B} A --> H end subgraph GroundTruth [Ground Truth RUL (Partial Labels)] J[RUL Labels] end subgraph KnowledgeDistillationA [Knowledge Distillation Block for Student A] K[Prediction Distillation Loss (y_s^A vs y_t)] L[Feature Alignment Loss (f_s^A vs f_t)] D -- Prediction Guidance --> K E -- Feature Guidance --> L G --> K F --> L J -- Supervised Guidance (if available) --> G K…arrow_forwarddetails explanation and background We solve this using a Teacher–Student knowledge distillation framework: We train a Teacher model on a clean and complete dataset where both inputs and labels are available. We then use that Teacher to teach two separate Student models: Student A learns from incomplete input (some sensor values missing). Student B learns from incomplete labels (RUL labels missing for some samples). We use knowledge distillation to guide both students, even when labels are missing. Why We Use Two Students Student A handles Missing Input Features: It receives input with some features masked out. Since it cannot see the full input, we help it by transferring internal features (feature distillation) and predictions from the teacher. Student B handles Missing RUL Labels: It receives full input but does not always have a ground-truth RUL label. We guide it using the predictions of the teacher model (prediction distillation). Using two students allows each to specialize in…arrow_forward
- We are doing a custom JSTL custom tag to make display page to access a tag handler. Write two custom tags: 1) A single tag which prints a number (from 0-99) as words. Ex: <abc:numAsWords val="32"/> --> produces: thirty-two 2) A paired tag which puts the body in a DIV with our team colors. Ex: <abc:teamColors school="gophers" reverse="true"> <p>Big game today</p> <p>Bring your lucky hat</p> <-- these will be green text on blue background </abc:teamColors> Details: The attribute for numAsWords will be just val, from 0 to 99 - spelling, etc... isn't important here. Print "twenty-six" or "Twenty six" ... . Attributes for teamColors are: school, a "required" string, and reversed, a non-required boolean. - pick any four schools. I picked gophers, cyclones, hawkeyes and cornhuskers - each school has two colors. Pick whatever seems best. For oine I picked "cyclones" and red text on a gold body - if…arrow_forwardI want a database on MySQL to analyze blood disease analyses with a selection of all its commands, with an ER drawing, and a complete chart for normalization. I want them completely.arrow_forwardAssignment Instructions: You are tasked with developing a program to use city data from an online database and generate a city details report. 1) Create a new Project in Eclipse called "HW7". 2) Create a class "City.java" in the project and implement the UML diagram shown below and add comments to your program. 3) The logic for the method "getCityCategory" of City Class is below: a. If the population of a city is greater than 10000000, then the method returns "MEGA" b. If the population of a city is greater than 1000000 and less than 10000000, then the method returns "LARGE" c. If the population of a city is greater than 100000 and less than 1000000, then the method returns "MEDIUM" d. If the population of a city is below 100000, then the method returns "SMALL" 4) You should create another new Java program inside the project. Name the program as "xxxx_program.java”, where xxxx is your Kean username. 3) Implement the following methods inside the xxxx_program program The main method…arrow_forward
- CPS 2231 - Computer Programming – Spring 2025 City Report Application - Due Date: Concepts: Classes and Objects, Reading from a file and generating report Point value: 40 points. The purpose of this project is to give students exposure to object-oriented design and programming using classes in a realistic application that involves arrays of objects and generating reports. Assignment Instructions: You are tasked with developing a program to use city data from an online database and generate a city details report. 1) Create a new Project in Eclipse called "HW7”. 2) Create a class "City.java" in the project and implement the UML diagram shown below and add comments to your program. 3) The logic for the method "getCityCategory" of City Class is below: a. If the population of a city is greater than 10000000, then the method returns "MEGA" b. If the population of a city is greater than 1000000 and less than 10000000, then the method returns "LARGE" c. If the population of a city is greater…arrow_forwardPlease calculate the average best-case IPC attainable on this code with a 2-wide, in-order, superscalar machine: ADD X1, X2, X3 SUB X3, X1, 0x100 ORR X9, X10, X11 ADD X11, X3, X2 SUB X9, X1, X3 ADD X1, X2, X3 AND X3, X1, X9 ORR X1, X11, X9 SUB X13, X14, X15 ADD X16, X13, X14arrow_forwardOutline the overall steps for configuring and securing Linux servers Consider and describe how a mixed Operating System environment will affect what you have to do to protect the company assets Describe at least three technologies that will help to protect CIA of data on Linux systemsarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
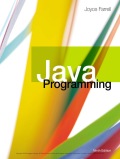
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
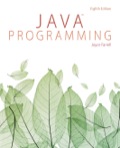
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
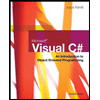
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
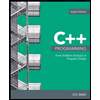
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
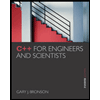
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage