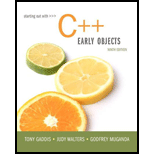
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 8.7, Problem 8.12CP
Program Plan Intro
Partial initialization of an array:
The C++ program allows the user to initialize the elements of an array while creating the array; the initialization list stores the value in an array in the specific order as they are initialized.
- Additionally, while initializing the array it is no need to initialize all the elements of an array; the uninitialized elements of the array will be set to “0” by default.
- For the “string” array, it stores the empty strings for uninitialized elements.
- If a local array is completely uninitialized, then the elements are stored with the garbage values.
For example:
Consider the following snippet,
// Constant for the array size
const int num_of_days = 6;
// Initialization list
int days[num_of_days] = {1, 2, 3};
In the above example, the sequence of values stored inside the braces and separated with commas is referred as the initialization list.
- The values are stored in the array in below format:
days[0] = 1;
days[1] = 2;
days[2] = 3;
days[3] = 0;
days[4] = 0;
days[5] = 0;
Thus, if an array is partially initialized, then the uninitialized elements will be assigned as “0”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
comprog array declaration
Given the following array definition
int nums[5] = {1, 2, 3};what will the following statement display?cout << nums[3];
Mcq:
How do you initialize an array in C?
A) int arr(3) = {1,2,3};
B) int arr[3] = {1,2,3};
Chapter 8 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 8.3 - Define the following arrays: A) empNum, a 100...Ch. 8.3 - Prob. 8.2CPCh. 8.3 - Prob. 8.3CPCh. 8.3 - Assume a program includes the following two...Ch. 8.3 - What is array bounds checking? Does C++ perform...Ch. 8.3 - What is the output of the following code? int...Ch. 8.3 - Complete the following program skeleton so it will...Ch. 8.7 - Define the following arrays: A) ages, a 10-element...Ch. 8.7 - Indicate if each of the following array...Ch. 8.7 - Prob. 8.10CP
Ch. 8.7 - Given the following array definition: int values...Ch. 8.7 - Prob. 8.12CPCh. 8.7 - Prob. 8.13CPCh. 8.7 - What is the output of the following code? const...Ch. 8.9 - Write a typedef statement that makes the name...Ch. 8.9 - Prob. 8.16CPCh. 8.9 - What is the output of the following program...Ch. 8.9 - The following program segments, when completed,...Ch. 8.11 - Prob. 8.19CPCh. 8.11 - Prob. 8.20CPCh. 8.11 - Prob. 8.21CPCh. 8.11 - Prob. 8.22CPCh. 8.11 - Prob. 8.23CPCh. 8.11 - Fill in the empty table below so it shows the...Ch. 8.11 - Write a function called displayArray7. The...Ch. 8.11 - Prob. 8.26CPCh. 8.12 - Prob. 8.27CPCh. 8.12 - Write definition statements for the following...Ch. 8.12 - Define gators to be an empty vector of ints and...Ch. 8.13 - True or false: The default constructor is the only...Ch. 8.13 - True or false: All elements in an array of objects...Ch. 8.13 - What will the following program display on the...Ch. 8.13 - Complete the following program so that it defines...Ch. 8.13 - Add two constructors to the Product structure...Ch. 8.13 - Prob. 8.35CPCh. 8.13 - Prob. 8.36CPCh. 8.13 - Prob. 8.37CPCh. 8.13 - Write the definition for an array of five Product...Ch. 8.13 - Write a structure declaration called Measurement...Ch. 8.13 - Write a structure declaration called Destination ,...Ch. 8.13 - Define an array of 20 Destination structures (see...Ch. 8 - The ________ indicates the number of elements, or...Ch. 8 - The size declarator must be a(n) _______ with a...Ch. 8 - Prob. 3RQECh. 8 - Prob. 4RQECh. 8 - The number inside the brackets of an array...Ch. 8 - C++ has no array ________ checking, which means...Ch. 8 - Prob. 7RQECh. 8 - If a numeric array is partially initialized, the...Ch. 8 - If the size declarator of an array definition is...Ch. 8 - Prob. 10RQECh. 8 - Prob. 11RQECh. 8 - Prob. 12RQECh. 8 - Arrays are never passed to functions by _______...Ch. 8 - To pass an array to a function, pass the ________...Ch. 8 - A(n) ________ array is like several arrays of the...Ch. 8 - Its best to think of a two -dimensional array as...Ch. 8 - Prob. 17RQECh. 8 - Prob. 18RQECh. 8 - When a two -dimensional array is passed to a...Ch. 8 - When you pass the name of an array as an argument...Ch. 8 - Look at the following array definition. int values...Ch. 8 - Given the following array definition: int values...Ch. 8 - Prob. 23RQECh. 8 - Assume that array1 and array2 are both 25-element...Ch. 8 - Prob. 25RQECh. 8 - How do you establish a parallel relationship...Ch. 8 - Look at the following array definition. double...Ch. 8 - Prob. 28RQECh. 8 - Prob. 29RQECh. 8 - Prob. 30RQECh. 8 - Prob. 31RQECh. 8 - The following code totals the values in each of...Ch. 8 - Prob. 33RQECh. 8 - Prob. 34RQECh. 8 - In a program you need to store the identification...Ch. 8 - Prob. 36RQECh. 8 - Prob. 37RQECh. 8 - Prob. 38RQECh. 8 - Each of the following functions contains errors....Ch. 8 - Soft Skills Diagrams are an important means of...Ch. 8 - Perfect Scores 1. Write a modular program that...Ch. 8 - Larger Than n Create a program with a function...Ch. 8 - Roman Numeral Converter Write a program that...Ch. 8 - Chips and Salsa Write a program that lets a maker...Ch. 8 - Monkey Business A local zoo wants to keep track of...Ch. 8 - Rain or Shine An amateur meteorologist wants to...Ch. 8 - Lottery Write a program that simulates a lottery....Ch. 8 - Rainfall Statistics Write a modular program that...Ch. 8 - Lo Shu Magic Square The Lo Shu Magic Square is a...Ch. 8 - Baseball Champions This challenge uses two files...Ch. 8 - Chips and Salsa Version 2 Revise Programming...Ch. 8 - Stats Class and Rainfall Statistics Create a Stats...Ch. 8 - Stats Class and Track Statistics Write a client...Ch. 8 - Prob. 14PCCh. 8 - Drivers License Exam The State Department of Motor...Ch. 8 - Array of Payro11 Objects Design a PayRoll class...Ch. 8 - Drink Machine Simulator Create a class that...Ch. 8 - Bin Manager Class Design and write an object...Ch. 8 - Tic-Tac-Toe Game Write a modular program that...Ch. 8 - Theater Ticket Sales Create a TicketManager class...
Knowledge Booster
Similar questions
- Look at the following array definition. int numbers [] = {2, 4, 6, 8, 10};What will the following statement display?cout << *(numbers + 3) << end1;arrow_forwardReverse ArrayWrite a function that accepts an int array and the array’s size as arguments. The function should create a copy of the array, except that the element values should be reversedin the copy. The function should return a pointer to the new array. Demonstrate thefunction in a complete program.arrow_forwardLotto Program C++Write a program that simulates the Powerball lottery. In Powerball, a ticket is comprised of 5 numbers between 1 and 69 that must be unique, and a Powerball number between 1 and 26. The Powerball does not have to be unique. Hint: You can use an array to represent the 5 unique numbers between 1 and 69, and an integer variable to represent the powerball. The program asks the player if they'd like to select numbers or do a 'quickpick', where the numbers are randomly generated for them. If they opt to select numbers, prompt them to type the numbers in and validate that they are unique (except the powerball), and in the correct range. The program then generates a 'drawing' of 5 unique numbers and a Powerball, and checks it against the user's lotto ticket. It assigns winnings accordingly. Because it is so rare to win the lottery, I suggest hard coding or printing values to when testing the part of the program that assigns winnings.arrow_forward
- Code for this in C: You have already created an array named NUMBERS with 20cells. You are to initialize all of the cells with odd subscripts to 1 and all of the cells with even subscripts to 2.arrow_forward1. Array Allocator Write a function that dynamically allocates an array of integers. The function should accept an integer argument indicating the number of elements to allocate. The function should return a pointer to the array.arrow_forwardPointers: P3: Given the following string, after you call func2, if you printed out the array, what would be printed out? string a = {"c","a","n", func2(a); "y"}; void func2(string *arr) { arr[3) - "t"; arr[2]="vi"; %3Darrow_forward
- 2. How do you initialize an array in C? a) int arr[3] = (1,2,3); b) int arr(3) = {1,2,3}; c) int arr[3] = {1,2,3}; d) int arr(3) = (1,2,3);arrow_forwardWrite the definition of a function named total that receives two parameters: an array ary of element type double and an int called size that contains the number of elements of the array. The function returns the sum of the elements of the array as a double. Use a for loop in your function definition. (8%) Write the prototype for the function total. (3%) Call function total with the array called myArray that contains 6 elements and assign the result to double myTotal. (3%)arrow_forwardProgramming in C SearchArray Use the following starter program: #include #include #define NUMNUMS 9 int main) int dataſ] = [ 4, 5, 8, 9, 13, 22, 44, 55, 65 ): return 0; Write code that does the following: 1. Ask the user for a number to search for. 2. Search the data array to see if the number is there. 3. If the number is there, say number found, otherwise say number not found. Please enter a number for which you would like to search:22 number found Process returned e (exe) Press any key to continue. execution time : 2.467 sarrow_forward
- 2. Intersection Write a method/function that takes two circular arrays, their sizes and start indexes and returns a linear array containing the common elements between the two circular arrays. DO NOT convert the circular arrays to linear arrays to solve the problem. Input: Circular array 1: [40,50,0,0,0,10,20,30] (start_1 =5, size_1 =5) Circular array 2 : [10,20,5,0,0,0,0,0,5,40,15,25] (start_2=8, size_2 =7) Output: [10,20,40] Use Python Languagearrow_forwardLook at the following array definition. int numbers[5] = { 1, 2, 3 };A) What value is stored in numbers[2]?B) What value is stored in numbers[4]?arrow_forwardC++ Write a program that populates an array with numbers and allows the user to run some actions on the array. Your array will be initialized in your main function to a constant global value, MAX_SIZE, which should be set to 20. To perform the subtasks, you will be writing three functions: fillArray: This function takes in an array of integers and a reference to an integer representing the number of filled elements. In this function, the user is prompred to enter a positive number that is no greater than 20, and this input will loop until the user enters a legal value. This value will be filled inside of the reference parameter mentioned above. From here, the array will be filled up to "value" elements with randomly generated numbers between 1 and 100. Make sure to set the random seed to 20 at the start of main() for consistent results. displayArray: This function takes in an array of integers and an integer representing the number of filled elements. Using a loop, all elements in the…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
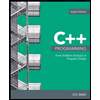
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning