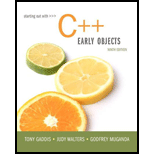
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 8.7, Problem 8.10CP
Program Plan Intro
Array:
An array is a data structure which stores multiple values of same types of data; the array values are stored in continuous memory locations.
- Size of an array has been declared inside a square bracket, which is named as size declarator.
- The size declarator must be declared as an integer with a value greater than “0” and the number inside the brackets represents the number of elements that the array can hold.
Syntax:
Syntax to declare an array,
data_type array_name[size_declarator];
Example:
Example to declare an array,
//Declaration of an array
int values[5];
In the above example, “int” represents the data type of the array, “values” is the array name and the number inside the bracket is the size declarator of the array.
- Here, the array “values” holds “5” values.
- The array index values starts from “0” and ends with “4” to hold five elements.
Given code:
/*Array declaration of type double that is assigned to hold four values. */
double array1[4] = {1.2, 3.2 , 4.2, 5.2};
/*Array declaration of type double that can hold four values. */
double array2[4] ;
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
2 #include
3 #include
4
5
6
7
8 void printArray(int myArr[][ARRAY_COLS])
9- {
10
11-
12
13-
const int ARRAY_COLS = 10;
3;
const int ARRAY_ROWS
14
15
16
17
18 }
19
20- /*
21
26
27 }
37 }
38
44
45
46
47
48
49
50
for (int i = 0; i < ARRAY_ROWS; i++)
{
22
23
*/
24 void zeroCorners (int myArr[] [ARRAY_COLS])
25 - {
myArr[0][0] = 999;
51
52
}
This function currently sets the upper-left-hand corder of the 2D array to 999
Instead, this should set all 4 corners of the 2-D array to zero.
for (int j = 0; j < ARRAY_COLS; j++)
{
std::cout << myArr[i][j] << ", ";
}
28
29 - /*
30 This function should Loop through every element in the 2-D array.
If an element is odd, then subtract one from to make it even.
31
32
HINT: function printArray() is an example of Looping through every element in 2D array
*/
33
34 void makeEven (int myArr[][ARRAY_COLS])
35 - {
36
// TODO - put code here
std::cout << "\n";
53
54 }
55
39 int main()
40 - {
41 -
42
43
int arr[][ARRAY_COLS] {
{1, 2, 3, 4, 5, 6, 7, 8, 9, 10),…
JAVA PROGRAM
Chapter 7. PC #16. 2D Array Operations
Write a program that creates a two-dimensional array initialized with test data. Use any primitive data type that you wish. The program should have the following methods:
• getTotal. This method should accept a two-dimensional array as its argument and return the total of all the values in the array.
• getAverage. This method should accept a two-dimensional array as its argument and return the average of all the values in the array.
• getRowTotal. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The method should return the total of the values in the specified row.
• getColumnTotal. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The method should return the…
IN C#
Q. Lucky Number Definition:● A lucky number is a number whose value is equal to the occurrence of that number in anarray.○ [2, 2, 3, 3] => 2● If there is more than one number whose value is equal to the occurrence of that numberin an array, then the lucky number will be the one with the maximum value among alllucky numbers.○ [1, 2, 2, 3, 3, 3, 4] => 3● If there is no number in an array whose value is equal to the occurrence of that numberin the array then the lucky number will be -1.○ [5] => -1Implement a function getLuckyNumber(numbers) that takes an array of positive integers asinput and returns the lucky number from the array.Test Cases:● getLuckyNumber([2, 2, 3, 3]) => 2● getLuckyNumber([1, 2, 2, 3, 3, 3, 4]) => 3● getLuckyNumber([5]) => -1getLuckyNumber(numbers){// function implementation// return number whose value is equal to the occurrence of the number in the array// e.g [2, 2, 3, 3] => lucky number = 2// in case there are multiple lucky numbers…
Chapter 8 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 8.3 - Define the following arrays: A) empNum, a 100...Ch. 8.3 - Prob. 8.2CPCh. 8.3 - Prob. 8.3CPCh. 8.3 - Assume a program includes the following two...Ch. 8.3 - What is array bounds checking? Does C++ perform...Ch. 8.3 - What is the output of the following code? int...Ch. 8.3 - Complete the following program skeleton so it will...Ch. 8.7 - Define the following arrays: A) ages, a 10-element...Ch. 8.7 - Indicate if each of the following array...Ch. 8.7 - Prob. 8.10CP
Ch. 8.7 - Given the following array definition: int values...Ch. 8.7 - Prob. 8.12CPCh. 8.7 - Prob. 8.13CPCh. 8.7 - What is the output of the following code? const...Ch. 8.9 - Write a typedef statement that makes the name...Ch. 8.9 - Prob. 8.16CPCh. 8.9 - What is the output of the following program...Ch. 8.9 - The following program segments, when completed,...Ch. 8.11 - Prob. 8.19CPCh. 8.11 - Prob. 8.20CPCh. 8.11 - Prob. 8.21CPCh. 8.11 - Prob. 8.22CPCh. 8.11 - Prob. 8.23CPCh. 8.11 - Fill in the empty table below so it shows the...Ch. 8.11 - Write a function called displayArray7. The...Ch. 8.11 - Prob. 8.26CPCh. 8.12 - Prob. 8.27CPCh. 8.12 - Write definition statements for the following...Ch. 8.12 - Define gators to be an empty vector of ints and...Ch. 8.13 - True or false: The default constructor is the only...Ch. 8.13 - True or false: All elements in an array of objects...Ch. 8.13 - What will the following program display on the...Ch. 8.13 - Complete the following program so that it defines...Ch. 8.13 - Add two constructors to the Product structure...Ch. 8.13 - Prob. 8.35CPCh. 8.13 - Prob. 8.36CPCh. 8.13 - Prob. 8.37CPCh. 8.13 - Write the definition for an array of five Product...Ch. 8.13 - Write a structure declaration called Measurement...Ch. 8.13 - Write a structure declaration called Destination ,...Ch. 8.13 - Define an array of 20 Destination structures (see...Ch. 8 - The ________ indicates the number of elements, or...Ch. 8 - The size declarator must be a(n) _______ with a...Ch. 8 - Prob. 3RQECh. 8 - Prob. 4RQECh. 8 - The number inside the brackets of an array...Ch. 8 - C++ has no array ________ checking, which means...Ch. 8 - Prob. 7RQECh. 8 - If a numeric array is partially initialized, the...Ch. 8 - If the size declarator of an array definition is...Ch. 8 - Prob. 10RQECh. 8 - Prob. 11RQECh. 8 - Prob. 12RQECh. 8 - Arrays are never passed to functions by _______...Ch. 8 - To pass an array to a function, pass the ________...Ch. 8 - A(n) ________ array is like several arrays of the...Ch. 8 - Its best to think of a two -dimensional array as...Ch. 8 - Prob. 17RQECh. 8 - Prob. 18RQECh. 8 - When a two -dimensional array is passed to a...Ch. 8 - When you pass the name of an array as an argument...Ch. 8 - Look at the following array definition. int values...Ch. 8 - Given the following array definition: int values...Ch. 8 - Prob. 23RQECh. 8 - Assume that array1 and array2 are both 25-element...Ch. 8 - Prob. 25RQECh. 8 - How do you establish a parallel relationship...Ch. 8 - Look at the following array definition. double...Ch. 8 - Prob. 28RQECh. 8 - Prob. 29RQECh. 8 - Prob. 30RQECh. 8 - Prob. 31RQECh. 8 - The following code totals the values in each of...Ch. 8 - Prob. 33RQECh. 8 - Prob. 34RQECh. 8 - In a program you need to store the identification...Ch. 8 - Prob. 36RQECh. 8 - Prob. 37RQECh. 8 - Prob. 38RQECh. 8 - Each of the following functions contains errors....Ch. 8 - Soft Skills Diagrams are an important means of...Ch. 8 - Perfect Scores 1. Write a modular program that...Ch. 8 - Larger Than n Create a program with a function...Ch. 8 - Roman Numeral Converter Write a program that...Ch. 8 - Chips and Salsa Write a program that lets a maker...Ch. 8 - Monkey Business A local zoo wants to keep track of...Ch. 8 - Rain or Shine An amateur meteorologist wants to...Ch. 8 - Lottery Write a program that simulates a lottery....Ch. 8 - Rainfall Statistics Write a modular program that...Ch. 8 - Lo Shu Magic Square The Lo Shu Magic Square is a...Ch. 8 - Baseball Champions This challenge uses two files...Ch. 8 - Chips and Salsa Version 2 Revise Programming...Ch. 8 - Stats Class and Rainfall Statistics Create a Stats...Ch. 8 - Stats Class and Track Statistics Write a client...Ch. 8 - Prob. 14PCCh. 8 - Drivers License Exam The State Department of Motor...Ch. 8 - Array of Payro11 Objects Design a PayRoll class...Ch. 8 - Drink Machine Simulator Create a class that...Ch. 8 - Bin Manager Class Design and write an object...Ch. 8 - Tic-Tac-Toe Game Write a modular program that...Ch. 8 - Theater Ticket Sales Create a TicketManager class...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- c plus plus language , please solve itarrow_forward1. Given the following array declaration: int A [3 ][2 ]={{13, -23}, {3, 80} ,{91,55} }; declare a pointer that points to A[2][0]? Answer:arrow_forwardAnalyze the statements below: double [] templ = {50.0, 69.0, 75.0, 80.0, 55.0}; printArray (temp1); The call to printArray sends the of/to the array temp1.arrow_forward
- Write the definition of a function named total that receives two parameters: an array ary of element type double and an int called size that contains the number of elements of the array. The function returns the sum of the elements of the array as a double. Use a for loop in your function definition. (8%) Write the prototype for the function total. (3%) Call function total with the array called myArray that contains 6 elements and assign the result to double myTotal. (3%)arrow_forwardJava:arrow_forwardProblem Description - JAVA PROGRAMMING Use a Two-dimensional (3x3) array to solve the following problem: Write an application that inputs nine numbers, each of which is between 1 and 10, inclusive. Display the array after the user inputs each value. Rotate/flip the array by changing places. Make the rows columns and vice versa. You have to move the elements to their new locations. Remember to validate the input and display an error message if the user inputs invalid data. Documentation and the screenshot(s) of the results. Example: 1 2 3 4 5 6 7 8 9 the result will be : 1 4 7 2 5 8 3 6 9arrow_forward
- Java - Functions with 1D Arrays Create a program that asks the user for the size of an integer array and the elements of the array. Then, create a function called maxArray() with the following details: Parameters: An integer array The size of the integer array Return type - int Return value - the maximum value of the integer array Call the function you created in the main and pass the integer array and its size to it. Make sure to store the return value in a variable so you could print it afterwards. Input 1. First line contains the size of the array 2. Succeeding lines are the elements of an array 3. First line contains the size of the array 4. Succeeding lines are the elements of an array 5. First line contains the size of the array 6. Succeeding lines are the elements of an array 7. First line contains the size of the array 8. Succeeding lines are the elements of an array Output Enter size of array: 5 Enter element 1: 1 Enter element 2: 2 Enter…arrow_forwardint[] array = { 1, 4, 3, 6 }; What would be a proper syntax to access 3 from this array?arrow_forwarda. double [] al = new double(2); b. double [] a2 = new {2.5}: c. double [] a4 = {5, 2}: d. double [] a3 2. Which of the following is correct array declaration? = new {5.1. 2.8}:arrow_forward
- JAVA ARRAY MANIPULATION QUESTION: make a method called manipulator that takes a double array as parameter and modifies it. The method will modify the array then print the array. The method must modify the existing array, it CAN NOT create a different array as the solution. The manipulator method will be a void method. Remember: To get an average of 2 digits you add them together then divide by 2. Average of x and y = (x+y)/2 Modifications the method will do: For every 2 consecutive elements in the array, both the elements are replaced by their average. If the number of elements in an array is odd don't modify the last number of the array. Basically, you find the average of 2 consecutive elements then replace both of the elements with that value. Examples: Array = {2.0,3.0} will be changed to {2.5,2.5} when passed to the manipulator method Array = {2.0,3.0,45} will be changed to {2.5,2.5,45} when passed to the manipulator method Array = {2.0,3.0,45,55} will be changed to…arrow_forward2. How do you initialize an array in C? a) int arr[3] = (1,2,3); b) int arr(3) = {1,2,3}; c) int arr[3] = {1,2,3}; d) int arr(3) = (1,2,3);arrow_forwardProgram 3 SRC voting application You must write a small application in JAVA that stores SRC candidate names and the votes they received. Your program must: 1. Create an array of SRC candidate names. Populate the array with ten names. 2. Create an array of votes. Populate the array with the number of votes for every candidate. The two arrays work in parallel. 3. Print the name of every candidate with his/her number of votes. 4. Print the total number of votes casted. 5. Add votes for a specific candidate. a. Read the name of the candidate b. Add one vote for the specific candidate C. Print the name of the candidate and his/her number of votesarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
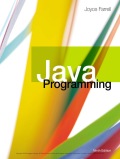
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT