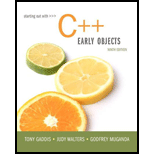
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 8, Problem 33RQE
Program Plan Intro
Structure array:
The structure needs an array if the items need to be grouped together.
- Array is a “derived” data type and it contains similar elements.
- The array index starts with 0.
- If the size of the array is 4, then the index values are “0”, “1”, “2”, and “3”.
Syntax:
struct_name array_name[size];
Example:
Book name[20];
Explanation:
“Book” is a structure name, “name” is an array name, and the value “20” is the “size” of an array.
Algorithm to define an array of structure to hold 12 records about city population and read the data from the specified file “pop.dat” and store it in structure members.
- Define the structure Population.
- Declare the structure variables such as city name and its population.
- Declare an array of structure cntry to hold 12 records.
- Define the main function
- Declare a file stream object.
- Open the file “pop.dat” using the object.
- Using for loop,
- Read city name and its population from the file and store it in structure members.
- Close the file.
- Read city name and its population from the file and store it in structure members.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
My daughter is a Girl Scout and it is time for our cookie sales. There are 15 neighbors nearby and she plans to visit every neighbor this evening. There is a 40% likelihood that someone will be home. If someone is home, there is an 85% likelihood that person will make a purchase. If a purchase is made, the revenue generated from the sale follows the Normal distribution with mean $18 and standard deviation $5.
Using @RISK, simulate our door-to-door sales using at least 1000 iterations and report the expected revenue, the maximum revenue, and the average number of purchasers. What is the probability that the revenue will be greater than $120?
Q4 For the network of Fig. 1.41:
a- Determine re
b- Find Aymid
=VolVi
=Vo/Vi
c- Calculate Zi.
d- Find Ay
smid
e-Determine fL, JLC, and fLE
f-Determine the low cutoff frequency.
g- Sketch the asymptotes of the Bode plot defined by the cutoff frequencies of part (e).
h-Sketch the low-frequency response for the amplifier using the results of part (f).
Ans: 28.48 2, -72.91, 2.455 KS2, -54.68, 103.4 Hz. 38.05 Hz. 235.79 Hz. 235.79 Hz.
14V
15.6ΚΩ
68kQ
0.47µF
Vo
0.82 ΚΩ
V₁
B-120
3.3kQ
0.47µF
10kQ
1.2k0
=20µF
Z₁
Fig. 1.41 Circuit for
a. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table
shown below:
A
B
C
Y
0
0
0
1
0
0
1
0
0
1
0
0
0
1
1
0
1
0
0
1
1
0
1
0
1
1
1
1
0
1
1
0
a. [10 pts] Minimize the Boolean equation you obtained in (a).
b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of
the circuit in your report.
Chapter 8 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 8.3 - Define the following arrays: A) empNum, a 100...Ch. 8.3 - Prob. 8.2CPCh. 8.3 - Prob. 8.3CPCh. 8.3 - Assume a program includes the following two...Ch. 8.3 - What is array bounds checking? Does C++ perform...Ch. 8.3 - What is the output of the following code? int...Ch. 8.3 - Complete the following program skeleton so it will...Ch. 8.7 - Define the following arrays: A) ages, a 10-element...Ch. 8.7 - Indicate if each of the following array...Ch. 8.7 - Prob. 8.10CP
Ch. 8.7 - Given the following array definition: int values...Ch. 8.7 - Prob. 8.12CPCh. 8.7 - Prob. 8.13CPCh. 8.7 - What is the output of the following code? const...Ch. 8.9 - Write a typedef statement that makes the name...Ch. 8.9 - Prob. 8.16CPCh. 8.9 - What is the output of the following program...Ch. 8.9 - The following program segments, when completed,...Ch. 8.11 - Prob. 8.19CPCh. 8.11 - Prob. 8.20CPCh. 8.11 - Prob. 8.21CPCh. 8.11 - Prob. 8.22CPCh. 8.11 - Prob. 8.23CPCh. 8.11 - Fill in the empty table below so it shows the...Ch. 8.11 - Write a function called displayArray7. The...Ch. 8.11 - Prob. 8.26CPCh. 8.12 - Prob. 8.27CPCh. 8.12 - Write definition statements for the following...Ch. 8.12 - Define gators to be an empty vector of ints and...Ch. 8.13 - True or false: The default constructor is the only...Ch. 8.13 - True or false: All elements in an array of objects...Ch. 8.13 - What will the following program display on the...Ch. 8.13 - Complete the following program so that it defines...Ch. 8.13 - Add two constructors to the Product structure...Ch. 8.13 - Prob. 8.35CPCh. 8.13 - Prob. 8.36CPCh. 8.13 - Prob. 8.37CPCh. 8.13 - Write the definition for an array of five Product...Ch. 8.13 - Write a structure declaration called Measurement...Ch. 8.13 - Write a structure declaration called Destination ,...Ch. 8.13 - Define an array of 20 Destination structures (see...Ch. 8 - The ________ indicates the number of elements, or...Ch. 8 - The size declarator must be a(n) _______ with a...Ch. 8 - Prob. 3RQECh. 8 - Prob. 4RQECh. 8 - The number inside the brackets of an array...Ch. 8 - C++ has no array ________ checking, which means...Ch. 8 - Prob. 7RQECh. 8 - If a numeric array is partially initialized, the...Ch. 8 - If the size declarator of an array definition is...Ch. 8 - Prob. 10RQECh. 8 - Prob. 11RQECh. 8 - Prob. 12RQECh. 8 - Arrays are never passed to functions by _______...Ch. 8 - To pass an array to a function, pass the ________...Ch. 8 - A(n) ________ array is like several arrays of the...Ch. 8 - Its best to think of a two -dimensional array as...Ch. 8 - Prob. 17RQECh. 8 - Prob. 18RQECh. 8 - When a two -dimensional array is passed to a...Ch. 8 - When you pass the name of an array as an argument...Ch. 8 - Look at the following array definition. int values...Ch. 8 - Given the following array definition: int values...Ch. 8 - Prob. 23RQECh. 8 - Assume that array1 and array2 are both 25-element...Ch. 8 - Prob. 25RQECh. 8 - How do you establish a parallel relationship...Ch. 8 - Look at the following array definition. double...Ch. 8 - Prob. 28RQECh. 8 - Prob. 29RQECh. 8 - Prob. 30RQECh. 8 - Prob. 31RQECh. 8 - The following code totals the values in each of...Ch. 8 - Prob. 33RQECh. 8 - Prob. 34RQECh. 8 - In a program you need to store the identification...Ch. 8 - Prob. 36RQECh. 8 - Prob. 37RQECh. 8 - Prob. 38RQECh. 8 - Each of the following functions contains errors....Ch. 8 - Soft Skills Diagrams are an important means of...Ch. 8 - Perfect Scores 1. Write a modular program that...Ch. 8 - Larger Than n Create a program with a function...Ch. 8 - Roman Numeral Converter Write a program that...Ch. 8 - Chips and Salsa Write a program that lets a maker...Ch. 8 - Monkey Business A local zoo wants to keep track of...Ch. 8 - Rain or Shine An amateur meteorologist wants to...Ch. 8 - Lottery Write a program that simulates a lottery....Ch. 8 - Rainfall Statistics Write a modular program that...Ch. 8 - Lo Shu Magic Square The Lo Shu Magic Square is a...Ch. 8 - Baseball Champions This challenge uses two files...Ch. 8 - Chips and Salsa Version 2 Revise Programming...Ch. 8 - Stats Class and Rainfall Statistics Create a Stats...Ch. 8 - Stats Class and Track Statistics Write a client...Ch. 8 - Prob. 14PCCh. 8 - Drivers License Exam The State Department of Motor...Ch. 8 - Array of Payro11 Objects Design a PayRoll class...Ch. 8 - Drink Machine Simulator Create a class that...Ch. 8 - Bin Manager Class Design and write an object...Ch. 8 - Tic-Tac-Toe Game Write a modular program that...Ch. 8 - Theater Ticket Sales Create a TicketManager class...
Knowledge Booster
Similar questions
- Using XML, design a simple user interface for a fictional app. Your UI should include at least three different UI components (e.g., TextView, Button, EditText). Explain the purpose of each component in your design-you need to add screenshots of your work with your name as part of the code to appear on the interface-. Screenshot is needed.arrow_forwardQ4) A thin ring of radius 5 cm is placed on plane z = 1 cm so that its center is at (0,0,1 cm). If the ring carries 50 mA along a^, find H at (0,0,a).arrow_forward4. [15 pts] A logic function F of four variables a; b; c; d is described by the following K-map. Derive the fully minimized SOP logic expression form of F. cd ab 00 01 11 10 00 0 0 0 1 01 1 0 0 1 11 1 0 1 1 10 0 0 1 1arrow_forward
- 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisimarrow_forwardHow would I go about creating this computer database in MariaDB with sql? Create a database name "dbXXXXXX" Select the database using the "use [database name]" command. Now you are in the database. Based on the above schema from Enrolment System database, create all the tables with the last 6 digits of "123456", then the table name for table Lecturer should be "123456_Lecturer". Refer to basic SQL lecture note to create table that has primary keys and Foreign Keys. Provide the datatype of each attributes. Add a column called "Department" with datatype "VARCHAR(12)" to the table "Lecturer". Shows the metadata of the updated "Lecturer" table. (Use Describe command) Drop the "Department" column from the table "Lecturer", and show the metadata of the updated "Lecturer" table. Insert three (3) data to each of the table in the tables created. Note: If you have foreign key issues, please disable foreign key constraints before inserting the data, see below SET FOREIGN_KEY_CHECKS=0;…arrow_forwardCSE330 Discrete Mathematics 1. In the classes, we discussed three forms of floating number representations as given below, (1) Standard/General Form, (2) Normalized Form, (3) Denormalized Form. 3. Consider the real number x = (3.395) 10 (a) (b) Convert the decimal number x into binary format up to 7 binary places (7 binary digits after decimal) Convert the calculated value into denormalized form and calculate fl(x) for m=4 Don't use any Al tool show answer in pen a nd paper then take pi ctures and sendarrow_forward
- Simplify the following expressions by means of a four-variable K-Map. AD+BD+ BC + ABDarrow_forwardCSE330 Discrete Mathematics 1. In the classes, we discussed three forms of floating number representations as given below, (1) Standard/General Form, (2) Normalized Form, (3) Denormalized Form. 2. Let ẞ 2, m = 6, emin = -3 and emax = 3. Answer the following questions: Compute the minimum of |x| for General and Normalized form (a) Compute the Machine Epsilon value for the General and Denormalized form. If we change the value of emax to 6 then how will it affect the value of maximum scale invariant error for the case of Normalized form? Explain your answer. show answer in pen a Don't use any Al tool nd paper then take pi ctures and sendarrow_forwardCSE330: Discrete Mathematics 1. In the classes, we discussed three forms of floating number representations as given below, (1) Standard/General Form, (2) Normalized Form, (3) Denormalized Form. Now, let's take, ẞ = 2, m = 3, emin = -2 and emax = 3. Based on these, answer the following: (a) (b) (c) (d) What are the maximum/largest numbers that can be stored in the system by these three forms defined above? (express your answer in decimal values) What are the non-negative minimum/smallest numbers that can be stored in the system by the denormalized form? (express your answer in decimal values) How many numbers (both non-negative and negative) can be represented in the above mentioned system using the general form? Explain your answer. Find all the decimal numbers for e = 3 and e = 2 in denormalized form, plot them on a real line and prove that all the numbers are not equally spaced. Write the equally spaced sets for the number line you drew. show your answer in Don't use any Al tool pen…arrow_forward
- 3.[20 pts] Find the minimum equivalent circuit for the one shown below (show your work): DAB 0 f(A,B,C,D)arrow_forwardSuppose your computer is responding very slowly to information requests from the Internet. You observe that your network gateway shows high levels of network activity even though you have closed your e-mail client, Web browser, and all other programs that access the Internet. What types of malwares could cause such symptoms? What steps can you take to check whether malware has gained access to your system? What tools can you use at each step? If you identify malware, what ways might it have entered your system? How can you restore your PC to safe operation, including the special software tools you may use?arrow_forwardR languagearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
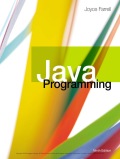
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
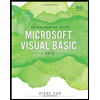
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
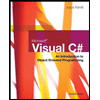
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
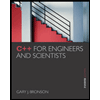
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
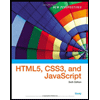
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning