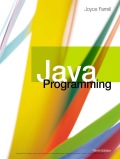
EBK JAVA PROGRAMMING
9th Edition
ISBN: 9781337671385
Author: FARRELL
Publisher: CENGAGE LEARNING - CONSIGNMENT
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 5, Problem 3GZ
Program Plan Intro
Card game
Program plan:
- In a file “Card.java”, create a class “Card”,
- Declare and initialize the necessary variables.
- Define the method “get_Suit()” to return the suit value.
- Define the method “get_Value()” to return the value.
- Define the method “set_Suit()” to set the suit value.
- Define the method “set_Value()”,
- Check whether the value is greater than or equal to low and less than or equal to high value,
- If it is true, set the given integer as the value.
- Otherwise,
- Set the lowest value.
- Check whether the value is greater than or equal to low and less than or equal to high value,
- In a file “War.java”, create a class “War”,
- Define the method “main ()”,
- Declare and initialize the necessary variables.
- Create two objects for “Card” class.
- Generate the random value for the player.
- Generate the random value for the computer.
- Set the value for the player and the computer.
- Generate the random suit for the player and the computer.
- Check whether the random value and the suite value of the player is same as that of computer,
- If it is true, increment the computer’s suit value by “1”.
- Check whether the computer’s suit value is greater than the highest value,
- Set the computer’s suit value to “1”.
- Check whether the player’s suit is “1”,
- If it is true, call the method “set_Suit()” with “s” as the parameter.
- Otherwise, check whether the player’s suit is “2”,
- If it is true, call the method “set_Suit()” with “h” as the parameter.
- Otherwise, check whether the player’s suit is “3”,
- If it is true, call the method “set_Suit()” with “d” as the parameter.
- Otherwise,
- Call the method “set_Suit()” with “c” as the parameter.
- Check whether the computer’s suit value is “1”,
- If it is true, call the method “set_Suit()” with “s” as the parameter.
- Otherwise, check whether the computer’s suit is “2”,
- If it is true, call the method “set_Suit()” with “h” as the parameter.
- Otherwise, check whether the computer’s suit is “3”,
- If it is true, call the method “set_Suit()” with “d” as the parameter.
- Otherwise,
- Call the method “set_Suit()” with “c” as the parameter.
- Print the messages in the console.
- Check whether the player’s value is same as the computer’s value,
- If it is true, print the string “It’s a tie”.
- Otherwise, check whether the player’s value is greater than the computer’s value,
- If it is true, print the string “I win”.
- Otherwise, print the string “You win”.
- Define the method “main ()”,
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
2. Signed Integers
Unsigned binary numbers work for natural numbers, but many calculations use negative
numbers as well. To deal with this, a number of different methods have been used to represent
signed numbers, but we will focus on two's complement, as it is the standard solution for
representing signed integers.
2.1 Two's complement
• Most significant bit has a negative value, all others are positive. So, the value of an n-digit
-2
two's complement number can be written as: Σ2 2¹ di 2n-1 dn
• Otherwise exactly the same as unsigned integers.
i=0
-
• A neat trick for flipping the sign of a two's complement number: flip all the bits (0 becomes 1,
or 1 becomes 0) and then add 1 to the least significant bit.
• Addition is exactly the same as with an unsigned number.
2.2 Exercises
For questions 1-3, answer each one for the case of a two's complement number and an
unsigned number, indicating if it cannot be answered with a specific representation.
1. (15 pts) What is the largest integer…
can u solve this question
1. Unsigned Integers
If we have an n-digit unsigned numeral dn-1d n-2...do in radix (or base) r, then the value of that
numeral is
n−1
r² di
Σi=0
which is basically saying that instead of a 10's or 100's place we have an r's or
r²'s place. For binary, decimal, and hex r equals 2, 10, and 16, respectively.
Just a reminder that in order to write down a large number, we typically use the IEC or SI
prefixing system:
IEC: Ki = 210, Mi = 220, Gi = 230, Ti = 240, Pi = 250, Ei = 260, Zi = 270, Yi = 280;
SI: K=103, M = 106, G = 109, T = 10¹², P = 1015, E = 10¹8, Z = 1021, Y = 1024.
1.1 Conversions
a. (15 pts) Write the following using IEC prefixes: 213, 223, 251, 272, 226, 244
21323 Ki8 Ki
223 23 Mi 8 Mi
b. (15 pts) Write the following using SI prefixes: 107, 10¹7, 10¹¹, 1022, 1026, 1015
107 10¹ M = 10 M
=
1017102 P = 100 P
c. (10 pts) Write the following with powers of 10: 7 K, 100 E, 21 G
7 K = 7*10³
Chapter 5 Solutions
EBK JAVA PROGRAMMING
Ch. 5 - Prob. 1RQCh. 5 - Prob. 2RQCh. 5 - Prob. 3RQCh. 5 - Prob. 4RQCh. 5 - Prob. 5RQCh. 5 - Prob. 6RQCh. 5 - Prob. 7RQCh. 5 - Prob. 8RQCh. 5 - Prob. 9RQCh. 5 - Prob. 10RQ
Ch. 5 - Prob. 11RQCh. 5 - Prob. 12RQCh. 5 - Prob. 13RQCh. 5 - Prob. 14RQCh. 5 - Prob. 16RQCh. 5 - Prob. 17RQCh. 5 - Prob. 18RQCh. 5 - Prob. 19RQCh. 5 - Prob. 20RQCh. 5 - Prob. 1PECh. 5 - Prob. 2PECh. 5 - Prob. 3PECh. 5 - Prob. 4PECh. 5 - Prob. 5PECh. 5 - Prob. 6PECh. 5 - Prob. 7PECh. 5 - Prob. 8PECh. 5 - Prob. 9PECh. 5 - Prob. 10PECh. 5 - Prob. 1GZCh. 5 - Prob. 2GZCh. 5 - Prob. 3GZCh. 5 - Prob. 4GZCh. 5 - Prob. 5GZ
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- answer shoul avoid using AI and should be basic and please explainarrow_forwardNode A is connected to node B by a 2000km fiber link having a bandwidth of 100Mbps. What is the total latency time (transmit + propagation) required to transmit a 4000 byte file using packets that include 1000 Bytes of data plus 40 Bytes of header.arrow_forwardanswer should avoid using AI and should be basic and explain pleasearrow_forward
- answer should avoid using AI (such as ChatGPT), do not any answer directly copied from AI would and explain codearrow_forwardanswer should avoid using AI (such as ChatGPT), do not any answer directly copied from AI would and explain codearrow_forwardWrite a c++ program that will count from 1 to 10 by 1. The default output should be: 1, 2, 3, 4, 5, 6 , 7, 8, 9, 10 There should be only a newline after the last number. Each number except the last should be followed by a comma and a space. To make your program more functional, you should parse command line arguments and change behavior based on their values. Argument Parameter Action -f, --first yes, an integer Change place you start counting -l, --last yes, an integer Change place you end counting -s, --skip optional, an integer, 1 if not specified Change the amount you add to the counter each iteration -h, —help none Print a help message including these instructions. -j, --joke none Tell a number based joke. So, if your program is called counter, counter -f 10 --last 4 --skip 2 should produce 10, 8, 6, 4 Please use the last supplied argument. If your code is called counter, counter -f 4 -f 5 -f 6 should count from 6. You should…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
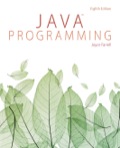
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
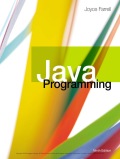
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
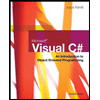
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
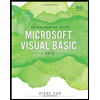
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
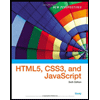
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY