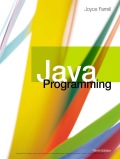
EBK JAVA PROGRAMMING
9th Edition
ISBN: 9781337671385
Author: FARRELL
Publisher: CENGAGE LEARNING - CONSIGNMENT
expand_more
expand_more
format_list_bulleted
Question
Chapter 5, Problem 7PE
Program Plan Intro
Job application
Program plan:
- In a file “JobApplicant.java”, create a class “JobApplicant”,
- Declare the necessary variables.
- Define the constructor,
- Assign the initial values for the job applicant.
- Define the method “get_Name()”,
- Return the name.
- Define the method “get_Phone()”,
- Return the phone number.
- Define the method “get_HasWordSkill()”,
- Return the Boolean value.
- Define the method “get_HasSpreadsheetSkill()”,
- Return the Boolean value.
- Define the method “get_HasDatabaseSkill()”,
- Return the Boolean value.
- Define the method “get_HasGraphicsSkill()”,
- Return the Boolean value.
- In a file “TestJobApplicants.java”, create a class “TestJobApplicants”,
- Define the method “main ()”,
- Call the constructor “JobApplicant()” that instantiates several job applicant objects.
- Assign the text messages.
- Execute for “true” statement for the first applicant.
- Call the method “display1()” with qualified message.
- Otherwise, call the method “display1()” with non-qualified message.
- Execute for “true” statement for the second applicant.
-
- Call the method “display1()” with qualified message.
- Otherwise, call the method “display1()” with non-qualified message.
- Execute for “true” statement for the third applicant.
-
- Call the method “display1()” with qualified message.
- Otherwise, call the method “display1()” with non-qualified message.
- Execute for “true” statement for the fourth applicant.
-
- Call the method “display1()” with qualified message.
- Otherwise, call the method “display1()” with non-qualified message.
- Call the method “display1()” with qualified message.
- Define the method “is_Qualified()” that accepts each job applicant properties,
- Set the count to “0”.
- Declare Boolean variable.
- Declare the final variable.
- Execute for the Boolean value at “word”,
- Increment the count by “1”.
- Execute for the Boolean value at “sp”,
-
- Increment the count by “1”.
- Execute for the Boolean value at “db”,
-
- Increment the count by “1”.
- Execute for the Boolean value at “gr”,
-
- Increment the count by “1”.
- Check whether the count is greater than or equal to minimum number of skills,
-
- If it is true, set the Boolean value to “true”.
- Otherwise, set the Boolean value to “false”.
- Return the Boolean value.
- Increment the count by “1”.
- Define the method “display1()”,
- Print the output.
- Define the method “main ()”,
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
Create a class that holds data about a job applicant. Include a name, a phone number, and four Boolean fields that represent whether the applicant is skilled in each of the following areas: word processing, spreadsheets, databases, and graphics. Include a constructor that accepts values for each of the fields. Also
include a get method for each field. Create an application that instantiates several job applicant objects and pass each in turn to a Boolean method that determines whether each applicant is qualified for an interview. Then, in the main() method, display an appropriate method for each applicant. A qualified applicant has at least three of the four skills. Save the files as JobApplicant.java and TestJobApplicants.java.
Using oop in java
Create a class for Subject containing the Name of the subject and score of the subject. There should be following methods:
set: it will take two arguments name and score, and set the values of the attributes. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero.
set: it will take one double value as argument and set the value of score only. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero.
display: it will display the name and score of the subject. Like “Name : Math, Score: 99.9”
getScore: it will return the value of score.
greaterThan: it will take subject’s object as argument, compare the calling object’s score with argument object’s score and return true if the calling object has greater score.
Static & Not Final Field: Accessed by every object, Changing
Non-Static & Final Field: Accessed by object itself, Non-Changing
Static & Final: Accessed by every object, Non-Changing
Non-Static & Not Final Field: Accessed by object itself, ChangingRead the following situation and decide how the variables should be defined.
You have a class named HeartsPlayerA round of Hearts starts with every player having 13 cardsPlayers then choose 3 cards to “trade” with a player (1st you pass left, 2nd you pass right, 3rd you pass across, 4th you keep)Players then strategically play cards in order to have the lowest scoreAt the end of the round, points are cumulatively totaled for each player.If one player’s total is greater than 100, the game ends and the player with the lowest score wins.
1. How should the following data fields be defined (with respect to final and static)?(a) playerPosition (These have values of North, South, East, or West)(b) directionOfPassing(c) totalScore…
Chapter 5 Solutions
EBK JAVA PROGRAMMING
Ch. 5 - Prob. 1RQCh. 5 - Prob. 2RQCh. 5 - Prob. 3RQCh. 5 - Prob. 4RQCh. 5 - Prob. 5RQCh. 5 - Prob. 6RQCh. 5 - Prob. 7RQCh. 5 - Prob. 8RQCh. 5 - Prob. 9RQCh. 5 - Prob. 10RQ
Ch. 5 - Prob. 11RQCh. 5 - Prob. 12RQCh. 5 - Prob. 13RQCh. 5 - Prob. 14RQCh. 5 - Prob. 16RQCh. 5 - Prob. 17RQCh. 5 - Prob. 18RQCh. 5 - Prob. 19RQCh. 5 - Prob. 20RQCh. 5 - Prob. 1PECh. 5 - Prob. 2PECh. 5 - Prob. 3PECh. 5 - Prob. 4PECh. 5 - Prob. 5PECh. 5 - Prob. 6PECh. 5 - Prob. 7PECh. 5 - Prob. 8PECh. 5 - Prob. 9PECh. 5 - Prob. 10PECh. 5 - Prob. 1GZCh. 5 - Prob. 2GZCh. 5 - Prob. 3GZCh. 5 - Prob. 4GZCh. 5 - Prob. 5GZ
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- __eq__(self, other): Method that returns True if self and other are considered the same Flight: if the origin and destination are the same for both Flights. Make sure that if “other” variable is not a Flight object, this means False should be returned. getFlightNumber(self): Getter that returns the Flight number getStart(self): Getter that returns the Plane Start getgoingTo(self): Getter that returns the Plane destination isDomesticFlight(self): Method that returns True if the flight is domestic, EX within a country (the Start and goingTo are in the same country); returns False if the flight is international (the Start and goingTo are in different countries) setStart(self, origin): Setter that sets (updates) the Plane Start setgoingTo(self, destination): Setter that sets (updates) the Plane GoingToarrow_forwardin C#, Visual Basic Create a Car class that has at least the following properties:• Year• Make• Price• Passengers-holds the number of seat belts available in the car (presumably how many passengers the car can hold). This field must be private since users presumably can’t change this value. Have two constructors: one that initializes everything to some default value for the base price of a car and one that also accepts values for luxury upgrades of: heated seats, sun-roof, and satellite radio. The class has two methods: 1) Get Paint Color() which accepts a standard base color option but allows the user to purchase a pricier customized color 2) Upgrade Costs() which computes the cost of user-selected luxury items and adds it to the price. Create three car objects: Ford (make Explorer), Lexus (make ES) and a Clown Car which overrides the passenger value to allow 20 clowns in the car. The Get Paint Color() and Upgrade Costs() functions needs to be called for each object and data for all…arrow_forwardCreate a class for Subject containing the Name of the subject and score of the subject. There should be following methods: set: it will take two arguments name and score, and set the values of the attributes. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. set: it will take one double value as argument and set the value of score only. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. display: it will display the name and score of the subject. Like “Name : Math, Score: 99.9” getScore: it will return the value of score. greaterThan: it will take subject’s object as argument, compare the calling object’s score with argument object’s score and return true if the calling object has greater score. Create a class “Main” having main method to perform following tasks. Create two objects of Subject class having value “Math, 99.9”…arrow_forward
- Create a class for Subject containing the Name of the subject and score of the subject. There should be following methods: set: it will take two arguments name and score, and set the values of the attributes. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. set: it will take one double value as argument and set the value of score only. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. display: it will display the name and score of the subject. Like “Name : Math, Score: 99.9” getScore: it will return the value of score. greaterThan: it will take subject’s object as argument, compare the calling object’s score with argument object’s score and return true if the calling object has greater score. note....... solve with javaarrow_forwardA company accepts user orders by part numbers interactively. Users might make the following errors as they enter data: - The part number is not numeric. The quantity is not numeric. - The part number is too low (less than 0). - The part number is too high (more than 999). - The quantity ordered is too low (less than 1). - The quantity ordered is too high (more than 5,000). Create a class that stores an array of usable error messages; save the file as DataMessages.java. Create a DataException class; each object of this class will store one of the messages. Save the file as DataException.java. Create an application that prompts the user for a part number and quantity. Allow for the possibility of nonnumeric entries as well as out-of-range entries, and display the appropriate message when an error occurs. If no error occurs, display the message "Valid entry". Save the program as PartAndQuantityEntry.java.arrow_forwardCreate an application named SalesTransactiobDemo that declares several SalesTransaction objects and displays their values and their sum. Name - The salesperson name (as a string) sales Amount- The sales amount (as a double) commission- The commission (as a double) RATE- A readonly field that stores the commission rate (as a double). Define a getRate() avcessor method that returns the RATE Include 3 constructors for the class. One constructor accepts values for the name, sales amount, and rate, and when the sales value is set, the constructor computes the commission as sales value times commission rate. The second constructor accepts a name and sales amount, but sets the commission rate to 0 The third constructor accepts a name and sets all the other fields to 0arrow_forward
- mau Open Leathing inta... - The class has data members that can hold the name of your cube, length of one of the sides and the color of your cube. - The class has a constructor that accepts the name, length of one of the sides, and color as arguments and sets the data members to those values. - The class has the following methods to set the corresponding data member. setName(string newName) setSide(double newSide) setColor(string newColor) - The class has a method called getVolume() that returns the volume of the cube, calculated by cubing the length of the side. - The class has a method called volumelncrease(double newVolume) that receive the percent the volume should increase, and then the side to the corresponding value. i - For example, 2.5 indicates 2.5% increasing of the volume. So you take the current volume, increase it by 2.5%, and then find the cube root to calculate the new side length. You can use the built in function called cbrt, part of the math library, to find the cube…arrow_forwardQuestionarrow_forwardDirections This exercise will provide you with the opportunity to utilize NetBeans to create an application that calculates interest. End Result: Console Create the Scanner object. Get the loan information from the end user by using the System class. Calculate the interest amount by using the BigDecimal class to make sure that all calculations are accurate. Format the interest rate, interest amount and loan amount and interest by using the NumberFormat abstract base class. It should round the interest that’s calculated to two decimal places, rounding up if the third decimal place is five or greater. The value for the formatted interest rate should allow for up to 3 decimal places. Display the results by using the System class. Assume that the user will enter valid double values for the loan amount and interest rate. The application should continue only if the user enters “y” or “Y” to continue.arrow_forward
- Using JAVASCRIPT write an object prototype for a Person that has a name and age, has a printInfo method, and also has a method that increments the persons age by 1 each time the method is called. Create two people using the 'new' keyword, and print both of their infos and increment one persons age by 3 years. Use an arrow function for both methods */ // Create our Person Prototype // Use an arrow to create the printInfo method // Create another arrow function for the addAge method that takes a single parameter // Adding to the agearrow_forwardWrite a program named DemoJobs for Harolds Home Services. The program should instantiate several Job objects and demonstrate their methods. The Job class contains four data fields—description (for example, wash windows), time in hours to complete (for example, 3.5), per-hour rate charged (for example, $25.00), and total fee (hourly rate times hours). Include properties to get and set each field except the total fee—that field will be read-only, and its value is calculated each time either the hourly fee or the number of hours is set. Overload the + operator so that two Jobs can be added. The sum of two Jobs is a new Job containing the descriptions of both original Jobs (joined by and), the sum of the time in hours for the original Jobs, and the average of the hourly rate for the original Jobs. Harold has realized that his method for computing the fee for combined jobs is not fair. For example, consider the following: His fee for painting a house is $100 per hour. If a job takes 10 hours, he earns $1000. His fee for dog walking is $10 per hour. If a job takes 1 hour, he earns $10. If he combines the two jobs and works a total of 11 hours, he earns only the average rate of $55 per hour, or $605. Devise an improved, weighted method for calculating Harolds fees for combined Jobs and include it in the overloaded operator+() method. Write a program named DemoJobs2 that demonstrates all the methods in the class work correctly.arrow_forwardCreate an application named CarDemo that declares at least two Car objects and demonstrates how they can be incremented using an overloaded ++ operator. Create a Car class that contains a model and a value for miles per gallon. Include two overloaded constructors. One accepts parameters for the model and miles per gallon; the other accepts a model and sets the miles per gallon to 20. Overload a ++ operator that increases the miles per gallon value by 1. The CarDemo application creates at least one Car using each constructor and displays the Car values both before and after incrementation.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
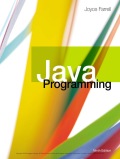
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
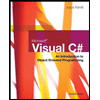
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
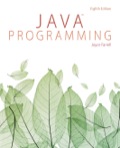
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Memory Management Tutorial in Java | Java Stack vs Heap | Java Training | Edureka; Author: edureka!;https://www.youtube.com/watch?v=fM8yj93X80s;License: Standard YouTube License, CC-BY