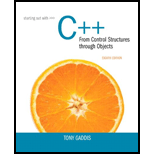
Starting Out with C++ from Control Structures to Objects (8th Edition)
8th Edition
ISBN: 9780133769395
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 18, Problem 7PC
Program Plan Intro
Dynamic MathStack
Program Plan:
MathStack.h:
- Include required header files
- Declare a class named “MathStack”. Inside the class,
- Inside “public” access specifier,
- Declare functions “add ()”, “sub ()”, “mult ()”, “div ()”, “addAll ()”, and “multAll ()”.
- Inside “public” access specifier,
MathStack.cpp:
- Include required header files.
- Give function definition to add elements “add()”.
- Declare required variables “number”, and “sum_Value”.
- Call the function “pop()”.
- Add the elements.
- Push the value into the stack using the function “push()”.
- Give function definition to subtract elements “sub()”.
- Declare required variables “number”, and “diff_Value”.
- Call the function “pop()”.
- Subtract the elements.
- Push the value into the stack using the function “push()”.
- Give function definition to multiply elements “mult()”.
- Declare required variables “number”, and “prod_Value”.
- Call the function “pop()”.
- Multiply the elements.
- Push the value into the stack using the function “push ()”.
- Give function definition to divide elements “div()”.
- Declare required variables “number”, and “quo_Value”.
- Call the function “pop()”.
- Divide the elements.
- Push the value into the stack using the function “push()”.
- Give function definition to add all the elements “addAll()”.
- Declare required variables “number”, and “sum_Value”.
- Call the function “pop()”.
- Add all the elements.
- Push the value into the stack using the function “push()”.
- Give function definition to multiply all the elements “multAll()”.
- Declare required variables “number”, and “prod_Value”.
- Call the function “pop()”.
- Multiply all the elements.
- Push the value into the stack using the function “push()”.
IntStack.h:
- Include required files.
- Declare a class named “IntStack”. Inside the class,
- Inside “protected” access specifier,
- Declare a pointer named “stackArray”.
- Declare variables “stackSize” and “top”.
- Inside “public” access specifier,
- Declare constructor and destructor.
- Give function declarations.
- Inside “protected” access specifier,
IntStack.cpp:
- Declare required header files.
- Give definition for constructor,
- Create a stack array and assign the size
- Give definition for destructor,
- Delete the array and assign it to null
- Give function definition to push elements “push()”
- Check if the stack is full using the function “isFull()”,
- If the condition is true then, print “The stack is full”.
- If the condition is not true then,
- Increment the variable.
- Assign “num” to the top position.
- Check if the stack is full using the function “isFull()”,
- Give function definition to pop elements “pop ()”,
- Check if the stack is empty using the function “isEmpty()”.
- If the condition is true then, print “The stack is empty”.
- Assign top element to the variable.
- Decrement the variable.
- If the condition is true then, print “The stack is empty”.
- Check if the stack is empty using the function “isEmpty()”.
- Give function definition to check if the stack is full “isFull()”.
- Assign “false” to the Boolean variable “status”.
- Check if the stack size if full.
- Assign true
- Return the Boolean variable “status”.
- Give function definition to check if the stack is empty “isEmpty()”.
- Assign “false” to the Boolean variable
- Check if top is empty
- Assign true.
- Return the variable
Main.cpp:
- Include required header files.
- Inside “main ()” function,
- Declare constant variables “STACKSIZE”, “ADDSIZE”, and “MULTSIZE”.
- Create three stacks “stack”, “addAllStack”, and “multAllStack”.
- Declare a variable “popVar”.
- Push two elements to perform add operation.
- Call the function “add()”.
- Display the element.
- Push two elements to perform multiplication operation.
- Call the function “mult()”.
- Display the element.
- Push two elements to perform division operation.
- Call the function “div()”.
- Display the element.
- Push two elements to perform subtraction operation.
- Call the function “sub()”.
- Display the element.
- Push four elements to perform addAll operation.
- Call the function “addAll()”.
- Display the element.
- Push six elements to perform multAll operation.
- Call the function “multAll()”.
- Display the element.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Show all the work
Show all the work
Show all the work
Chapter 18 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Ch. 18.3 - Describe what LIFO means.Ch. 18.3 - What is the difference between static and dynamic...Ch. 18.3 - What are the two primary stack operations?...Ch. 18.3 - What STL types does the STL stack container adapt?Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - What is the difference between a static stack and...Ch. 18 - Prob. 4RQECh. 18 - Prob. 5RQECh. 18 - The STL stack is considered a container adapter....
Ch. 18 - What types may the STL stack be based on? By...Ch. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - The STL stack container is an adapter for the...Ch. 18 - Prob. 18RQECh. 18 - Prob. 19RQECh. 18 - Prob. 20RQECh. 18 - Prob. 21RQECh. 18 - Prob. 22RQECh. 18 - Prob. 23RQECh. 18 - Prob. 24RQECh. 18 - Prob. 25RQECh. 18 - Prob. 26RQECh. 18 - Write two different code segments that may be used...Ch. 18 - Prob. 28RQECh. 18 - Prob. 29RQECh. 18 - Prob. 30RQECh. 18 - Prob. 31RQECh. 18 - Prob. 32RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Dynamic String Stack Design a class that stores...Ch. 18 - Prob. 7PCCh. 18 - Prob. 8PCCh. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Inventory Bin Stack Design an inventory class that...Ch. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- [5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}. 2) [12 marks] Consider the following languages over the alphabet ={a ,b}, (i) The language of all words that begin and end an a (ii) The language where every a in a word is immediately followed by at least one b. (a) Express each as a Regular Expression (b) Draw an FA for each language (c) For Language (i), draw a TG using at most 3 states (d) For Language (ii), construct a CFG.arrow_forwardQuestion 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forwardGenerate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.arrow_forward
- Can I get help with this case please, thank youarrow_forwardI need help to solve the following, thank youarrow_forwardreminder it an exercice not a grading work GETTING STARTED Open the file SC_EX19_EOM2-1_FirstLastNamexlsx, available for download from the SAM website. Save the file as SC_EX19_EOM2-1_FirstLastNamexlsx by changing the “1” to a “2”. If you do not see the .xlsx file extension in the Save As dialog box, do not type it. The program will add the file extension for you automatically. With the file SC_EX19_EOM2-1_FirstLastNamexlsx still open, ensure that your first and last name is displayed in cell B6 of the Documentation sheet. If cell B6 does not display your name, delete the file and download a new copy from the SAM website. Brad Kauffman is the senior director of projects for Rivera Engineering in Miami, Florida. The company performs engineering projects for public utilities and energy companies. Brad has started to create an Excel workbook to track estimated and actual hours and billing amounts for each project. He asks you to format the workbook to make the…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
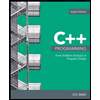
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
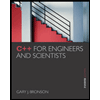
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
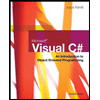
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
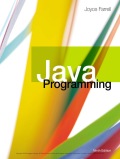
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
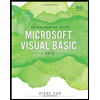
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning