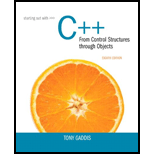
Starting Out with C++ from Control Structures to Objects (8th Edition)
8th Edition
ISBN: 9780133769395
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 18, Problem 20RQE
Program Description Answer
“queue” and “deque” are the two queue-like data structures that are provided by STL.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
C++ Code for a QueueThe program should features a Queue class with insert(), remove(), peek(),isFull(), isEmpty(), and size() member functions.The main() program creates a queue of five cells, inserts four items, removes threeitems, and inserts four more. The sixth insertion invokes the wraparound feature. All the items are then removed and displayed. The output looks like this:40 50 60 70 80
// FILE: DPQueue.h// CLASS PROVIDED: p_queue (priority queue ADT)//// TYPEDEFS and MEMBER CONSTANTS for the p_queue class:// typedef _____ value_type// p_queue::value_type is the data type of the items in// the p_queue. It may be any of the C++ built-in types// (int, char, etc.), or a class with a default constructor, a// copy constructor, an assignment operator, and a less-than// operator forming a strict weak ordering.//// typedef _____ size_type// p_queue::size_type is the data type considered best-suited// for any variable meant for counting and sizing (as well as// array-indexing) purposes; e.g.: it is the data type for a// variable representing how many items are in the p_queue.// It is also the data type of the priority associated with// each item in the p_queue//// static const size_type DEFAULT_CAPACITY = _____// p_queue::DEFAULT_CAPACITY is the default initial capacity of a// p_queue that is created by the default…
// FILE: DPQueue.h// CLASS PROVIDED: p_queue (priority queue ADT)//// TYPEDEFS and MEMBER CONSTANTS for the p_queue class:// typedef _____ value_type// p_queue::value_type is the data type of the items in// the p_queue. It may be any of the C++ built-in types// (int, char, etc.), or a class with a default constructor, a// copy constructor, an assignment operator, and a less-than// operator forming a strict weak ordering.//// typedef _____ size_type// p_queue::size_type is the data type considered best-suited// for any variable meant for counting and sizing (as well as// array-indexing) purposes; e.g.: it is the data type for a// variable representing how many items are in the p_queue.// It is also the data type of the priority associated with// each item in the p_queue//// static const size_type DEFAULT_CAPACITY = _____// p_queue::DEFAULT_CAPACITY is the default initial capacity of a// p_queue that is created by the default…
Chapter 18 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Ch. 18.3 - Describe what LIFO means.Ch. 18.3 - What is the difference between static and dynamic...Ch. 18.3 - What are the two primary stack operations?...Ch. 18.3 - What STL types does the STL stack container adapt?Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - What is the difference between a static stack and...Ch. 18 - Prob. 4RQECh. 18 - Prob. 5RQECh. 18 - The STL stack is considered a container adapter....
Ch. 18 - What types may the STL stack be based on? By...Ch. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - The STL stack container is an adapter for the...Ch. 18 - Prob. 18RQECh. 18 - Prob. 19RQECh. 18 - Prob. 20RQECh. 18 - Prob. 21RQECh. 18 - Prob. 22RQECh. 18 - Prob. 23RQECh. 18 - Prob. 24RQECh. 18 - Prob. 25RQECh. 18 - Prob. 26RQECh. 18 - Write two different code segments that may be used...Ch. 18 - Prob. 28RQECh. 18 - Prob. 29RQECh. 18 - Prob. 30RQECh. 18 - Prob. 31RQECh. 18 - Prob. 32RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Dynamic String Stack Design a class that stores...Ch. 18 - Prob. 7PCCh. 18 - Prob. 8PCCh. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Inventory Bin Stack Design an inventory class that...Ch. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Similar questions
- SKELETON CODE IS PROVIDED ALONG WITH C AND H FILES. #include <stdio.h> #include <stdlib.h> #include <string.h> #include <stdbool.h> #include "node.h" #include "stack_functions.h" #define NUM_VERTICES 10 /** This function takes a pointer to the adjacency matrix of a Graph and the size of this matrix as arguments and prints the matrix */ void print_graph(int * graph, int size); /** This function takes a pointer to the adjacency matrix of a Graph, the size of this matrix, the source and dest node numbers along with the weight or cost of the edge and fills the adjacency matrix accordingly. */ void add_edge(int * graph, int size, int src, int dst, int cost); /** This function takes a pointer to the adjacency matrix of a graph, the size of this matrix, source and destination vertex numbers as inputs and prints out the path from the source vertex to the destination vertex. It also prints the total cost of this…arrow_forwardIn C++ Pleasearrow_forwardIn a stack, items are added and deleted only at one end, referred to as the __________of the stack.arrow_forward
- Stack: Stacks are a type of container with LIFO (Last In First Out) type of working, where a new element is added at one end and (top) an element is removed from that end only. Your Stack should not be of the fixed sized. It should be able to grow itself. So using the class made in task 1, make a class named as Stack, having following additional functionalities: bool empty() : Returns whether the Stack is empty or not. Time Complexity should be: O(1) bool full() : Returns whether the Stack is full or not. Time Complexity should be: O(1)int size() : Returns the current size of the Stack. Time Complexity should be: O(1)Type top () : Returns the last element of the Stack. Time Complexity should be: O(1) void push(Type) : Adds the element of type Type at the top of the stack. Time Complexity should be: O(1) Type pop() : Deletes the top most element of the stack and returns it. Time Complexity should be: O(1) Write non-parameterized constructor for the above class. Write Copy…arrow_forwardCreate a queue, size of queue will be dependent on the user. Insert the numbers in the queue till the queue reaches the size. Create a menu and perform the following function on that queue. This is all done by using oop in C++. Enqueue: Add an element to the end of the queue Dequeue: Remove an element from the front of the queue IsEmpty: Check if the queue is empty IsFull: Check if the queue is full Peek: Get the value of the front of the queue without removing itarrow_forwardRead this: Complete the code in Visual Studio using C++ Programming Language with 1 file or clear answer!! - Give me the answer in Visual Studio so I can copy*** - Separate the files such as .cpp, .h, or .main if any! Develop a C++ "doubly" linked list class of your own that can hold a series of signed shorts Develop the following functionality: Develop a linked list node struct/class You can use it as a subclass like in the book (Class contained inside a class) You can use it as its own separate class Your choice Maintain a private pointer to a node class pointer (head) Constructor Initialize head pointer to null Destructor Make sure to properly delete every node in your linked list push_front(value) Insert the value at the front of the linked list pop_front() Remove the node at the front of the linked list If empty, this is a no operation operator << Display the contents of the linked list just like you would print a character…arrow_forward
- Descending priority queue can be implemented using________.arrow_forwardin c++ Write a function that takes a queue with 20 integer values and deletes the elements thatare between 15 and 20. In other words, only the elements less than 15 or greater than 20remain in the queue. This means that you need to have an auxiliary queue to store theelements between 15 and 20 and restore them back to the original queue.arrow_forwarddata structures-java language quickly plsarrow_forward
- 3- Write a program that randomly generates 10 numbers (between 1 and 8), inserts into queue and then finds how many distinct elements exist in the queue. Example 1: Example 2: Queue: 2 40 3 3 2 18 4 18 18 3 Queue: 1 1 4 33 16 16 4 16 4 Output: 5 Output: 4 Notes: • You must use ONLY queue data structure. Don't use other different data structures like string or normal (pure) array or stack or array list. • Don't write any other method in the Qeueu class. All methods must be written in the main program.arrow_forwardfor c++ thank you Error Testing The DynIntStack and Dyn IntQueue classes shown in this chapter are abstract data types using a dynamic stack and dynamic queue, respectively. The classes do not cur- rently test for memory allocation errors. Modify the classes so they determine whether new nodes cannot be created by handling the bad_alloc exception. here is the extention.h file please use it #ifndef DYNINTQUEUE_H #define DYNINTQUEUE_H class DynIntQueue { private: // Structure for the queue nodes struct QueueNode { int value; // Value in a node QueueNode *next; // Pointer to the next node }; QueueNode *front; // The front of the queue QueueNode *rear; // The rear of the queue int numItems; // Number of items in the queue public: // Constructor DynIntQueue(); // Destructor ~DynIntQueue(); // Queue operations void enqueue(int); void dequeue(int &); bool isEmpty() const; bool isFull() const; void…arrow_forwardRead this: Complete the code in Visual Studio using C++ Programming Language - Give me the answer in Visual Studio so I can copy*** - Separate the files such as .cpp, .h, or .main if any! Develop a C++ "doubly" linked list class of your own that can hold a series of signed shorts Develop the following functionality: Develop a linked list node struct/class You can use it as a subclass like in the book (Class contained inside a class) You can use it as its own separate class Your choice Maintain a private pointer to a node class pointer (head) Constructor Initialize head pointer to null Destructor Make sure to properly delete every node in your linked list push_front(value) Insert the value at the front of the linked list pop_front() Remove the node at the front of the linked list If empty, this is a no operation operator << Display the contents of the linked list just like you would print a character string operator [] Treat like…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
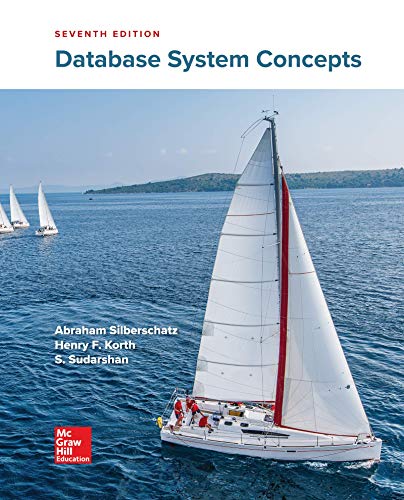
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
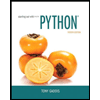
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
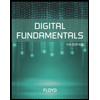
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
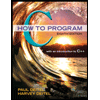
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
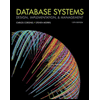
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
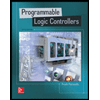
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education