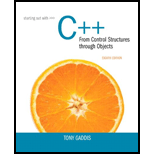
Starting Out with C++ from Control Structures to Objects (8th Edition)
8th Edition
ISBN: 9780133769395
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 18, Problem 5PC
Program Plan Intro
Error Testing
Dynamic Stack:
Program Plan:
Main.cpp:
- Include required header files.
- Inside the “main ()” function,
- Create an object named “dstack” for stack.
- Declare a variable named “popElem”.
- Push 3 elements inside the stack using the function “push ()”.
- Pop 3 elements from the stack using the function “pop ()”.
DynIntStack.h:
- Include required header files.
- Create a template.
- Declare a class named “DynIntStack”. Inside the class
- Inside the “private” access specifier,
- Give structure declaration for the stack
- Create an object for the template
- Create a stack pointer name “next”.
- Create a stack pointer name “top”
- Give structure declaration for the stack
- Inside the “public” access specifier,
- Give a declaration for a constructor.
- Assign null to the top node.
- Give function declaration for “push ()”, “pop ()”,and “isEmpty ()”.
- Give a declaration for a constructor.
- Inside the “private” access specifier,
- Give the class template.
- Define function definition for “push ()”.
- Assign null to the new node.
- Dynamically allocate memory for new node,
- Assign “num” to the value of new node.
- Check if the stack is empty using the function “isEmpty ()”
- If the condition is true then assign new node as the top and make the next node as null.
- If the condition is not true then, assign top node to the next of new node and assign new node as the top.
- Give the class template.
- Define function definition for “pop ()”.
- Assign null to the temp node.
- Check if the stack is empty using the function “is_Empty ()”
- If the condition is true then print “The stack is empty”.
- If the condition is not true then,
- Assign top value to the variable “num”.
- Link top of next node to temp node.
- Delete the top most node and make temp as the top node.
- Define function definition for “isEmpty ()”.
- Assign Boolean value to the variable
- Check if the top node is null
- Assign true to “status”.
- Return the status
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Stack:
Stacks are a type of container with LIFO (Last In First Out) type of working, where a new element is added at one end and (top) an element is removed from that end only. Your Stack should not be of the fixed sized. It should be able to grow itself. So using the class made in task 1, make a class named as Stack, having following additional functionalities:
bool empty() : Returns whether the Stack is empty or not. Time Complexity should be: O(1)
bool full() : Returns whether the Stack is full or not. Time Complexity should be: O(1)int size() : Returns the current size of the Stack. Time Complexity should be: O(1)Type top () : Returns the last element of the Stack. Time Complexity should be: O(1)
void push(Type) : Adds the element of type Type at the top of the stack. Time Complexity should be: O(1)
Type pop() : Deletes the top most element of the stack and returns it. Time Complexity should be: O(1)
Write non-parameterized constructor for the above class.
Write Copy…
In C++ Please
for c++ thank you
Error Testing
The DynIntStack and Dyn IntQueue classes shown in this chapter are abstract data types using a dynamic stack and dynamic queue, respectively. The classes do not cur- rently test for memory allocation errors. Modify the classes so they determine whether new nodes cannot be created by handling the bad_alloc exception.
here is the extention.h file please use it
#ifndef DYNINTQUEUE_H
#define DYNINTQUEUE_H
class DynIntQueue
{
private:
// Structure for the queue nodes
struct QueueNode
{
int value; // Value in a node
QueueNode *next; // Pointer to the next node
};
QueueNode *front; // The front of the queue
QueueNode *rear; // The rear of the queue
int numItems; // Number of items in the queue
public:
// Constructor
DynIntQueue();
// Destructor
~DynIntQueue();
// Queue operations
void enqueue(int);
void dequeue(int &);
bool isEmpty() const;
bool isFull() const;
void…
Chapter 18 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Ch. 18.3 - Describe what LIFO means.Ch. 18.3 - What is the difference between static and dynamic...Ch. 18.3 - What are the two primary stack operations?...Ch. 18.3 - What STL types does the STL stack container adapt?Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - What is the difference between a static stack and...Ch. 18 - Prob. 4RQECh. 18 - Prob. 5RQECh. 18 - The STL stack is considered a container adapter....
Ch. 18 - What types may the STL stack be based on? By...Ch. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - The STL stack container is an adapter for the...Ch. 18 - Prob. 18RQECh. 18 - Prob. 19RQECh. 18 - Prob. 20RQECh. 18 - Prob. 21RQECh. 18 - Prob. 22RQECh. 18 - Prob. 23RQECh. 18 - Prob. 24RQECh. 18 - Prob. 25RQECh. 18 - Prob. 26RQECh. 18 - Write two different code segments that may be used...Ch. 18 - Prob. 28RQECh. 18 - Prob. 29RQECh. 18 - Prob. 30RQECh. 18 - Prob. 31RQECh. 18 - Prob. 32RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Dynamic String Stack Design a class that stores...Ch. 18 - Prob. 7PCCh. 18 - Prob. 8PCCh. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Inventory Bin Stack Design an inventory class that...Ch. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Similar questions
- 1 Implement a Queue Data Structure specifically to store integer data using a Singly Linked List. 2 The data members should be private. 3 You need to implement the following public functions: 4 1. Constructor: 5 It initialises the data members as required. 6 7 8 2. enqueue(data) : This function should take one argument of type integer. It enqueues the element into the queue and returns nothing. 3. dequeue(): It dequeues/removes the element from the front of the queue and in turn, returns the element being dequeued or removed. In case the queue is empty, it r 4. front (): 10 11 It returns the element being kept at the front of the queue. In case the queue is empty, it returns -1. 12 5. getSize(): 13 It returns the size of the queue at any given instance of time. 14 6. 1sEmpty(): 15 It returns a boolean value indicating whether the queue is empty or not. 16 Operations Performed on the Stack: 17 Query-1 (Denoted by an integer 1): Enqueues an integer data to the queue. 18 19 Query-2…arrow_forwardCourse: Data Structure and Algorithms Language: C++ Question is well explained Question #2Implement a class for Circular Doubly Linked List (with a dummy header node) which stores integers in unsorted order. Your class definitions should look like as shown below: class CDLinkedList;class DNode {friend class CDLinkedList;private int data;private DNode next;private DNode prev;};class CDLinkedList {private:DNode head; // Dummy header nodepublic CDLinkedList(); // Default constructorpublic bool insert (int val); public bool removeSecondLastValue (); public void findMiddleValue(); public void display(); };arrow_forwardc++arrow_forward
- Course: Data Structure and Algorithims Language: Java Kindly make the program in 2 hours. Task is well explained. You have to make the proogram properly in Java: Restriction: Prototype cannot be change you have to make program by using given prototype. TAsk: Create a class Node having two data members int data; Node next; Write the parametrized constructor of the class Node which contain one parameter int value assign this value to data and assign next to null Create class LinkList having one data members of type Node. Node head Write the following function in the LinkList class publicvoidinsertAtLast(int data);//this function add node at the end of the list publicvoid insertAthead(int data);//this function add node at the head of the list publicvoid deleteNode(int key);//this function find a node containing "key" and delete it publicvoid printLinkList();//this function print all the values in the Linklist public LinkListmergeList(LinkList l1,LinkList l2);// this function…arrow_forwardC++ Code for a QueueThe program should features a Queue class with insert(), remove(), peek(),isFull(), isEmpty(), and size() member functions.The main() program creates a queue of five cells, inserts four items, removes threeitems, and inserts four more. The sixth insertion invokes the wraparound feature. All the items are then removed and displayed. The output looks like this:40 50 60 70 80arrow_forwardComputer science JAVA programming languagearrow_forward
- Create a node class named LinkedNodes that uses up to 4 dynamic pointers to connect it to the other 8 nodes in the structure (a total of 9). The data in each node will be a unique integer number from 1 through 9. Include in your class definition these functions at a minimum: getVal(), setVal(), getNext(), setNext(), getPrev(), setPrev(), constructor(s) and destructor. Write a simple program to load these nodes into a linked structure. Do not use an array, the pointers will do that for you. You should be able to reach any node from any other node in the structure by traversing a maximum of 2 nodes from the current one. The output from your program should report the traversals between all nodes in the structure, starting with the node whose value is one. (See the example below) Node 1 2 3 4 5 6 7 8 9 -> Traverses to Node 1 Node 2 1->2 2->6->1 3->6->1 3->7->2 4->6->1 4->7->2 4->8->3 5->6->1 5->7->2 5->8->3 6->1->2 6->1->3 7->1->3 8->3 6->1 7->1 8->1 9->1 Node 3 1->3 2->3 7->2 8->2 9->2…arrow_forwardIn c++ Also add comments explaining each linearrow_forwardc++ Write a client function that returns the back of a queue while leaving the queue unchanged. This function can call any of the methods of the ADT queue. It can also create new queues. The return type is ITemType, and it accepts a queue as a parameter.arrow_forward
- Reference-based Linked Lists: Select all of the following statements that are true. As a singly linked list's node references both its predecessor and its successor, it is easily possible to traverse such a list in both directions. According to the terminology introduced in class, the head reference variable in a singly linked list object references the list's first node. According to the terminology introduced in class, in a doubly linked list, each node references both the head and tail node. In a double-ended singly linked list, the tail reference variable provides access to the entire list. In a circular linked list, the last node references the first node.arrow_forwardStack: Stacks are a type of container with LIFO (Last In First Out) type of working, where a new element is added at one end and (top) an element is removed from that end only. Your Stack should not be of the fixed sized. It should be able to grow itself. bool empty() : Returns whether the Stack is empty or not. Time Complexity should be: O(1) bool full() : Returns whether the Stack is full or not. Time Complexity should be: O(1)int size() : Returns the current size of the Stack. Time Complexity should be: O(1)Type top () : Returns the last element of the Stack. Time Complexity should be: O(1) void push(Type) : Adds the element of type Type at the top of the stack. Time Complexity should be: O(1) Type pop() : Deletes the top most element of the stack and returns it. Time Complexity should be: O(1) Write non-parameterized constructor for the above class. Write Copy constructor for the above class. Write Destructor for the above class. Now write a global function show stack which…arrow_forwarddata structures-java language quickly plsarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
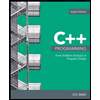
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning