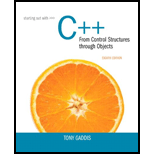
Starting Out with C++ from Control Structures to Objects (8th Edition)
8th Edition
ISBN: 9780133769395
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 18, Problem 10PC
Program Plan Intro
File Filter
Program Plan:
Main.cpp:
- Include required header files.
- Inside “main ()” function,
- Create an object “file1” for input file stream.
- Create an object “ofile” for output file stream.
- Declare a class template
- Declare character variables named “popChar”, and “ch”.
- Till the end of file,
- Convert each character and Enqueue the character using the function “enqueue ()”.
- Close “file1”.
- Until the stack gets empty,
- Pop the character stack and write it on output file “ofile” using the function “dequeue ()”.
- Close the output file“ofile”.
- Open the output file “ofile”.
- Till the end of file,
- Get a character from queue and print it on the console screen.
DynQueue.h:
- Include required header files.
- Create template class
- Declare a class named “DynQueue”. Inside the class,
- Inside the “private” access specifier,
- Create a structure named “QueueNode”.
- Create an object for the template
- Create a pointer named “next”.
- Create two pointers named “front” and “rear”.
- Declare a variable.
- Create a structure named “QueueNode”.
- Inside “public” access specifier,
- Declare constructor and destructor.
- Declare the functions “enqueue ()”, “dequeue ()”, “isEmpty ()”, “isFull ()”, and “clear ()”.
- Inside the “private” access specifier,
- Declare template class.
- Give definition for the constructor.
- Assign the values.
- Declare template class.
- Give definition for the destructor.
- Call the function “clear ()”.
- Declare template class.
- Give function definition for “enqueue ()”.
- Make the pointer “newNode” as null.
- Assign “num” to newNode->value.
- Make newNode->next as null.
- Check whether the queue is empty using “isEmpty ()” function.
- If the condition is true then, assign newNode to “front” and “rear”.
- If the condition is not true then,
- Assign newNode to rear->next
- Assign newNode to “rear”.
- Increment the variable “numItems”.
- Declare template class.
- Give function definition for “dequeue ()”.
- Assign temp pointer as null.
- Check if the queue is empty using “isEmpty ()” function.
- If the condition is true then print “The queue is empty”.
- If the condition is not true then,
- Assign the value of front to the variable “num”.
- Make front->next as “temp”.
- Delete the front value
- Make temp as front.
- Decrement the variable “numItems”.
- Declare template class.
- Give function definition for “isEmpty ()”.
- Assign “true” to a Boolean variable
- Check if “numItems” is true.
- If the condition is true then assign “false” to the variable.
- Return the Boolean variable.
- Declare template class.
- Give function definition for “clear ()”.
- Create an object for template.
- Dequeue values from queue till the queue becomes empty using “while” condition.
- Create an object for template.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Describe three (3) Multiplexing techniques common for fiber optic links
Could you help me to know features of the following concepts:
- commercial CA
- memory integrity
- WMI filter
Briefly describe the issues involved in using ATM technology in Local Area Networks
Chapter 18 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Ch. 18.3 - Describe what LIFO means.Ch. 18.3 - What is the difference between static and dynamic...Ch. 18.3 - What are the two primary stack operations?...Ch. 18.3 - What STL types does the STL stack container adapt?Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - What is the difference between a static stack and...Ch. 18 - Prob. 4RQECh. 18 - Prob. 5RQECh. 18 - The STL stack is considered a container adapter....
Ch. 18 - What types may the STL stack be based on? By...Ch. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - The STL stack container is an adapter for the...Ch. 18 - Prob. 18RQECh. 18 - Prob. 19RQECh. 18 - Prob. 20RQECh. 18 - Prob. 21RQECh. 18 - Prob. 22RQECh. 18 - Prob. 23RQECh. 18 - Prob. 24RQECh. 18 - Prob. 25RQECh. 18 - Prob. 26RQECh. 18 - Write two different code segments that may be used...Ch. 18 - Prob. 28RQECh. 18 - Prob. 29RQECh. 18 - Prob. 30RQECh. 18 - Prob. 31RQECh. 18 - Prob. 32RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Dynamic String Stack Design a class that stores...Ch. 18 - Prob. 7PCCh. 18 - Prob. 8PCCh. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Inventory Bin Stack Design an inventory class that...Ch. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
- a) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forwardusing r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
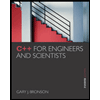
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
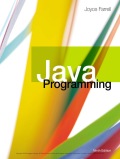
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
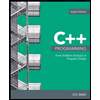
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
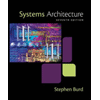
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
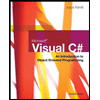
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,