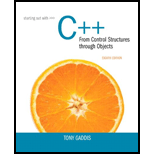
Starting Out with C++ from Control Structures to Objects (8th Edition)
8th Edition
ISBN: 9780133769395
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 18, Problem 1PC
Program Plan Intro
Static Stack Template
Program Plan:
Main.cpp:
- Include required header files.
- Inside the “main ()” function,
- Create an object named “stck” for stack.
- Declare a variable named “popElem”.
- Push 5 elements inside the stack using the function “push_Elem ()”.
- Pop 5 elements from the stack using the function “pop_Elem ()”.
Stack.h:
- Include required header files.
- Create a template.
- Declare a class named “Stack”. Inside the class
- Inside the “private” access specifier,
- Create an object for the template
- Declare the variables “stackSize” and “top_Elem”.
- Inside the “public” access specifier,
- Give a declaration for an overloaded constructor.
- Give function declaration for “push_Elem ()”, “pop_Elem ()”, “is_Full ()”, and “is_Empty ()”.
- Inside the “private” access specifier,
- Give function definition for the overloaded constructor.
- Create stack size
- Assign the value to the “stackSize”.
- Assign -1 to the variable “top_Elem”
- Give function definition for “push_Elem ()”.
- Check if the stack is full using the function “is_Full ()”
- If the condition is true then print “The stack is full”.
- If the condition is not true then,
- Increment the variable “top_Elem”.
- Assign the element to the top position.
- Check if the stack is full using the function “is_Full ()”
- Give function definition for “pop_Elem ()”.
- Check if the stack is empty using the function “is_Empty ()”
- If the condition is true then print “The stack is empty”.
- If the condition is not true then,
- Assign the element to the variable “num”.
- Decrement the variable “top_Elem”.
- Check if the stack is empty using the function “is_Empty ()”
- Give function definition for “is_Full ()”.
- Assign Boolean value to the variable
- Check if the top and the stack size is same
- Assign true to “status”.
- Return the status
- Give function definition for “is_Empty ()”.
- Assign Boolean value to the variable
- Check if the top is equal to -1.
- Assign true to “status”.
- Return the status
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
c++ data structures ADT Unsorted List
The specifications for the Unsorted List ADT states that the item to be deleted is in the list.
Rewrite the specifications for DeleteItem so that the list is unchanged if the item to be deleted is not in the list.
Rewrite the specifications for DeleteItem so that all copies of the item to be deleted are removed if they exist
Solve in C Program
Implement a program using stack and queue operations
Do in C Program
Implement using stack and queue operations
Chapter 18 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Ch. 18.3 - Describe what LIFO means.Ch. 18.3 - What is the difference between static and dynamic...Ch. 18.3 - What are the two primary stack operations?...Ch. 18.3 - What STL types does the STL stack container adapt?Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - What is the difference between a static stack and...Ch. 18 - Prob. 4RQECh. 18 - Prob. 5RQECh. 18 - The STL stack is considered a container adapter....
Ch. 18 - What types may the STL stack be based on? By...Ch. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - The STL stack container is an adapter for the...Ch. 18 - Prob. 18RQECh. 18 - Prob. 19RQECh. 18 - Prob. 20RQECh. 18 - Prob. 21RQECh. 18 - Prob. 22RQECh. 18 - Prob. 23RQECh. 18 - Prob. 24RQECh. 18 - Prob. 25RQECh. 18 - Prob. 26RQECh. 18 - Write two different code segments that may be used...Ch. 18 - Prob. 28RQECh. 18 - Prob. 29RQECh. 18 - Prob. 30RQECh. 18 - Prob. 31RQECh. 18 - Prob. 32RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Dynamic String Stack Design a class that stores...Ch. 18 - Prob. 7PCCh. 18 - Prob. 8PCCh. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Inventory Bin Stack Design an inventory class that...Ch. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Similar questions
- C++ Question You need to write a class called LinkedList that implements the following List operations: public void add(int index, Object item); // adds an item to the list at the given index, that index may be at start, end or after or before the // specific element 2.public void remove(int index); // removes the item from the list that has the given index 3.public void remove(Object item); // finds the item from list and removes that item from the list 4.public List duplicate(); // creates a duplicate of the list // postcondition: returns a copy of the linked list 5.public List duplicateReversed(); // creates a duplicate of the list with the nodes in reverse order // postcondition: returns a copy of the linked list with the nodes in 6.public List ReverseDisplay(); //print list in reverse order 7.public Delete_Smallest(); // Delete smallest element from linked list 8.public List Delete_duplicate(); // Delete duplicate elements from a given linked list.Retain the…arrow_forwardC++ ProgrammingActivity: Deque Linked List Explain the flow of the main code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow. SEE ATTACHED PHOTO FOR THE PROBLEM INSTRUCTIONS int main(int argc, char** argv) { DLLDeque* deque = new DLLDeque(); int test; cin >> test; //Declarations int tempnum; int num; int sum; int count; bool addfirst = false; bool addlast = false; bool remfirst = false; bool remlast = false; switch (test) { case 0: //Implementation do{ cin >> num; if(num == 0){ break; } //Reset tempnum = num; count = 0; sum = 0; addfirst = false; addlast = false; remfirst = false; remlast =…arrow_forwarddata structers C programming languagearrow_forward
- Please use C++arrow_forwardA "generic" data structure cannot use a primitive type as its generic type. O True Falsearrow_forwardLINKED LIST IMPLEMENTATION Linked list Write a C++ program to implement insertion, deletion, and display operations in a Linked List Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement.The class Node has the following member variable Datatype Variable Usage int data to store data Node* next to store the next node Define the following public member functions in the class LinkedList. Member function Function description void insertNode(int value) This function inserts the data into the linked list at the end void deleteNode(int value) This function deletes the node from the linked list void display() This function is used to display the nodes in the linked list In the main() function, read inputs and call the functions of the LinkedList class based on the inputs. Note: If the Linked list is empty while…arrow_forward
- Multiple choice in data structures Assume the function: void F(stack<T> &S){ } and we send a stack S to the function F, as a result of it Select one: a. Both (copy constructor and destructor) should not be called b. Destructor should be called c. Copy constructor should be called d. Both (copy constructor and destructor) should be calledarrow_forwardC++ Program #include <iostream>#include <cstdlib>#include <ctime>using namespace std; int getData() { return (rand() % 100);} class Node {public: int data; Node* next;}; class LinkedList{public: LinkedList() { // constructor head = NULL; } ~LinkedList() {}; // destructor void addNode(int val); void addNodeSorted(int val); void displayWithCount(); int size(); void deleteAllNodes(); bool exists(int val);private: Node* head;}; // function to check data exist in a listbool LinkedList::exists(int val){ if (head == NULL) { return false; } else { Node* temp = head; while (temp != NULL) { if(temp->data == val){ return true; } temp = temp->next; } } return false;} // function to delete all data in a listvoid LinkedList::deleteAllNodes(){ if (head == NULL) { cout << "List is empty, No need to delete…arrow_forwardWrite JAVA code General Problem Description: It is desired to develop a directory application based on the use of a double-linked list data structure, in which students are kept in order according to their student number. In this context, write the Java codes that will meet the requirements given in detail below. Requirements: Create a class named Student to represent students. In the Student class; student number, name and surname and phone numbers for communication are kept. Student's multiple phones number (multiple mobile phones, home phones, etc.) so phone numbers information will be stored in an “ArrayList”. In the Student class; parameterless, taking all parameters and It is sufficient to have 3 constructor methods, including a copy constructor, get/set methods and toString.arrow_forward
- C++ Queue LinkedList, Refer to the codes I've made as a guide and add functions where it can add/insert/remove function to get the output of the program, refer to the image as an example of input & output. Put a comment or explain how the program works/flow.arrow_forwardWhat is meant by garbage collectionarrow_forwardC++ data structures Write functions fill and print. 1. function fill in 2 queue with random intgers " rand%100 ". 2. function to print the random intgers from the 2 queue we filled. 3. store the common items in the 2 queues in an additional queue like " queue number 3" note: solve it without using different data structure such as array.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
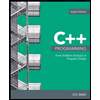
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning