You need to write a class called LinkedList that implements the following List operations: public void add(int index, Object item); // adds an item to the list at the given index, that index may be at start, end or after or before the // specific element 2.public void remove(int index); // removes the item from the list that has the given index 3.public void remove(Object item); // finds the item from list and removes that item from the list 4.public List duplicate(); // creates a duplicate of the list // postcondition: returns a copy of the linked list 5.public List duplicateReversed(); // creates a duplicate of the list with the nodes in reverse order // postcondition: returns a copy of the linked list with the nodes in 6.public List ReverseDisplay(); //print list in reverse order 7.public Delete_Smallest(); // Delete smallest element from linked list 8.public List Delete_duplicate(); // Delete duplicate elements from a given linked list.Retain the earliest entries. 9 Make a function that adds a linked list to itself at the end. Input: 4 -> 2 -> 1 -> NULL Output: 4 -> 2 -> 1 -> 4 -> 2 -> 1 -> NULL note : code should work on Visual studio 2017 and provide screenshot of output
C++ Question
You need to write a class called LinkedList that implements the following List operations:
- public void add(int index, Object item);
// adds an item to the list at the given index, that index may be at start, end or after or before the
// specific element
2.public void remove(int index);
// removes the item from the list that has the given index
3.public void remove(Object item);
// finds the item from list and removes that item from the list
4.public List duplicate();
// creates a duplicate of the list
// postcondition: returns a copy of the linked list
5.public List duplicateReversed();
// creates a duplicate of the list with the nodes in reverse order
// postcondition: returns a copy of the linked list with the nodes in
6.public List ReverseDisplay();
//print list in reverse order
7.public Delete_Smallest();
// Delete smallest element from linked list
8.public List Delete_duplicate();
// Delete duplicate elements from a given linked list.Retain the earliest entries.
9 Make a function that adds a linked list to itself at the end.
Input:
4 -> 2 -> 1 -> NULL
Output:
4 -> 2 -> 1 -> 4 -> 2 -> 1 -> NULL
note : code should work on Visual studio 2017 and provide screenshot of output

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

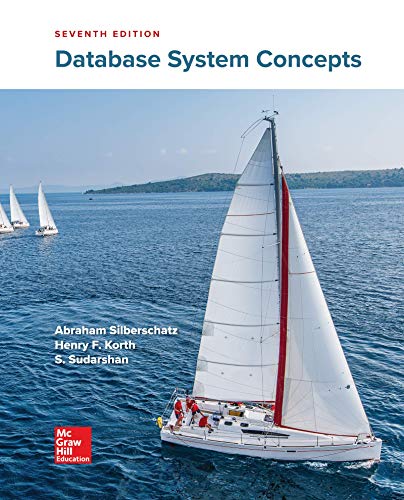
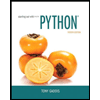
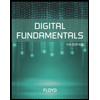
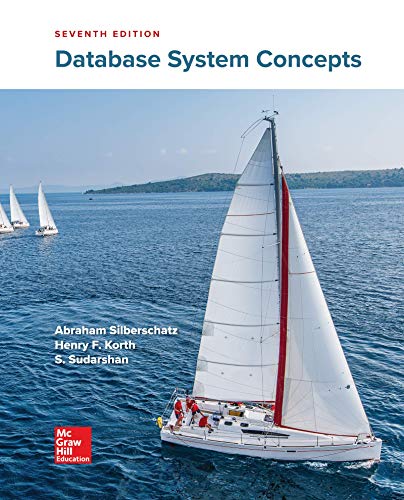
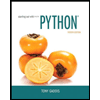
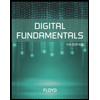
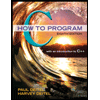
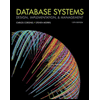
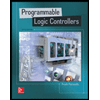