* An int called WEAPON_TYPE indicating their weapon type. This field should be given the value of 3, representing the swords. * An int called BASE ATTACK DAMAGE indicating their base attack damage. This class also has the following public method: * A constructor that takes as input a Tile which represents the position of the warrior in the game. The constructor uses the input to create a warrior with the given position, BASE HEALTH, BASE_COST, WEAPON_TYPE and BASE ATTACK DAMAGE. Write a class Axebringer derived from the Warrior class. The Axebringer class rep- resents warriors who wield axes. These warriors are less precise, but when their attacks land, they inflict high damage. They are heavy units that are difficult to move. This class has the following public static fields: * An double called BASE HEALTH indicating the base health of an Axebringer. * An int called BASE_COST indicating their base training cost. * An int called WEAPON_TYPE indicating their weapon type. This field should be given the value of 2, representing the axes. * An int called BASE ATTACK DAMAGE indicating their base attack damage. The Axebringer class also has the following public method: * A constructor that takes as input a Tile which represents the position of the warrior in the game. The constructor uses the input to create a warrior with the given position, BASE HEALTH, BASE_COST, WEAPON_TYPE and BASE ATTACK DAMAGE. STEP 1: The skeleton Let's start by creating a skeleton for some of the classes you will need. • Write a class called Tile. You can think of a tile as a square on the board on which the game will be played. We will come back to this class later. For the moment you can leave it empty while you work on creating classes that represents characters in the game. • Write an abstract class Fighter which has the following private fields: - A Tile field named position, representing the fighter's position in the game. A double field named health, representing the fighter's health points (HP). An int field named weaponType, representing the type of weapon the fighter is using. This value is used to rank different weapon types: higher values indicate higher weapon ranks. -An int field named attackDamage, representing the fighter's attack power. The class must also have the following public methods: 3 A constructor that takes as input a Tile indicating the position of the fighter, a double indicating its hp, an int indicating its weapon type, and an int indicating its attack damage. The constructor uses its inputs to initialize the corresponding fields. Please do not make a copy of the Tile object. A final getPosition() method to retrieve the position of this fighter. A final getHealth() method to retrieve the health points of this fighter. - A final getWeaponType() method to retrieve the weapon type of this fighter. - A final getAttackDamage () method to retrieve the attack damage of this fighter. A setPosition() method that takes a Tile as input and updates the value stored in the appropriate field. This method should not create a copy of the input Tile and should not return anything. All of the following must be subclasses of the Fighter class: Write a class Monster derived from the Fighter class. The Monster class has the following public method: * A constructor that takes as input a Tile indicating the position of the fighter, a double indicating its hp, an int indicating its weapon type, and an int indicating its attack damage. The constructor uses the inputs to create a Monster with the above characteristics. Write an abstract class Warrior derived from the Fighter class. The Warrior class has the following private field: * An int field named requiredSkillPoints, representing the number of skill points required to train this warrior. A player can only deploy a warrior if they have accumulated the necessary skill points. Different warriors require different amounts of skill points. The Warrior class also has the following public methods: A constructor that takes as input a Tile indicating the position of the fighter, a double indicating its hp, an int indicating its weapon type, an int indicating its attack damage and an int indicating its cost in skill points respectively. The constructor uses the inputs to create a Warrior with the above characteristics. * A getTraining Cost () method that returns the amount of skill points required to deploy this warrior. Write a class Bladesworn derived from the Warrior class. The Bladesworn class rep- resents warriors who wield swords as their weapons. These warriors are precise in their attacks and highly agile, making them effective at defending themselves and possibly advancing forward. This class has the following public static fields: * An double called BASE HEALTH indicating the base health of Bladesworns. * An int called BASE COST indicating their base training cost.
* An int called WEAPON_TYPE indicating their weapon type. This field should be given the value of 3, representing the swords. * An int called BASE ATTACK DAMAGE indicating their base attack damage. This class also has the following public method: * A constructor that takes as input a Tile which represents the position of the warrior in the game. The constructor uses the input to create a warrior with the given position, BASE HEALTH, BASE_COST, WEAPON_TYPE and BASE ATTACK DAMAGE. Write a class Axebringer derived from the Warrior class. The Axebringer class rep- resents warriors who wield axes. These warriors are less precise, but when their attacks land, they inflict high damage. They are heavy units that are difficult to move. This class has the following public static fields: * An double called BASE HEALTH indicating the base health of an Axebringer. * An int called BASE_COST indicating their base training cost. * An int called WEAPON_TYPE indicating their weapon type. This field should be given the value of 2, representing the axes. * An int called BASE ATTACK DAMAGE indicating their base attack damage. The Axebringer class also has the following public method: * A constructor that takes as input a Tile which represents the position of the warrior in the game. The constructor uses the input to create a warrior with the given position, BASE HEALTH, BASE_COST, WEAPON_TYPE and BASE ATTACK DAMAGE. STEP 1: The skeleton Let's start by creating a skeleton for some of the classes you will need. • Write a class called Tile. You can think of a tile as a square on the board on which the game will be played. We will come back to this class later. For the moment you can leave it empty while you work on creating classes that represents characters in the game. • Write an abstract class Fighter which has the following private fields: - A Tile field named position, representing the fighter's position in the game. A double field named health, representing the fighter's health points (HP). An int field named weaponType, representing the type of weapon the fighter is using. This value is used to rank different weapon types: higher values indicate higher weapon ranks. -An int field named attackDamage, representing the fighter's attack power. The class must also have the following public methods: 3 A constructor that takes as input a Tile indicating the position of the fighter, a double indicating its hp, an int indicating its weapon type, and an int indicating its attack damage. The constructor uses its inputs to initialize the corresponding fields. Please do not make a copy of the Tile object. A final getPosition() method to retrieve the position of this fighter. A final getHealth() method to retrieve the health points of this fighter. - A final getWeaponType() method to retrieve the weapon type of this fighter. - A final getAttackDamage () method to retrieve the attack damage of this fighter. A setPosition() method that takes a Tile as input and updates the value stored in the appropriate field. This method should not create a copy of the input Tile and should not return anything. All of the following must be subclasses of the Fighter class: Write a class Monster derived from the Fighter class. The Monster class has the following public method: * A constructor that takes as input a Tile indicating the position of the fighter, a double indicating its hp, an int indicating its weapon type, and an int indicating its attack damage. The constructor uses the inputs to create a Monster with the above characteristics. Write an abstract class Warrior derived from the Fighter class. The Warrior class has the following private field: * An int field named requiredSkillPoints, representing the number of skill points required to train this warrior. A player can only deploy a warrior if they have accumulated the necessary skill points. Different warriors require different amounts of skill points. The Warrior class also has the following public methods: A constructor that takes as input a Tile indicating the position of the fighter, a double indicating its hp, an int indicating its weapon type, an int indicating its attack damage and an int indicating its cost in skill points respectively. The constructor uses the inputs to create a Warrior with the above characteristics. * A getTraining Cost () method that returns the amount of skill points required to deploy this warrior. Write a class Bladesworn derived from the Warrior class. The Bladesworn class rep- resents warriors who wield swords as their weapons. These warriors are precise in their attacks and highly agile, making them effective at defending themselves and possibly advancing forward. This class has the following public static fields: * An double called BASE HEALTH indicating the base health of Bladesworns. * An int called BASE COST indicating their base training cost.
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 20RQ
Related questions
Question

Transcribed Image Text:* An int called WEAPON_TYPE indicating their weapon type. This field should be given
the value of 3, representing the swords.
* An int called BASE ATTACK DAMAGE indicating their base attack damage.
This class also has the following public method:
* A constructor that takes as input a Tile which represents the position of the warrior
in the game. The constructor uses the input to create a warrior with the given
position, BASE HEALTH, BASE_COST, WEAPON_TYPE and BASE ATTACK DAMAGE.
Write a class Axebringer derived from the Warrior class. The Axebringer class rep-
resents warriors who wield axes. These warriors are less precise, but when their attacks
land, they inflict high damage. They are heavy units that are difficult to move. This
class has the following public static fields:
* An double called BASE HEALTH indicating the base health of an Axebringer.
* An int called BASE_COST indicating their base training cost.
* An int called WEAPON_TYPE indicating their weapon type. This field should be given
the value of 2, representing the axes.
* An int called BASE ATTACK DAMAGE indicating their base attack damage.
The Axebringer class also has the following public method:
* A constructor that takes as input a Tile which represents the position of the warrior
in the game. The constructor uses the input to create a warrior with the given
position, BASE HEALTH, BASE_COST, WEAPON_TYPE and BASE ATTACK DAMAGE.

Transcribed Image Text:STEP 1: The skeleton
Let's start by creating a skeleton for some of the classes you will need.
• Write a class called Tile. You can think of a tile as a square on the board on which the game
will be played. We will come back to this class later. For the moment you can leave it empty
while you work on creating classes that represents characters in the game.
• Write an abstract class Fighter which has the following private fields:
- A Tile field named position, representing the fighter's position in the game.
A double field named health, representing the fighter's health points (HP).
An int field named weaponType, representing the type of weapon the fighter is using.
This value is used to rank different weapon types: higher values indicate higher weapon
ranks.
-An int field named attackDamage, representing the fighter's attack power.
The class must also have the following public methods:
3
A constructor that takes as input a Tile indicating the position of the fighter, a double
indicating its hp, an int indicating its weapon type, and an int indicating its attack
damage. The constructor uses its inputs to initialize the corresponding fields. Please do
not make a copy of the Tile object.
A final getPosition() method to retrieve the position of this fighter.
A final getHealth() method to retrieve the health points of this fighter.
- A final getWeaponType() method to retrieve the weapon type of this fighter.
- A final getAttackDamage () method to retrieve the attack damage of this fighter.
A setPosition() method that takes a Tile as input and updates the value stored in the
appropriate field. This method should not create a copy of the input Tile and should
not return anything.
All of the following must be subclasses of the Fighter class:
Write a class Monster derived from the Fighter class. The Monster class has the
following public method:
* A constructor that takes as input a Tile indicating the position of the fighter, a
double indicating its hp, an int indicating its weapon type, and an int indicating
its attack damage. The constructor uses the inputs to create a Monster with the
above characteristics.
Write an abstract class Warrior derived from the Fighter class. The Warrior class
has the following private field:
* An int field named requiredSkillPoints, representing the number of skill points
required to train this warrior. A player can only deploy a warrior if they have
accumulated the necessary skill points. Different warriors require different amounts
of skill points.
The Warrior class also has the following public methods:
A constructor that takes as input a Tile indicating the position of the fighter,
a double indicating its hp, an int indicating its weapon type, an int indicating
its attack damage and an int indicating its cost in skill points respectively. The
constructor uses the inputs to create a Warrior with the above characteristics.
* A getTraining Cost () method that returns the amount of skill points required to
deploy this warrior.
Write a class Bladesworn derived from the Warrior class. The Bladesworn class rep-
resents warriors who wield swords as their weapons. These warriors are precise in their
attacks and highly agile, making them effective at defending themselves and possibly
advancing forward. This class has the following public static fields:
* An double called BASE HEALTH indicating the base health of Bladesworns.
* An int called BASE COST indicating their base training cost.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
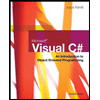
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
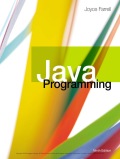
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
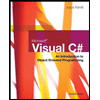
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
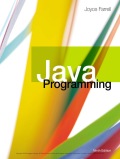
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
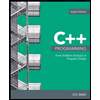
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
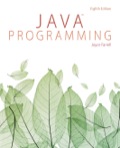
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
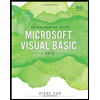
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning