PYTHON PROGRAMMING ONLY Im making a patient portal/ record keeping system but my code isnt working please help code below (class Menu section isnt working also menu =Menu() isnt working) CODE: class Person: def __init__(self, name, dob, address, phone_number): self.name = name self.dob = dob self.address = address self.phone_number = phone_number class Patient(Person): def __init__(self, name, dob, address, phone_number, medical_history): super().__init__(name, dob, address, phone_number) self.medical_history = medical_history class Menu: def __init__(self): self.options = { 1: self.view_personal_info, 2: self.update_personal_info, 3: self.view_medical_history, 4: self.update_medical_history, 5: self.exit } def display(self): print("Menu:\n") print("1. View personal information") print("2. Update personal information") print("3. View medical history") print("4. Update medical history") print("5. Exit") def run(self): while True: self.display() choice = input("Enter your choice: ") try: choice = int(choice) except ValueError: print("Invalid choice. Please enter a number.") continue if choice in self.options: self.options[choice]() else: print("Invalid choice. Please try again.") def view_personal_info(self): print(f"Name: {patient.name}") print(f"Date of Birth: {patient.dob}") print(f"Address: {patient.address}") print(f"Phone Number: {patient.phone_number}") print("\n") def update_personal_info(self): name = input("Enter your name: ") dob = input("Enter your date of birth: ") address = input("Enter your address: ") phone_number = input("Enter your phone number: ") patient.name = name patient.dob = dob patient.address = address patient.phone_number = phone_number print("Your personal information has been updated.") print("\n") def view_medical_history(self): print(f"Medical History: {patient.medical_history}") print("\n") def update_medical_history(self): medical_history = input("Enter your updated medical history: ") patient.medical_history = medical_history print("Your medical history has been updated.") print("\n") def exit(self): print("Goodbye!") exit() class Data: def __init__(self, file_name): self.file_name = file_name def load(self): with open(self.file_name, 'r') as f: data = f.read() return data patient = Patient("John Doe", "01/01/1990", "123 Main St.", "555-555-5555", "No significant medical history.") menu = Menu() menu.run()
PYTHON PROGRAMMING ONLY
Im making a patient portal/ record keeping system but my code isnt working please help code below
(class Menu section isnt working also menu =Menu() isnt working)
CODE:
class Person:
def __init__(self, name, dob, address, phone_number):
self.name = name
self.dob = dob
self.address = address
self.phone_number = phone_number
class Patient(Person):
def __init__(self, name, dob, address, phone_number, medical_history):
super().__init__(name, dob, address, phone_number)
self.medical_history = medical_history
class Menu:
def __init__(self):
self.options = {
1: self.view_personal_info,
2: self.update_personal_info,
3: self.view_medical_history,
4: self.update_medical_history,
5: self.exit
}
def display(self):
print("Menu:\n")
print("1. View personal information")
print("2. Update personal information")
print("3. View medical history")
print("4. Update medical history")
print("5. Exit")
def run(self):
while True:
self.display()
choice = input("Enter your choice: ")
try:
choice = int(choice)
except ValueError:
print("Invalid choice. Please enter a number.")
continue
if choice in self.options:
self.options[choice]()
else:
print("Invalid choice. Please try again.")
def view_personal_info(self):
print(f"Name: {patient.name}")
print(f"Date of Birth: {patient.dob}")
print(f"Address: {patient.address}")
print(f"Phone Number: {patient.phone_number}")
print("\n")
def update_personal_info(self):
name = input("Enter your name: ")
dob = input("Enter your date of birth: ")
address = input("Enter your address: ")
phone_number = input("Enter your phone number: ")
patient.name = name
patient.dob = dob
patient.address = address
patient.phone_number = phone_number
print("Your personal information has been updated.")
print("\n")
def view_medical_history(self):
print(f"Medical History: {patient.medical_history}")
print("\n")
def update_medical_history(self):
medical_history = input("Enter your updated medical history: ")
patient.medical_history = medical_history
print("Your medical history has been updated.")
print("\n")
def exit(self):
print("Goodbye!")
exit()
class Data:
def __init__(self, file_name):
self.file_name = file_name
def load(self):
with open(self.file_name, 'r') as f:
data = f.read()
return data
patient = Patient("John Doe", "01/01/1990", "123 Main St.", "555-555-5555", "No significant medical history.")
menu = Menu()
menu.run()

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

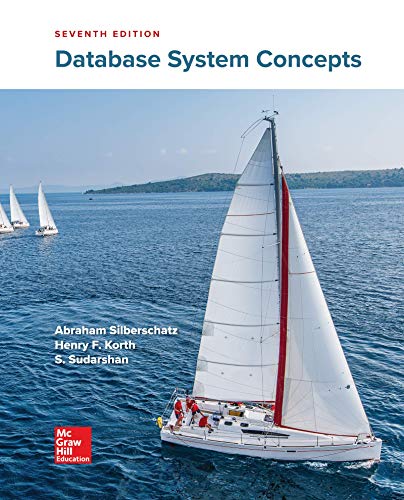
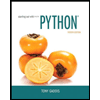
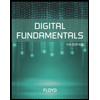
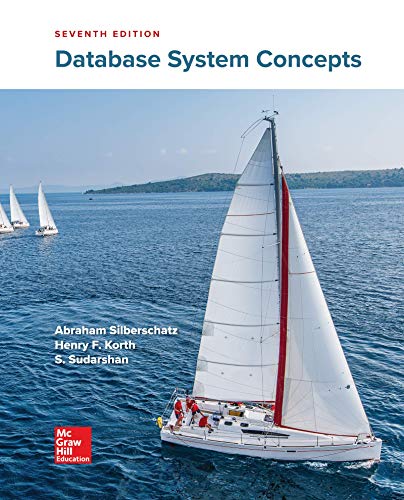
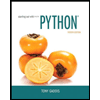
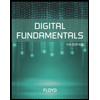
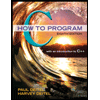
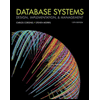
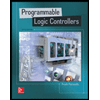