This assignment will continue with the user class you created in your last assignment. You will add a new_login function that will take three arguments, all as integers; month, day, and year. It will then verify that it is a valid date and set the last_login attribute to the correct string. Note: that just means no negative numbers, days are from 1 to 31 and months are from 1 to 12. We don't care if it's the 30th day of February. Cut and paste my hard-coded calls that will create several instances of User and call their functions. There is no user input on this assignment. Expected Output Example 1 Accessing user 1000: Jim Bob last logged in on June 12, 1998 Welcome to the jungle Jim Bob, you gonna die Welcome to the jungle Joe Bob, you gonna die Accessing user 1001: Joe Bob last logged in on July 4, 2001 Accessing user 1001: Joe Bob last logged in on February 3, 2025 That is not a valid month That is not a valid day. That is not a valid year Specifications • You should submit a single file called M7A2.py • It should follow the submission standards outlined here: Submission Standards • Your program will continue the User class you defined in M7A1.py Your program must end with the hard-coded code below (there is more this time)
This assignment will continue with the user class you created in your last assignment. You will add a new_login function that will take three arguments, all as integers; month, day, and year. It will then verify that it is a valid date and set the last_login attribute to the correct string. Note: that just means no negative numbers, days are from 1 to 31 and months are from 1 to 12. We don't care if it's the 30th day of February. Cut and paste my hard-coded calls that will create several instances of User and call their functions. There is no user input on this assignment. Expected Output Example 1 Accessing user 1000: Jim Bob last logged in on June 12, 1998 Welcome to the jungle Jim Bob, you gonna die Welcome to the jungle Joe Bob, you gonna die Accessing user 1001: Joe Bob last logged in on July 4, 2001 Accessing user 1001: Joe Bob last logged in on February 3, 2025 That is not a valid month That is not a valid day. That is not a valid year Specifications • You should submit a single file called M7A2.py • It should follow the submission standards outlined here: Submission Standards • Your program will continue the User class you defined in M7A1.py Your program must end with the hard-coded code below (there is more this time)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
class User:
def __init__(self, first_name, last_name, user_id, last_login, password):
self.first_name = first_name
self.last_name = last_name
self.user_id = user_id
self.last_login = last_login
self.password = password
def describe_user(self):
print(f"Accessing user {self.user_id}:")
print(f"{self.first_name} {self.last_name} last logged in on {self.last_login}")
def greet_user(self):
print(f"Welcome to the jungle {self.first_name} {self.last_name}, you gonna die")
def __init__(self, first_name, last_name, user_id, last_login, password):
self.first_name = first_name
self.last_name = last_name
self.user_id = user_id
self.last_login = last_login
self.password = password
def describe_user(self):
print(f"Accessing user {self.user_id}:")
print(f"{self.first_name} {self.last_name} last logged in on {self.last_login}")
def greet_user(self):
print(f"Welcome to the jungle {self.first_name} {self.last_name}, you gonna die")
Hard-code
# hard-coded calls
jim = User(first_name='Jim',
last_name='Bob',
user_id=1000,
last_login='June 12, 1998',
password='password123')
jim.describe_user()
jim.greet_user()
joe = User('Joe',
'Bob',
1001,
'July 4, 2001',
'babygirl')
joe.greet_user()
joe.describe_user()
joe.new_login(2, 3, 2025)
joe.describe_user()
joe.new_login(15, 3, 2025)
joe.new_login(2, 35, 2025)
joe.new_login(2, 3, -55)

Transcribed Image Text:·
Overview
This assignment will continue with the user class you created in your last assignment. You will add a new_login) function that will take three arguments, all as integers; month), day, and
year. It will then verify that it is a valid date and set the last_login attribute to the correct string. Note: that just means no negative numbers, days are from 1 to 31 and months are
from 1 to 12. We don't care if it's the 30th day of February.
Cut and paste my hard-coded calls that will create several instances of User and call their functions. There is no user input on this assignment.
Expected Output
Example 1
Accessing user 1000:
Jim Bob last logged in on June 12, 1998
Welcome to the jungle Jim Bob, you gonna die
Welcome to the jungle Joe Bob, you gonna die
Accessing user 1001:
Joe Bob last logged in on July 4, 2001
Accessing user 1001:
Joe Bob last logged in on February 3, 2025
That is not a valid month
That is not a valid day
That is not a valid year
Specifications
• You should submit a single file called M7A2.py
• It should follow the submission standards outlined here: Submission Standards
• Your program will continue the User class you defined in M7A1.py
.
• Your program must end with the hard-coded code below (there is more this time)

Transcribed Image Text:Tips and Tricks
Return statements will stop processing a function
Hard-Coded
Copy/paste the code below into your M7A1.py document, after your function definition.
# hard-coded calls
jim = User (first_name='Jim',
last_name='Bob',
user_id=1000,
last_login='June 12, 1998',
password='password123')
jim.describe_user()
jim.greet_user()
joe = User('Joe',
'Bob',
1001,
July 4, 2001',
'babygirl')
joe.greet_user()
joe.describe_user()
joe.new_login (2, 3, 2025)
joe.describe_user()
joe.new_login(15, 3, 2025)
joe.new_login (2, 35, 2025)
joe.new_login (2, 3, -55)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
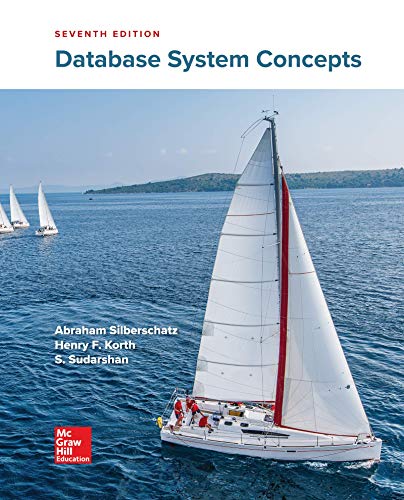
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
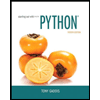
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
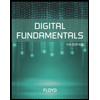
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
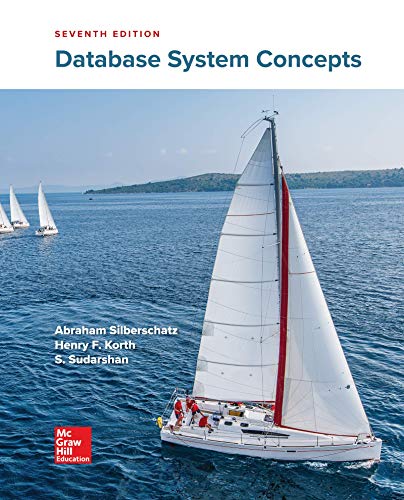
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
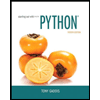
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
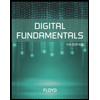
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
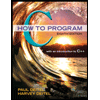
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
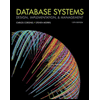
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
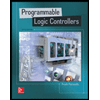
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education