Develop a Python script that processes object records. Read and write records from data file using pickle module. Specifications: Develop a tracking system for a Weight Loss Club. The system maintains and tracks membership Information for club members. The member-level information includes: 1. Member full name 2. Member ID 3. Gender 4. Weight 5. Phone (хxx-хх-хххх format)
class SavingsAccount(object):
RATE = 0.02
def __init__(self, name, pin, balance = 0.0):
self._name = name
self._pin = pin
self._balance = balance
def __str__(self):
result = 'Name: ' + self._name + '\n'
result += 'PIN: ' + self._pin + '\n'
result += 'Balance: ' + str(self._balance)
return result
def __eq__(self,a):
if self._name == a._name and self._pin == a._pin and self._balance == a._balance:
return True
else:
return False
def __gt__(self,a):
if self._balance > a._balance:
return True
else:
return False
def getBalance(self):
return self._balance
def getName(self):
return self._name
def getPin(self):
return self._pin
def deposit(self, amount):
self._balance += amount
return self._balance
def withdraw(self, amount):
Returns None if successful, or an
error message if unsuccessful."""
if amount < 0:
return 'Amount must be >= 0'
elif self._balance < amount:
return 'Insufficient funds'
else:
self._balance -= amount
return None
def computeInterest():
"""Computes, deposits, and returns the interest."""
interest = self._balance * SavingsAccount.RATE
self.deposit(interest)
return interest
account1= SavingsAccount('name1','1234',10000)
account2= SavingsAccount('name1','1234',10000)
account3= SavingsAccount('name2','1234',12000)
print(account1 == account2)
print(account1 == account3)
print(account1 > account2)
print(account3 > account2)
import pickle
class SavingsAccount(object):
RATE = 0.02
def __init__(self, name, pin, balance = 0.0):
self._name = name
self._pin = pin
self._balance = balance
def __str__(self):
result = 'Name: ' + self._name + '\n'
result += 'PIN: ' + self._pin + '\n'
result += 'Balance: ' + str(self._balance)
return result
def getBalance(self):
return self._balance
def getName(self):
return self._name
def getPin(self):
return self._pin
def deposit(self, amount):
"""Deposits the given amount."""
self._balance += amount
return self._balance
def withdraw(self, amount):
"""Withdraws the given amount.
Returns None if successful, or an
error message if unsuccessful."""
if amount < 0:
return 'Amount must be >= 0'
elif self._balance < amount:
return 'Insufficient funds'
else:
self._balance -= amount
return None
def computeInterest(self):
"""Computes, deposits, and returns the interest."""
interest = self._balance * SavingsAccount.RATE
self.deposit(interest)
return interest
class Bank(object):
"""This class represents a bank as a dictionary of
accounts. An optional file name is also associated
with the bank, to allow transfer of accounts to and
from permanent file storage."""
def __init__(self, fileName = None):
"""Creates a new dictionary to hold the accounts.
If a file name is provided, loads the accounts from
a file of pickled accounts."""
self._accounts = {}
self.fileName = fileName
if fileName != None:
fileObj = open(fileName, 'rb')
while True:
try:
account = pickle.load(fileObj)
self.add(account)
except EOFError:
fileObj.close()
break
def add(self, account):
"""Inserts an account using its PIN as a key."""
self._accounts[account.getPin()] = account
def remove(self, pin):
return self._accounts.pop(pin, None)
def get(self, pin):
return self._accounts.get(pin, None)
def computeInterest(self):
"""Computes interest for each account and
returns the total."""
total = 0
for account in self._accounts.values():
total += account.computeInterest()
return total
def __str__(self):
"""Return the string rep of the entire bank."""
return '\n'.join(map(str, self._accounts.values()))
def save(self, fileName = None):
"""Saves pickled accounts to a file. The parameter
allows the user to change file names."""
if fileName != None:
self.fileName = fileName
elif self.fileName == None:
return
fileObj = open(self.fileName, 'wb')
for account in self._accounts.values():
pickle.dump(account, fileObj)
fileObj.close()


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

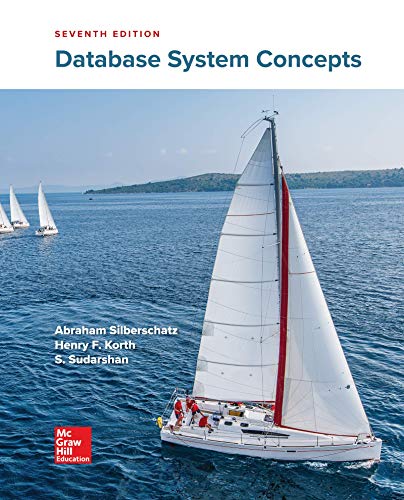
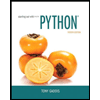
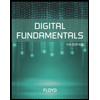
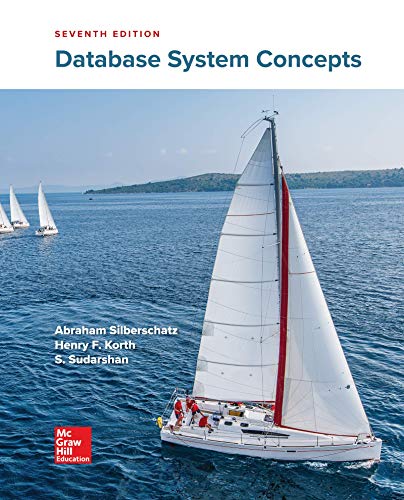
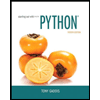
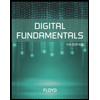
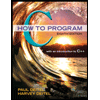
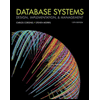
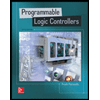